How to handle complex form data structures in Java?
How to handle complex form data structures in Java?
As web applications become increasingly complex, the processing of form data is also becoming more and more complex. Processing complex form data structures in Java is a common requirement. This article will introduce several methods and techniques for processing complex form data and provide corresponding Java code examples.
1. Use HttpServletRequest to receive form data
In Java Web applications, we can use the HttpServletRequest object to receive form data. The HttpServletRequest object provides a series of methods to obtain parameter values in the form, including getParameter(), getParameterValues(), getParameterMap(), etc. The following is a sample code that demonstrates how to use the HttpServletRequest object to receive form data:
@WebServlet("/formHandler") public class FormHandlerServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 接收表单数据 String username = request.getParameter("username"); String password = request.getParameter("password"); String[] hobbies = request.getParameterValues("hobbies"); // 对接收到的数据进行处理 // TODO: 处理表单数据 // 返回处理结果 response.getWriter().write("表单数据处理成功!"); } }
The above code obtains the username and password parameter values in the form through the getParameter() method of the HttpServletRequest object. If there are multiple parameters with the same name in the form, you can use the getParameterValues() method to obtain all parameter values and store them in an array. After receiving the form data, we can process the data, such as storing it in the database or performing validation operations. Finally, we can return the processing results to the client through the getWriter() method of the HttpServletResponse object.
2. Use JavaBean to encapsulate form data
JavaBean is a common Java object, usually used to encapsulate form data. We can define a JavaBean class corresponding to the form data structure, and then directly assign the form data to the properties of the JavaBean object through the request parameters. The following is a sample code that uses JavaBean to encapsulate form data:
public class User { private String username; private String password; private String[] hobbies; // 省略getter和setter方法 }
In the above example, the User class defines the properties corresponding to the form data structure, including username, password, and hobbies. We can directly assign the request parameters to the properties of the User object through the HttpServletRequest object in the Servlet that processes form data. The following is a sample code:
@WebServlet("/formHandler") public class FormHandlerServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 创建User对象 User user = new User(); // 接收表单数据并赋值给User对象 user.setUsername(request.getParameter("username")); user.setPassword(request.getParameter("password")); user.setHobbies(request.getParameterValues("hobbies")); // 对接收到的数据进行处理 // TODO: 处理表单数据 // 返回处理结果 response.getWriter().write("表单数据处理成功!"); } }
The above code directly assigns the parameter values in the form to the properties of the User object through the HttpServletRequest object. This approach makes the code more concise and readable.
3. Use third-party libraries to process complex form data
In actual development, we may encounter processing more complex form data structures, such as nested objects or collections. At this point, some third-party libraries can be used to handle this complex form data. The following is an introduction to several commonly used third-party libraries:
- Apache Commons BeanUtils
Apache Commons BeanUtils provides some tool classes that can easily assign form data to properties of Java objects. The sample code is as follows:
@WebServlet("/formHandler") public class FormHandlerServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 创建User对象 User user = new User(); // 接收表单数据并赋值给User对象 try { BeanUtils.populate(user, request.getParameterMap()); } catch (IllegalAccessException | InvocationTargetException e) { e.printStackTrace(); } // 对接收到的数据进行处理 // TODO: 处理表单数据 // 返回处理结果 response.getWriter().write("表单数据处理成功!"); } }
- Jackson
Jackson is a Java library for processing JSON data and can convert JSON data into Java objects. If the form data is transmitted in JSON format, you can use the Jackson library to convert the JSON data into Java objects. The sample code is as follows:
@WebServlet("/formHandler") public class FormHandlerServlet extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 从请求中获取JSON数据 StringBuilder jsonBuilder = new StringBuilder(); String line; BufferedReader reader = request.getReader(); while ((line = reader.readLine()) != null) { jsonBuilder.append(line); } String jsonString = jsonBuilder.toString(); // 使用Jackson将JSON数据转换为Java对象 ObjectMapper objectMapper = new ObjectMapper(); User user = objectMapper.readValue(jsonString, User.class); // 对接收到的数据进行处理 // TODO: 处理表单数据 // 返回处理结果 response.getWriter().write("表单数据处理成功!"); } }
The above code obtains the JSON data in the request through the getReader() method of the HttpServletRequest object, and then uses the readValue() method of the ObjectMapper object to convert the JSON data into a Java object.
The above are several methods and techniques for dealing with complex form data structures. Based on actual needs, you can choose an appropriate method to process form data. No matter which method is used, we need to ensure the security and correctness of the data to avoid potential security holes or logic errors. During the code implementation process, appropriate parameter verification and exception handling can be performed according to specific circumstances to improve the stability and security of the program.
The above is the detailed content of How to handle complex form data structures in Java?. For more information, please follow other related articles on the PHP Chinese website!
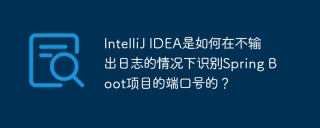
Start Spring using IntelliJIDEAUltimate version...
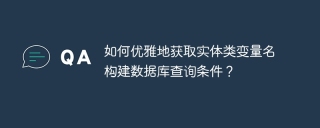
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
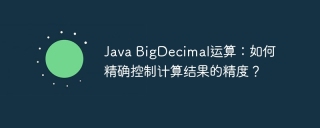
Java...
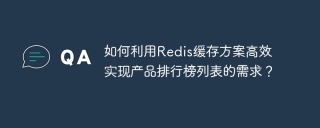
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
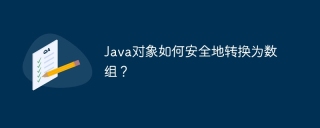
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
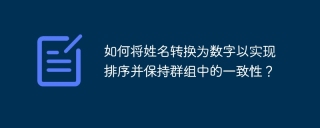
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
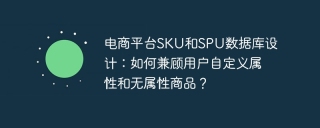
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
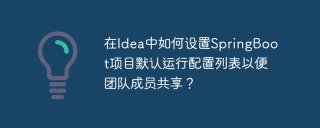
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
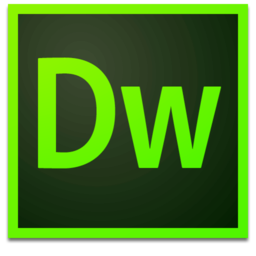
Dreamweaver Mac version
Visual web development tools
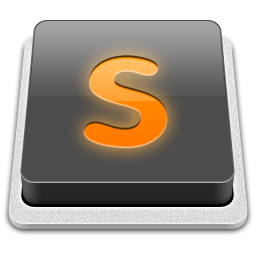
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
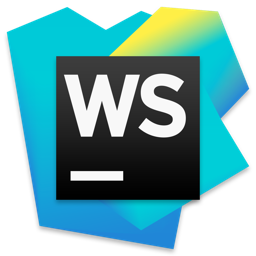
WebStorm Mac version
Useful JavaScript development tools