


Python realizes the docking of Baidu intelligent voice interface and easily builds intelligent audio applications
Python realizes the docking of Baidu intelligent voice interface and easily builds intelligent audio applications
Abstract:
Baidu intelligent voice interface provides developers with powerful audio processing function, which can be used to build various smart audio applications. This article will introduce how to use Python to connect Baidu intelligent voice interface, and provide code examples to help readers get started quickly.
1. Overview
Baidu Intelligent Voice Interface is an important component of Baidu Cloud artificial intelligence platform, providing speech recognition, speech synthesis and other functions. We can use Python to achieve docking by calling Baidu Cloud's API.
2. Preparation
To use Baidu Intelligent Voice Interface, you first need to register a developer account on Baidu Cloud Platform, create an application, and obtain the corresponding API Key and Secret Key. Then, you need to install Python related libraries, including requests, etc.
3. Text-to-speech
Use Baidu Intelligent Voice Interface to implement text-to-speech, which can convert text into voice files. The following is a simple example:
import requests def text_to_speech(text, filename): url = "https://tsn.baidu.com/text2audio" params = { "tex": text, "lan": "zh", "cuid": "your_cuid", "ctp": "1", "tok": "your_access_token", } response = requests.get(url, params=params) with open(filename, "wb") as f: f.write(response.content) text = "你好,欢迎使用百度智能语音接口" filename = "output.mp3" text_to_speech(text, filename)
In the code, we use the requests library to send HTTP requests, call Baidu's interface, and convert text to speech. It should be noted that the cuid and tok parameters need to be replaced with your own.
4. Speech Recognition
Use Baidu Intelligent Speech Interface to realize speech recognition, which can convert speech files into text content. The following is a simple example:
import requests def speech_to_text(filename): url = "https://vop.baidu.com/server_api" params = { "cuid": "your_cuid", "token": "your_access_token", "dev_pid": "1536", } headers = { "Content-Type": "audio/wav; rate=16000" } with open(filename, "rb") as f: audio_data = f.read() response = requests.post(url, params=params, headers=headers, data=audio_data) result = response.json() if result["err_no"] == 0: text = result["result"][0] return text else: return None filename = "input.wav" text = speech_to_text(filename) print(text)
In the code, we use the requests library to send HTTP requests, call Baidu's interface, and convert voice files into text. Similarly, the cuid and token parameters need to be replaced with your own.
5. Speech synthesis
Use Baidu intelligent voice interface to achieve speech synthesis, which can synthesize multiple speech fragments into one speech file. The following is a simple example:
import requests def synthesis(inputs, filename): url = "https://tsn.baidu.com/text2audio" params = { "tex": inputs, "lan": "zh", "cuid": "your_cuid", "ctp": "1", "tok": "your_access_token", } response = requests.get(url, params=params) with open(filename, "wb") as f: f.write(response.content) inputs = "你好,欢迎使用百度智能语音接口" filename = "output.mp3" synthesis(inputs, filename)
In the code, we use the requests library to send HTTP requests, call Baidu's interface, and synthesize multiple voice clips into a voice file. Similarly, the cuid and tok parameters need to be replaced with your own.
6. Summary
Through the introduction of this article, we have learned how to use Python to connect to Baidu intelligent voice interface, and have given several commonly used sample codes. Readers can expand and optimize according to their own needs to further build smart audio applications. At the same time, we also noticed some key parameters in the API, which need to be modified according to your actual situation.
It should be reminded that the use of Baidu intelligent voice interface needs to comply with Baidu's development specifications and privacy policy, and comply with relevant laws and regulations.
The above is the detailed content of Python realizes the docking of Baidu intelligent voice interface and easily builds intelligent audio applications. For more information, please follow other related articles on the PHP Chinese website!
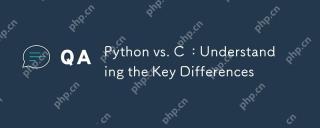
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
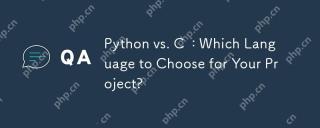
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
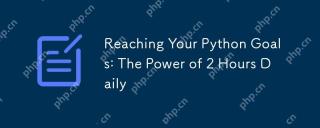
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
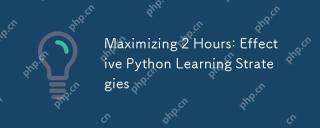
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
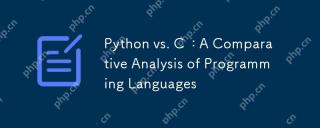
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
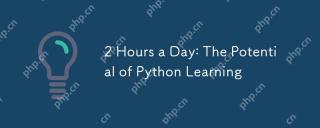
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
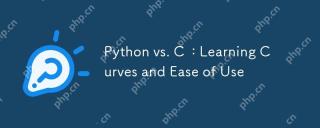
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
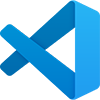
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor