


Python implements page simulation click and scroll function analysis for headless browser collection applications
Python implements page simulation click and scroll function analysis for headless browser collection applications
When collecting network data, we often encounter the need to simulate user operations. Such as clicking buttons, drop-down scrolling, etc. A common way to achieve these operations is to use a headless browser.
A headless browser is actually a browser without a user interface that simulates user operations through programming. The Python language provides many libraries to implement headless browser operations, the most commonly used of which is the selenium library.
The selenium library is a very powerful network automation testing tool in the Python language. It can simulate user operations in the browser, including clicking buttons, filling out forms, drop-down scrolling, etc. Below we will introduce how to use the selenium library to implement page simulation click and scroll functions.
First, we need to install the selenium library in the Python environment. You can use the pip command to install:
pip install selenium
Next, we need to download the corresponding headless browser driver. The selenium library supports multiple browsers, such as Chrome, Firefox, etc. Here we take Chrome as an example. You need to download the corresponding version of the Chrome driver and add it to the system environment variables.
from selenium import webdriver # 初始化Chrome浏览器驱动 driver = webdriver.Chrome() # 设置浏览器窗口大小 driver.set_window_size(1366, 768) # 打开网页 driver.get("https://www.example.com") # 模拟点击按钮 element = driver.find_element_by_xpath("//button[@id='submit']") element.click() # 模拟输入文本框 input_element = driver.find_element_by_xpath("//input[@id='username']") input_element.send_keys("your_username") # 模拟下拉滚动 driver.execute_script("window.scrollTo(0, document.body.scrollHeight);") # 关闭浏览器 driver.quit()
In the above code, we first imported the webdriver module of the selenium library and initialized a Chrome browser driver. Then set the browser window size and open a web page. Next, we use xpath to locate the button element that needs to be clicked and simulate the click operation. At the same time, we can also locate the input box through xpath and simulate the input operation. Finally, the page is scrolled down by executing JavaScript code.
It should be noted that since selenium simulates real user operations, we need to ensure that the elements of the page have been fully loaded when performing page simulation operations. You can use the time module to add a delay wait to ensure that page elements are loaded.
In addition, selenium also supports some other common operations, such as obtaining element attributes, taking screenshots, etc. Code can be written according to actual needs.
In summary, Python needs to use the selenium library to implement the page simulation click and scroll function of a headless browser collection application, and simulate user operations by calling the browser driver. Through the above code examples, we can easily implement page simulation click and scroll functions, which is very useful for scenarios such as data collection.
The above is the detailed content of Python implements page simulation click and scroll function analysis for headless browser collection applications. For more information, please follow other related articles on the PHP Chinese website!
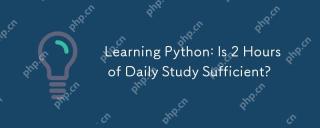
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
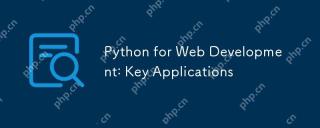
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
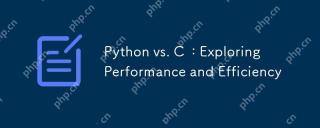
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
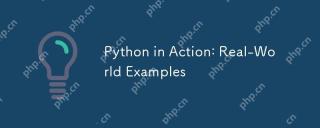
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
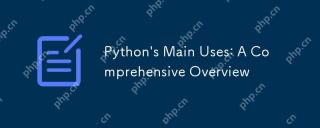
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
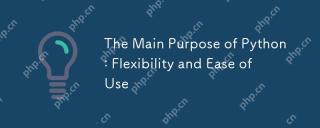
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
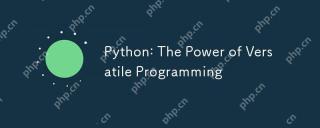
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
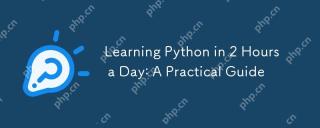
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
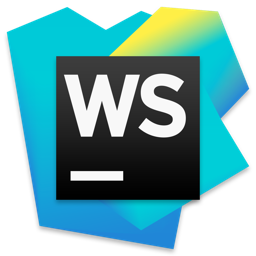
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor