


Study the many-to-many relationship in PHP object-oriented programming
In PHP object-oriented programming, the many-to-many relationship refers to two There is a many-to-many relationship between entities. This relationship often appears in practical applications, such as the relationship between students and courses. A student can choose multiple courses, and a course can also be selected by multiple students. A common way to implement this relationship is to establish the join through an intermediate table.
Below we will use code examples to demonstrate how to implement many-to-many relationships in PHP.
First, we need to create three classes: Student class, Course class and Enrollment class as intermediate tables.
class Student { private $name; private $courses; public function __construct($name) { $this->name = $name; $this->courses = array(); } public function enrollCourse($course) { $this->courses[] = $course; $course->enrollStudent($this); } public function getCourses() { return $this->courses; } } class Course { private $name; private $students; public function __construct($name) { $this->name = $name; $this->students = array(); } public function enrollStudent($student) { $this->students[] = $student; } public function getStudents() { return $this->students; } } class Enrollment { private $student; private $course; public function __construct($student, $course) { $this->student = $student; $this->course = $course; } }
In the above code, the relationship between the student class (Student) and the course class (Course) is many-to-many. The enrollCourse() method in the student class is used to associate students with courses, and the enrollStudent() method in the course class also associates students with courses. In this way, we can get other entities associated with any entity.
Now let’s test these classes.
