


Solutions to solve Java date calculation error exception (DateCalculationErrorExceotion)
Solution to solve Java date calculation error exception (DateCalculationErrorException)
Summary: When using Java for date calculation, you may sometimes encounter some error exceptions. This article will introduce some common date calculation error exceptions, and give solutions and code examples to help developers better deal with these problems.
1. Problem Description
In Java, date calculation is a common requirement. However, when doing date calculations, you sometimes encounter some error exceptions, as follows:
- NumberFormatException: When converting a string to a date object, a NumberFormatException exception may occur. This is usually because the date string is not in the expected format or contains non-numeric characters.
- ParseException: When using the SimpleDateFormat class to format or parse dates, a ParseException may occur. This may be due to the format of the date string not matching the specified format pattern, or the presence of an invalid date value.
- NullPointerException: When using date objects, if null value checking is not performed correctly, a NullPointerException may occur. This usually happens when calling a method on a date object.
2. Solutions and code examples
The following will introduce how to solve the above problems one by one and give corresponding code examples.
- Resolving NumberFormatException exception
To resolve NumberFormatException exception, you can use the try-catch statement to catch the exception and handle it. The following is a sample code:
try { String dateString = "20221231"; SimpleDateFormat dateFormat = new SimpleDateFormat("yyyyMMdd"); Date date = dateFormat.parse(dateString); // 日期计算逻辑 } catch (NumberFormatException e) { e.printStackTrace(); // 异常处理逻辑 }
In the above code, we use the parse method of the SimpleDateFormat class to convert the string into a date object. If the format of the string is incorrect, a NumberFormatException will be thrown. In the catch statement block, we can perform exception handling, such as outputting error information or performing other operations.
- Resolving ParseException exception
To resolve ParseException exception, you can use the try-catch statement to catch the exception and process it. The following is a sample code:
try { String dateString = "2022-12-31"; SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd"); Date date = dateFormat.parse(dateString); // 日期计算逻辑 } catch (ParseException e) { e.printStackTrace(); // 异常处理逻辑 }
In the above code, we also use the parse method of the SimpleDateFormat class to convert the string into a date object. If the format of the string does not match the specified format pattern, a ParseException will be thrown. In the catch statement block, we can perform exception handling, such as outputting error information or performing other operations.
- Resolving NullPointerException
To resolve NullPointerException, you can perform a null value check before using the date object. Here is a sample code:
Date date = null; if (date != null) { // 日期计算逻辑 } else { // 空值处理逻辑 }
In the above code, we avoid NullPointerException by checking whether the date object is null or not. If the date object is empty, we can perform corresponding null value processing logic, such as outputting an error message or performing other operations.
3. Summary
When using Java for date calculations, we may encounter some common errors and exceptions. This article describes some common problems and provides solutions and corresponding code examples. I hope this content can help developers better solve date calculation problems and write more robust code.
The above is the detailed content of Solutions to solve Java date calculation error exception (DateCalculationErrorExceotion). For more information, please follow other related articles on the PHP Chinese website!
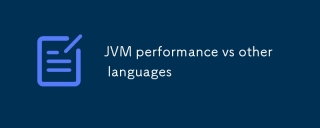
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
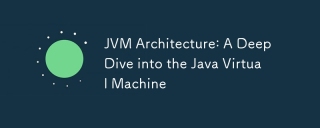
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
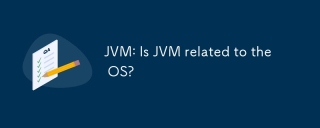
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
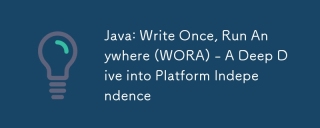
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
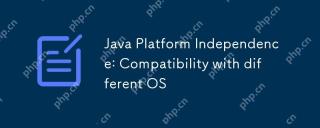
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
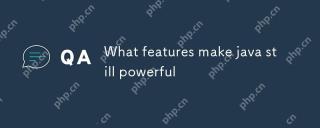
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
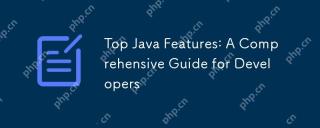
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
