Implementing high-concurrency online ordering system based on Swoole
A high-concurrency online ordering system based on Swoole
Introduction:
With the popularization of the Internet and the improvement of people's living standards, takeout ordering has become a modern One of the common services in life. The online ordering system needs to cope with a large number of user requests while ensuring high performance and high concurrency of the system. In this article, we will introduce how to implement a highly concurrent online ordering system based on Swoole, a powerful PHP extension.
Swoole is a coroutine and asynchronous programming extension for PHP that can be used to build high-performance network communication applications. It can be used with web servers such as Nginx or Apache to improve the concurrent processing capabilities of applications through asynchronous IO and event-driven methods.
Step 1: Environment setup
First, we need to install the Swoole extension. Can be installed via PECL or source code. Once installed, the Swoole extension can be enabled in the php.ini file.
Step 2: Create a server
The next step is to create a Swoole server instance to handle user requests. Here is a simple example:
<?php $server = new SwooleHttpServer("0.0.0.0", 80); $server->on("request", function ($request, $response) { $response->header("Content-Type", "text/html; charset=utf-8"); $response->end("Hello, World!"); }); $server->start();
In this example, we create an HTTP server instance and bind it to port 80 at IP address 0.0.0.0. When a request arrives, the server calls a callback function to process the request and return a response.
Step 3: Write business logic
Next, we need to write specific business logic to implement the functions of the online ordering system. This includes processing user requests, querying restaurant ordering information, processing orders and other operations.
<?php $server = new SwooleHttpServer("0.0.0.0", 80); $server->on("request", function ($request, $response) { // 获取用户请求的路径 $path = $request->server['request_uri']; // 根据路径不同,执行不同的业务逻辑 switch ($path) { case "/restaurant": // 处理餐厅信息查询逻辑 $response->header("Content-Type", "application/json; charset=utf-8"); $response->end(json_encode(["name" => "餐厅A", "address" => "xxx"])); break; case "/order": // 处理用户下单逻辑 $response->header("Content-Type", "text/html; charset=utf-8"); $response->end("下单成功"); break; default: $response->header("Content-Type", "text/html; charset=utf-8"); $response->end("页面不存在"); break; } }); $server->start();
In this example, we execute different business logic according to the user's request path. For example, when the user access path is "/restaurant", the restaurant information is returned; when the user access path is "/order", the user places an order; otherwise, a prompt that the page does not exist is returned.
Step 4: Testing and Optimization
After completing the code writing, we need to test and perform performance optimization. You can use the ab command or other professional performance testing tools to simulate concurrent requests and test and analyze performance indicators.
Based on the test results, we can carry out targeted optimization, such as using caching technology, adjusting server options, using connection pools and other means to improve the performance and concurrency of the system.
Conclusion:
This article introduces how to use Swoole to implement a highly concurrent online ordering system. By using the coroutine and asynchronous IO features provided by Swoole, the performance and concurrency of the system can be greatly improved. At the same time, we also introduce simple sample code to help readers better understand and apply Swoole. I hope readers can successfully build a high-performance online ordering system through the guidance of this article.
The above is the detailed content of Implementing high-concurrency online ordering system based on Swoole. For more information, please follow other related articles on the PHP Chinese website!
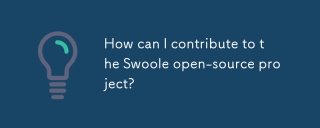
The article outlines ways to contribute to the Swoole project, including reporting bugs, submitting features, coding, and improving documentation. It discusses required skills and steps for beginners to start contributing, and how to find pressing is
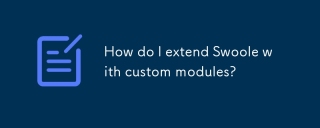
Article discusses extending Swoole with custom modules, detailing steps, best practices, and troubleshooting. Main focus is enhancing functionality and integration.
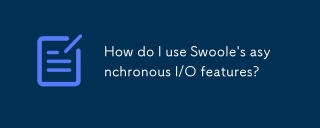
The article discusses using Swoole's asynchronous I/O features in PHP for high-performance applications. It covers installation, server setup, and optimization strategies.Word count: 159
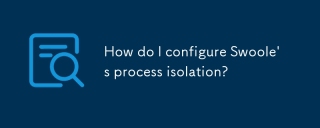
Article discusses configuring Swoole's process isolation, its benefits like improved stability and security, and troubleshooting methods.Character count: 159
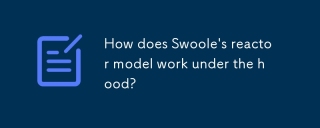
Swoole's reactor model uses an event-driven, non-blocking I/O architecture to efficiently manage high-concurrency scenarios, optimizing performance through various techniques.(159 characters)
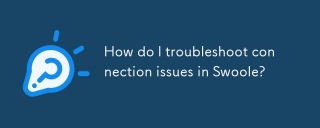
Article discusses troubleshooting, causes, monitoring, and prevention of connection issues in Swoole, a PHP framework.
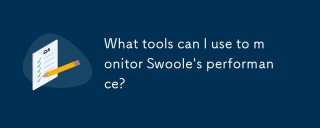
The article discusses tools and best practices for monitoring and optimizing Swoole's performance, and troubleshooting methods for performance issues.
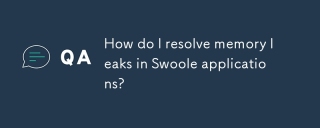
Abstract: The article discusses resolving memory leaks in Swoole applications through identification, isolation, and fixing, emphasizing common causes like improper resource management and unmanaged coroutines. Tools like Swoole Tracker and Valgrind


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
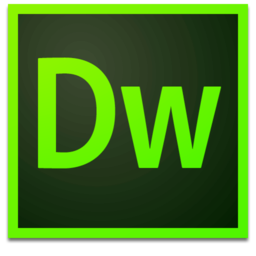
Dreamweaver Mac version
Visual web development tools
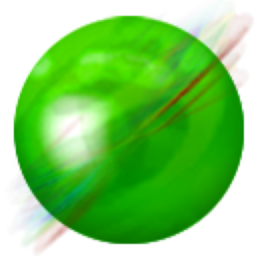
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment