


How to use Vue form processing to implement data preprocessing before form submission
Overview:
In web development, forms are one of the most common elements. . Before submitting the form, we often need to perform some preprocessing on the data entered by the user, such as format verification, data conversion, etc. The Vue framework provides convenient and easy-to-use form processing functions. This article will introduce how to use Vue form processing to implement data preprocessing before form submission.
1. Create a Vue instance and form control
First, we need to create a Vue instance and define a component containing the form control. Here is a simple example:
<template> <div> <form @submit.prevent="submitForm"> <label for="username">用户名:</label> <input type="text" id="username" v-model="username"> <label for="password">密码:</label> <input type="password" id="password" v-model="password"> <button type="submit">提交</button> </form> </div> </template> <script> export default { data() { return { username: '', password: '' } }, methods: { submitForm() { // 表单数据提交处理 } } } </script>
The above code defines a form containing username and password input boxes, and a submit button. Two responsive attributes username
and password
are defined in data
, which are used to bind the value of the input box. A submitForm
method is defined in methods
for handling form submission events.
2. Data preprocessing
Before submitting the form, we can preprocess the input data in the submitForm
method. Here is an example of preprocessing usernames and passwords:
methods: { submitForm() { // 数据预处理 let processedUsername = this.username.trim(); let processedPassword = this.password.trim(); // 进行校验 if (processedUsername === '') { alert('请输入用户名'); return; } if (processedPassword === '') { alert('请输入密码'); return; } // 正常提交表单 // ... } }
In the above code, we first use the trim()
method to remove possible spaces in the input box. Then it determines whether the user has entered a valid username and password through a simple non-empty check. If the verification passes, we can continue with subsequent operations, such as submitting a form asynchronously or sending a request.
3. Submit the form
After the data preprocessing passes, we can perform the form submission operation. There are two common ways here:
-
Submit the form asynchronously:
methods: { submitForm() { // 数据预处理 // ... // 异步提交表单 axios.post('/api/submit', { username: this.username, password: this.password }) .then(res => { // 处理提交成功后的逻辑 }) .catch(error => { // 处理提交失败后的逻辑 }); } }
The above code uses the Axios library to send a POST request, Send the username and password to the backend as the request body. Through the .then()
and .catch()
methods, we can handle the logic after successful submission and failed submission.
-
Send request:
methods: { submitForm() { // 数据预处理 // ... // 使用form的submit()方法提交表单 document.getElementById('myForm').submit(); } }
