


Interpretation of PHP writing specifications: an essential guide to standardizing the development process
Introduction:
In the software development process, writing specifications is very important, it can Improve code readability, maintainability, and overall quality. This article will explain the PHP writing specifications in detail and provide developers with an essential guide to standardize the development process.
1. Naming convention:
- Class names, interface names, and namespaces must use camel case naming with the first letter capitalized.
- Variable names, function names, and method names use all lowercase and underscore nomenclature.
- Use all uppercase and underscore nomenclature for constant names.
Example:
class UserController { public function getUserInfo() { // 方法实现 } } interface Logger { public function log($message); } namespace AppControllers; use AppModelsUserModel;
2. Indentation and line breaks:
- Use four spaces for indentation.
- Use Unix newline character (
), do not use Windows newline character (
). - Use a blank line to separate between functions and classes, between class methods, and between code logic blocks.
Example:
class UserController { public function getUserInfo() { // 方法实现 } public function updateUser($userId) { // 方法实现 } }
3. Comment specifications:
- Use single-line comments (//) or multi-line comments (/ /) for code comments.
- Comments should contain useful information, explaining the main functions, input and output of the code, etc.
- Classes and methods should have standardized DocBlock comments, including detailed descriptions, parameter descriptions and return value descriptions.
Example:
/** * 获取用户信息 * @param int $userId 用户ID * @return array 用户信息数组 */ public function getUserInfo($userId) { // 方法实现 } // 下面是一个单行注释的示例 $age = 18; // 设置用户年龄为18岁
4. Code formatting:
- The length of each line of code should not exceed 80 characters.
- Only write one statement per line, multiple statements are not allowed on the same line.
- Spaces should be added on both sides of unary operators and before and after binary operators.
Example:
$name = "Tom"; $age = 18; if ($age > 20 && $name === "Tom") { // 代码块 }
5. Error handling and exception capture:
- Try to avoid using global exception capture and should use it in specific code blocks try-catch to catch exceptions.
- Exception handling should be initiated as early as possible to avoid exception propagation.
Example:
try { // 可能抛出异常的代码块 } catch (Exception $e) { // 异常处理 }
6. Writing specifications for functions and methods:
- A function or method should only complete one function.
- The parameters of functions and methods must be properly verified and filtered.
- Use appropriate comments within functions or methods for explanation and clarification.
Example:
/** * 计算两个数的和 * @param int $num1 第一个数 * @param int $num2 第二个数 * @return int 两个数的和 */ function add($num1, $num2) { if (!is_numeric($num1) || !is_numeric($num2)) { throw new InvalidArgumentException('Invalid argument, numbers expected'); } return $num1 + $num2; }
Conclusion:
Good writing specifications can make the code easier to read and understand, improve code quality and maintainability. When developing with PHP, following the above writing specifications will give you a better development experience. I hope this article can provide PHP developers with an essential guide to standardizing the development process.
The above is the detailed content of Interpretation of PHP writing specifications: an essential guide to standardizing the development process. For more information, please follow other related articles on the PHP Chinese website!
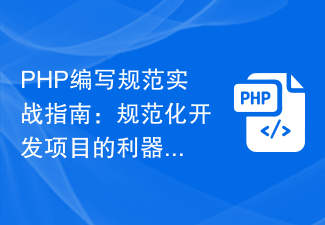
PHP编写规范实战指南:规范化开发项目的利器引言:在一个团队协作的开发环境中,编写规范是非常重要的。它不仅可以提高代码的可读性和可维护性,还可以减少错误和冲突的发生。本文将介绍一些PHP编写规范的实战指南,并通过代码示例来展示具体的规范。一、命名规范:类名、方法名和属性名使用驼峰命名法,并且首字母小写。常量名全部大写,多个单词用下划线分割。变量名使用有意义且
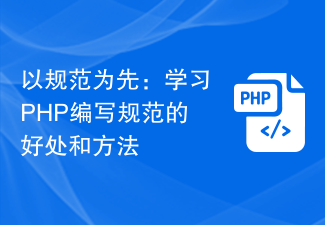
以规范为先:学习PHP编写规范的好处和方法一、引言编程规范,作为程序员必备的基本素养之一,在保证代码质量、可读性、可维护性等方面具有重要作用。对于PHP程序员而言,学习和遵守PHP编写规范是提升自身能力,提高团队协作效率的重要一步。本文将讨论学习PHP编写规范的好处,并提供方法与实例。二、学习PHP编写规范的好处提高代码质量:规范化的编码习惯能够减少错误和漏
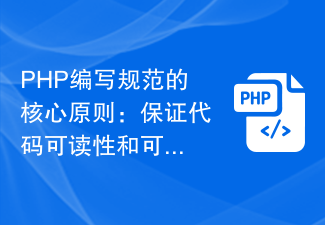
PHP编写规范的核心原则:保证代码可读性和可维护性摘要:在PHP开发中,编写规范的代码是非常重要的。良好的编码风格可以提高代码的可读性和可维护性,减少错误的发生。本文将介绍一些PHP编写规范的核心原则,并给出相应的代码示例。代码缩进与行宽:代码缩进可以增加代码的可读性,建议使用4个空格作为缩进的标准。行宽一般建议不超过80个字符,超过时可以进行换行处理。示例
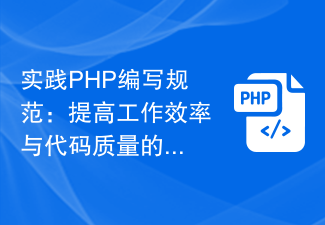
实践PHP编写规范:提高工作效率与代码质量的行动指南在当前的软件开发领域中,PHP是最受欢迎和广泛应用的语言之一。然而,由于开发人员的水平参差不齐,导致很多项目的代码质量参差不齐,可维护性低下。为了提高工作效率和代码质量,本文将介绍一些实践PHP编写规范的行动指南。选择合适的编码风格选择合适的编码风格是编写规范化代码的首要任务。常见的编码风格有PSR-1和P
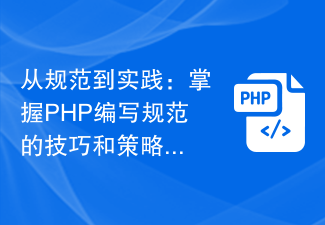
从规范到实践:掌握PHP编写规范的技巧和策略引言PHP是一种功能强大且广泛应用的编程语言,用于创建动态网页和应用程序。然而,许多开发人员在编写PHP代码时往往缺乏规范和一致性。良好的编码规范是保证代码质量和可维护性的重要因素。本文将介绍一些掌握PHP编写规范的技巧和策略,并提供一些代码示例。一、命名规范变量和函数名变量和函数名应该以小写字母开头,并使用驼峰命
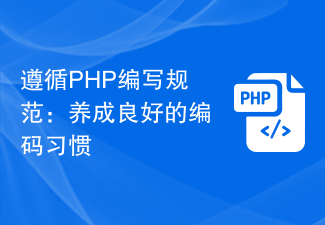
遵循PHP编写规范:养成良好的编码习惯在PHP开发中,编写规范是非常重要的,它能够提高代码的可读性和可维护性,降低代码出错的概率。遵循PHP编写规范,能够让我们的代码更加规范、统一,便于团队协作和后期维护。以下是一些常见的PHP编写规范和养成良好的编码习惯。缩进和空格在PHP中,缩进和空格的使用对于代码的可读性和美观性起着重要作用。一般来说,推荐使用四个空格
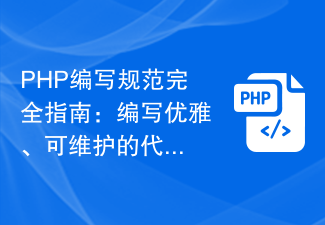
PHP编写规范完全指南:编写优雅、可维护的代码之道导言:在现代软件开发过程中,使用一致的编码风格和规范是非常重要的。它不仅能够提高代码的可读性和可维护性,而且有助于整个团队的协作。本文将介绍一些PHP编写规范的基本原则和最佳实践,并提供一些实例代码作为参考。一、命名规范类名应使用大驼峰式命名法,例如:MyClass。方法和函数名应使用小驼峰式命名法,例如:m
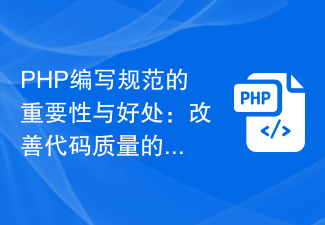
PHP编写规范的重要性与好处:改善代码质量的利器引言编写规范是开发团队中的一个重要事项。无论是个人开发还是团队合作,统一的编写规范能够提高代码的可读性、可维护性和可扩展性。在PHP开发中,编写规范更是至关重要的,本文将阐述PHP编写规范的重要性与好处,并通过示例代码来说明如何遵循规范。一、提高代码可读性命名规范良好的命名规范能够让代码的含义更加清晰


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
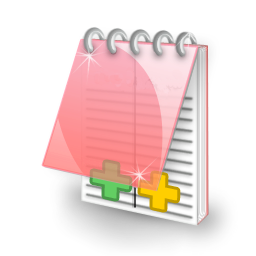
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
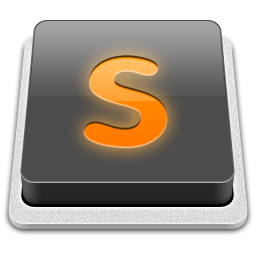
SublimeText3 Mac version
God-level code editing software (SublimeText3)
