


Exploration of Queue Optimization Techniques in PHP High Concurrency Processing
Exploration of Queue Optimization Techniques in PHP High Concurrency Processing
Introduction:
In today's Internet era, the processing of high concurrent requests has become a very important issue . As a scripting language mainly used for web development, PHP has some bottlenecks in concurrency processing. This article will explore PHP's queue optimization techniques in high-concurrency processing to help developers better handle requests in high-concurrency environments.
1. Why is queue optimization needed?
In a high-concurrency environment, you often encounter an influx of requests. If these requests are processed directly at the same time, it will inevitably cause excessive pressure on the server, resulting in slow system response or even crash. Therefore, we need a mechanism to schedule and process requests, and queue optimization plays an important role at this time.
2. Use queues for concurrent request processing
- Use message queues
Message queues are a way to asynchronously transmit messages, mainly including producers, consumers and Queue three parts. In PHP, you can use message queues such as RabbitMQ and Beanstalkd for high-concurrency processing. The following is a sample code using RabbitMQ:
// Producer
$connection = new AMQPConnection([
'host' => 'localhost', 'port' => '5672', 'login' => 'guest', 'password' => 'guest'
]);
$channel = $connection ->channel();
$channel->queue_declare('queue_name', false, false, false, false);
$message = new AMQPMessage(json_encode(['data' => 'hello ']));
$channel->basic_publish($message, '', 'queue_name');
$channel->close();
$connection->close();
// Consumer
$connection = new AMQPConnection([
'host' => 'localhost', 'port' => '5672', 'login' => 'guest', 'password' => 'guest'
]);
$channel = $connection->channel();
$channel ->queue_declare('queue_name', false, false, false, false);
$callback = function ($message) {
$data = json_decode($message->body, true); // 进行具体处理逻辑 // ... $message->ack();
};
$channel->basic_consume(' queue_name', '', false, false, false, false, $callback);
while (count($channel->callbacks)) {
$channel->wait();
}
$channel-> close();
$connection->close();
- Use process pool
Multiple processes in PHP can effectively utilize the resources of multi-core CPUs. By creating a process pool, and each process takes tasks from the queue for processing, the efficiency of concurrent processing can be maximized. The following is a sample code using swoole extension:
$pool = new SwooleProcessPool(10); // Create a process pool of 10 processes
$pool->on('WorkerStart' , function ($pool, $workerId) {
swoole_timer_tick(1000, function ($timerId) { $message = getMessageFromQueue(); // 从队列中取出任务 if ($message) { // 进行具体处理逻辑 // ... } if (队列为空) { swoole_timer_clear($timerId); // 停止任务处理 } });
});
$pool->start();
3. Some precautions for queue optimization
- Message reliability
In order to ensure the reliability of the message, we can confirm the message on the consumer side, such as $message->ack() in the sample code. If an exception occurs when the consumer processes the message or the processing time is too long, the message confirmation mechanism can be used to ensure that the message will not be lost. - Avoid queue blocking
In actual applications, queue blocking may be encountered. To avoid this situation, multiple consumers can be used to jointly consume messages in the queue to improve processing efficiency. - Monitoring and Alarming
In a high-concurrency environment, we need to monitor the queue, discover problems in time and repair them. You can use tools (such as Prometheus, Grafana, etc.) to monitor and set up alarm mechanisms.
Conclusion:
Queue optimization is an essential part of high-concurrency processing. By using message queues and process pools, concurrent processing efficiency can be effectively improved and the smooth operation of the system can be ensured. At the same time, in practical applications, we need to pay attention to the reliability of messages and queue blocking issues.
(Note: The above example code is for reference only, and the specific implementation can be adjusted and expanded according to actual business needs.)
Total word count: 980 words
The above is the detailed content of Exploration of Queue Optimization Techniques in PHP High Concurrency Processing. For more information, please follow other related articles on the PHP Chinese website!
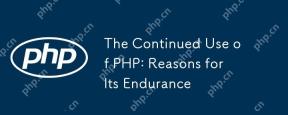
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
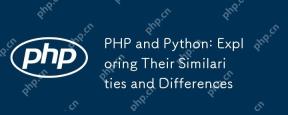
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
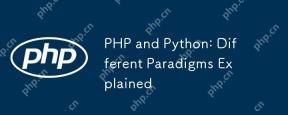
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
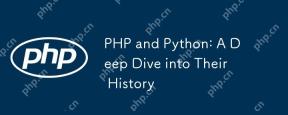
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
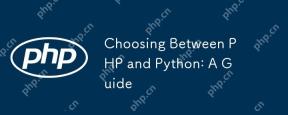
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
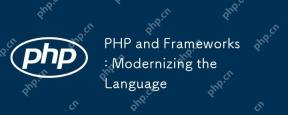
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
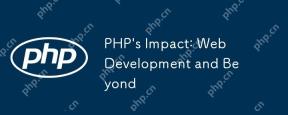
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
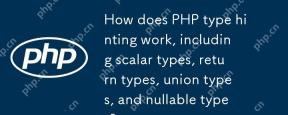
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.