The above code uses the document.getElementById().submit()
method to submit Form. It should be noted that this method does not go through Vue's data binding and preprocessing links, and is generally used when files need to be transferred.
Summary:
This article introduces how to use Vue form processing to achieve data preprocessing before form submission. By defining the Vue component, binding the input box value and writing the logic to submit the form, we can easily preprocess, verify and submit the data entered by the user. This improves the user experience of the form and increases data security and consistency. I hope this article can help readers better use Vue to process form data.
The above is the detailed content of How to use Vue form processing to implement data preprocessing before form submission. For more information, please follow other related articles on the PHP Chinese website!
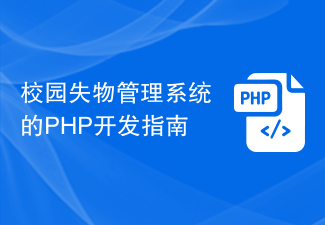
校园失物管理系统的PHP开发指南随着大学校园越来越大、人流量增加,学生们在学校里遗失物品的情况也变得越来越常见。为了更好地管理校园内的失物招领情况,开发一个校园失物管理系统就显得非常必要。本文将简要介绍如何使用PHP语言开发一个简单而实用的校园失物管理系统,其中包括具体的代码示例。首先,我们需要确定系统的功能需求。一个简单的校园失物管理系统主要应包括以下功能
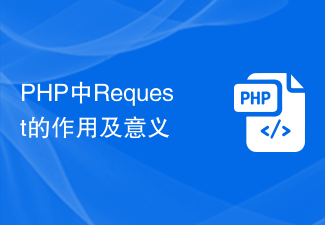
PHP中Request的作用及意义在PHP编程中,Request是指向Web服务器发送请求的一种机制,它在Web开发中起着至关重要的作用。Request主要用于获取客户端发送过来的数据,比如表单提交、GET或POST请求等,通过Request能够获取到用户输入的数据,并对这些数据进行处理和响应。本文将介绍PHP中Request的作用及意义,并给出具体的代码示
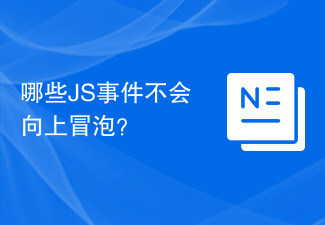
JS事件中有哪些不会冒泡的情况?事件冒泡(EventBubbling)是指在触发了某个元素的事件后,事件会从最内层元素开始沿着DOM树向上传递,直到最外层的元素,这种传递方式称为事件冒泡。但是,并不是所有的事件都能冒泡,有一些特殊情况下事件是不会冒泡的。本文将介绍在JavaScript中有哪些情况下事件不会冒泡。一、使用stopPropagati
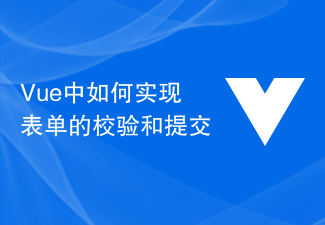
Vue中如何实现表单的校验和提交在Web开发中,表单是用户与网页进行交互的重要界面,表单中用户输入的数据需要进行校验和提交,以确保数据的合法性和完整性。Vue.js是一个流行的前端框架,它提供了便捷的表单校验和提交方法,使我们能够快速地实现表单功能。本文将介绍如何使用Vue.js来实现表单的校验和提交,并提供具体的代码示例。一、表单校验安装vee-valid
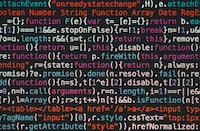
2.1使用CSRFTokenCSRFToken是一个随机生成的字符串,在用户会话中生成并存储,并在每个请求中随表单或链接一起发送。当服务器收到请求时,会验证CSRFToken是否与会话中的Token一致,如果不一致,则认为是CSRF攻击,并拒绝请求。2.2使用RefererHeaderRefererHeader是一个Http请求头,包含了请求来源的URL。服务器可以检查RefererHeader来确定请求是否来自合法来源。如果RefererHeader不存在或指向一个不合法来源,则认为是CSRF
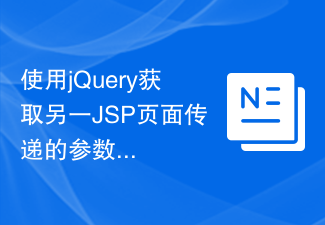
标题:使用jQuery查询另一个JSP页面传递的参数在开发Web应用程序时,经常会遇到需要在一个JSP页面中获取另一个JSP页面传递过来的参数的情况。这时候,可以借助jQuery来实现这一功能。下面将介绍如何使用jQuery查询另一个JSP页面传递的参数,并给出具体的代码示例。首先,我们需要明确一点,JSP页面之间传递参数一般有两种方式:一种是通过URL参数
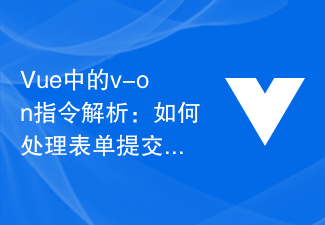
Vue中的v-on指令解析:如何处理表单提交事件在Vue.js中,v-on指令用于绑定事件监听器,可以捕获并处理各种DOM事件。其中,处理表单提交事件是Vue中常见的操作之一。本文将介绍如何使用v-on指令处理表单提交事件,并提供具体的代码示例。首先,需要明确Vue中的表单提交事件指的是当用户点击submit按钮或按下回车键时触发的事件。在Vue中,可以通过
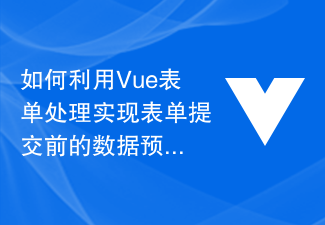
如何利用Vue表单处理实现表单提交前的数据预处理概述:在Web开发中,表单是平常最常见的元素之一。而在表单提交前,我们经常需要对用户输入的数据进行一些预处理,例如格式校验、数据转换等。Vue框架提供了方便易用的表单处理功能,本文将介绍如何利用Vue表单处理实现表单提交前的数据预处理。一、创建Vue实例和表单控件首先,我们需要创建一个Vue实例并定义一个包含表


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
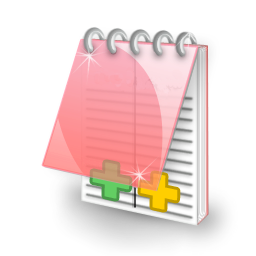
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use