How do I extend Swoole with custom modules?
Extending Swoole with custom modules involves several steps that allow you to enhance its functionality to meet specific needs. Here's a detailed guide on how to do this:
- Understand Swoole's Architecture: Before you start, familiarize yourself with Swoole's core architecture, especially the coroutine and event-driven models. This understanding will help you decide where your custom modules fit best within Swoole's framework.
-
Create Your Custom Module: Begin by creating a PHP class that encapsulates the functionality you want to add. This class should follow PHP's object-oriented programming principles. For example, if you want to add a custom logging feature, your class might look like this:
class CustomLogger { public function log($message) { // Custom logging logic here } }
-
Integrate with Swoole: Swoole allows you to load PHP classes at runtime. To integrate your custom module, you can create a coroutine or a worker process within your Swoole server to instantiate and use your custom class:
$server = new Swoole\Http\Server("0.0.0.0", 9501); $server->on('WorkerStart', function ($server, $workerId) { // Load your custom module require_once __DIR__ . '/path/to/CustomLogger.php'; $logger = new CustomLogger(); $server->on('Request', function ($request, $response) use ($logger) { $logger->log("New request received"); $response->end("Hello, World!"); }); }); $server->start();
- Testing and Deployment: After writing your custom module, thoroughly test it within your Swoole application. Ensure that the module does not conflict with existing Swoole features or degrade performance. Once tested, deploy your application with the new module.
What are the best practices for integrating custom modules into Swoole?
Integrating custom modules into Swoole effectively requires following these best practices:
- Modular Design: Keep your modules as independent and modular as possible. This design philosophy will make your modules easier to maintain and upgrade without affecting other parts of your application.
- Efficient Resource Management: Swoole is designed for high performance and low latency. Ensure that your custom modules are resource-efficient, particularly in terms of memory and CPU usage. Use coroutines to manage blocking operations to avoid impacting performance.
- Error Handling and Logging: Implement robust error handling within your modules. Also, ensure that your modules log events and errors appropriately, which can be crucial for debugging and maintenance.
- Compatibility and Version Control: Always consider Swoole’s version when developing custom modules. Keep track of the Swoole versions you are compatible with and ensure smooth upgrades by testing against new versions.
- Documentation: Thoroughly document your custom modules, including their functionality, how they interact with Swoole, and any dependencies or prerequisites for their use.
Can you recommend tools or libraries that facilitate the creation of custom modules for Swoole?
Several tools and libraries can aid in the creation of custom modules for Swoole:
- Swoole IDE Helper: This tool provides auto-completion and type hints for Swoole classes in your IDE, which can significantly speed up development and reduce errors when creating custom modules.
- PHP-FFI: The Foreign Function Interface (FFI) allows you to call C functions and use C data types directly from PHP code. This can be useful if you need to optimize performance-critical parts of your custom modules.
- Swoole Tracker: This tool helps track the performance of Swoole applications, which is crucial when optimizing your custom modules to ensure they do not negatively impact your application's performance.
- PHPUnit: A widely used testing framework for PHP. Writing unit tests for your custom modules with PHPUnit ensures they work as expected and helps catch regressions during future updates.
How do I troubleshoot common issues when extending Swoole with custom modules?
Troubleshooting issues with custom Swoole modules involves several strategies:
- Check Swoole Logs: Start by reviewing the logs generated by Swoole. They often contain valuable information about errors and warnings related to your custom modules.
- Use Debugging Tools: Tools like Xdebug can be invaluable for stepping through your code and identifying where things go wrong. Ensure your debugging tool is compatible with Swoole's asynchronous nature.
- Performance Profiling: If your module is causing performance issues, use profiling tools like Blackfire or Swoole Tracker to identify bottlenecks and optimize accordingly.
- Check for Compatibility Issues: Ensure your custom modules are compatible with the current version of Swoole. Sometimes, version incompatibilities can lead to unexpected behaviors.
- Community and Forums: If you encounter an issue that you can’t resolve, consider reaching out to the Swoole community or forums like Stack Overflow. Often, others might have encountered and solved similar problems.
By following these steps and best practices, you can effectively extend Swoole with custom modules and troubleshoot any issues that arise during the process.
The above is the detailed content of How do I extend Swoole with custom modules?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
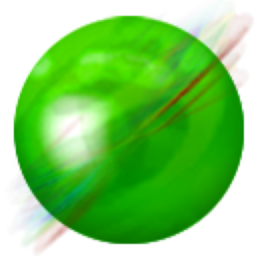
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
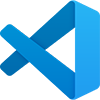
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