// 创建学生对象 $student1 = new Student("Alice"); $student2 = new Student("Bob"); // 创建课程对象 $course1 = new Course("Math"); $course2 = new Course("English"); // 学生选课 $student1->enrollCourse($course1); $student1->enrollCourse($course2); $student2->enrollCourse($course1); // 输出学生的课程 echo $student1->getCourses()[0]->getName(); // 输出 "Math" echo $student1->getCourses()[1]->getName(); // 输出 "English" echo $student2->getCourses()[0]->getName(); // 输出 "Math" // 输出课程的学生 echo $course1->getStudents()[0]->getName(); // 输出 "Alice" echo $course1->getStudents()[1]->getName(); // 输出 "Bob" echo $course2->getStudents()[0]->getName(); // 输出 "Alice"
Through the above code, we created two student objects and two course objects, and associated students and courses through the enrollCourse() method. By calling the getCourses() method and getStudents() method, we can get the student's courses and the students of the course, thereby realizing a many-to-many relationship query.
The above is an example of implementing a many-to-many relationship in PHP object-oriented programming. By using intermediate tables to establish associations between entities, we can easily handle many-to-many relationships and query and operate related data. This design pattern is very common in actual development and is very helpful for establishing complex relationships. Hope the above content can be helpful to you!
The above is the detailed content of Studying Many-to-Many Relationships in PHP Object-Oriented Programming. For more information, please follow other related articles on the PHP Chinese website!
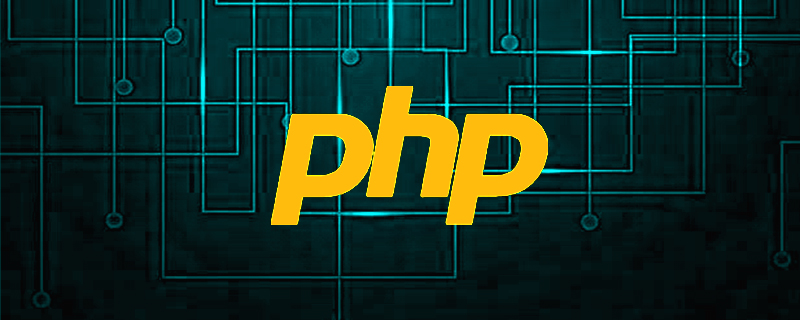
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
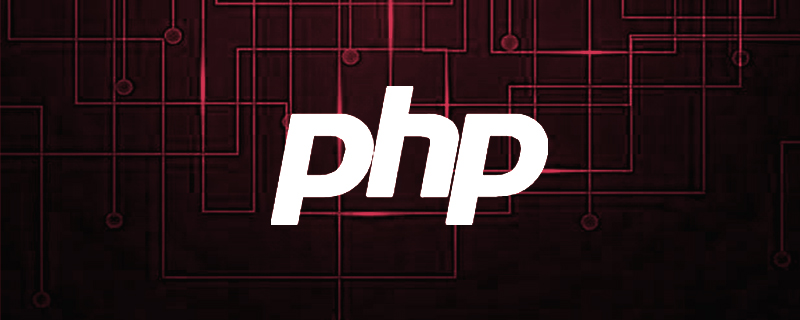
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
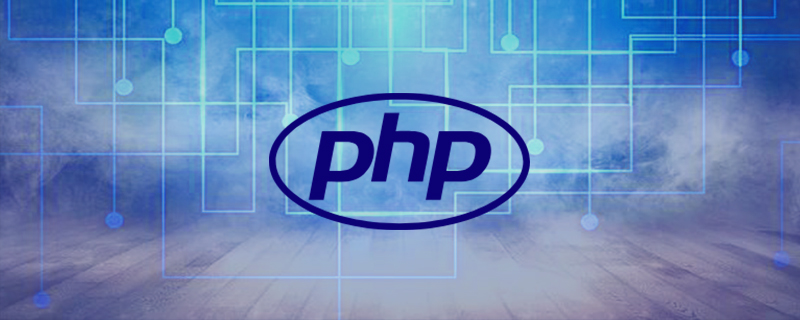
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
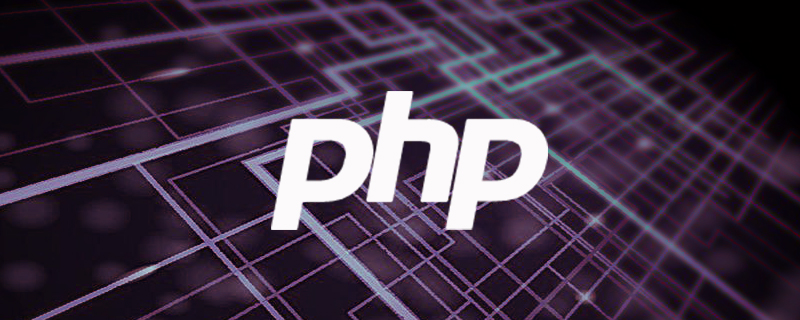
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
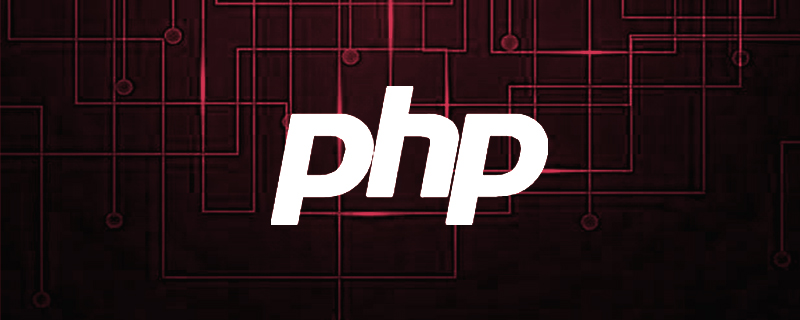
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
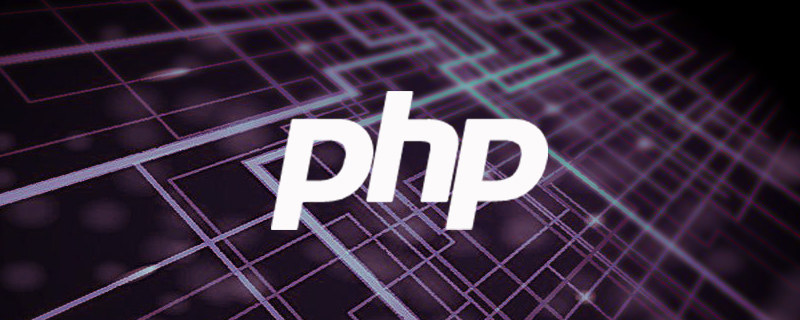
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
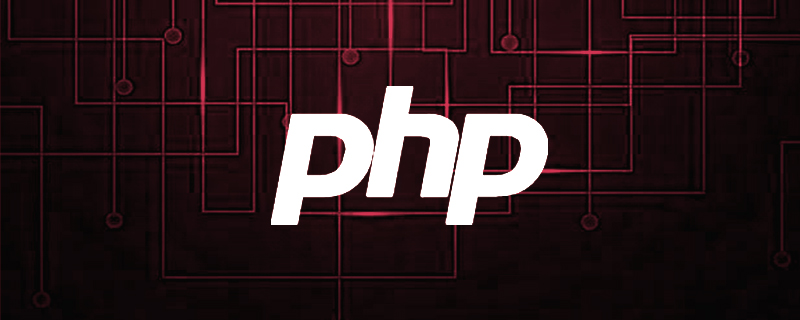
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
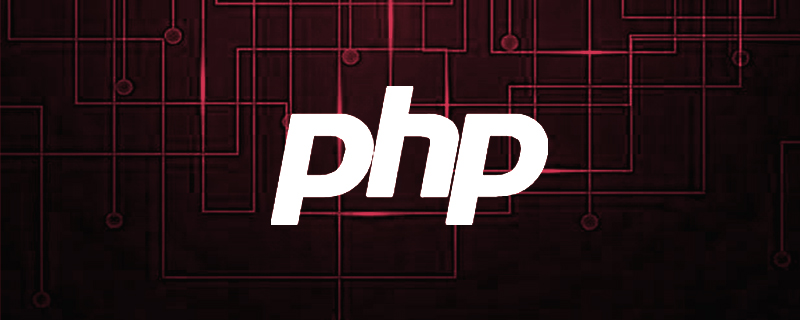
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
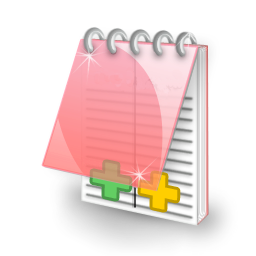
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
