Secure implementation of PHP encrypted transfer protocol
Secure implementation of PHP encrypted transmission protocol
Introduction:
With the rapid development of the Internet, data security has become an increasingly important issue. When transmitting sensitive data, encrypted transmission protocols are a common solution to ensure data confidentiality and integrity. This article will introduce how to implement a secure encrypted transmission protocol in PHP and provide corresponding code examples.
1. Implementation of HTTPS protocol
HTTPS (Hypertext Transfer Protocol Secure) is an encrypted transmission version of HTTP, which uses the SSL/TLS protocol to encrypt and decrypt data. In order to implement HTTPS in PHP, we need to install and enable an SSL certificate on the web server.
Sample code:
<?php // 启用HTTPS if(!isset($_SERVER['HTTPS']) || $_SERVER['HTTPS'] != 'on') { header("Location: https://" . $_SERVER['HTTP_HOST'] . $_SERVER['REQUEST_URI']); exit(); } // 其他PHP代码 ?>
The above code will redirect all HTTP requests to HTTPS requests and ensure that the data is transmitted through encryption.
2. Data Encryption and Decryption
In the process of transmitting data using the HTTPS protocol, the data will be encrypted and decrypted by default. But in some cases, we need to perform additional encryption and decryption operations on the data to improve security.
Sample code:
<?php // 数据加密 function encryptData($data, $key) { $iv = openssl_random_pseudo_bytes(openssl_cipher_iv_length('AES-256-CBC')); $encrypted = openssl_encrypt($data, 'AES-256-CBC', $key, 0, $iv); return base64_encode($encrypted . '::' . $iv); } // 数据解密 function decryptData($data, $key) { $parts = explode('::', base64_decode($data), 2); $decrypted = openssl_decrypt($parts[0], 'AES-256-CBC', $key, 0, $parts[1]); return $decrypted; } // 使用示例 $data = "Hello, World!"; $key = "my_secret_key"; $encryptedData = encryptData($data, $key); echo "加密后的数据: " . $encryptedData . " "; $decryptedData = decryptData($encryptedData, $key); echo "解密后的数据: " . $decryptedData . " "; ?>
The above code uses the AES-256-CBC algorithm to encrypt and decrypt data, and a secret key needs to be provided. The encrypted data will be Base64 encoded for easy transmission during transmission.
3. Security Certificate Verification
When using the HTTPS protocol to transmit data, in order to ensure the security of the connection, we need to verify the security certificate of the server. PHP provides some functions and options to implement certificate verification.
Sample code:
<?php // 创建一个cURL句柄 $ch = curl_init(); // 设置URL和其他cURL选项 curl_setopt($ch, CURLOPT_URL, "https://www.example.com/"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 2); // 执行cURL请求 $response = curl_exec($ch); // 处理响应内容 // ... // 关闭cURL句柄 curl_close($ch); ?>
The above code uses the cURL library to make HTTPS requests and enables certificate verification by setting the CURLOPT_SSL_VERIFYPEER
and CURLOPT_SSL_VERIFYHOST
options.
Conclusion:
It is crucial to protect the security of data during data transmission. By implementing the HTTPS protocol, data encryption and decryption, and security certificate verification, we can effectively protect the transmitted data and ensure its confidentiality and integrity. When writing secure applications using PHP, it is important to consider and implement these security measures.
The above is the detailed content of Secure implementation of PHP encrypted transfer protocol. For more information, please follow other related articles on the PHP Chinese website!
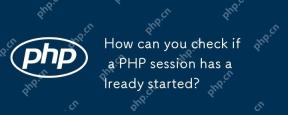
In PHP, you can use session_status() or session_id() to check whether the session has started. 1) Use the session_status() function. If PHP_SESSION_ACTIVE is returned, the session has been started. 2) Use the session_id() function, if a non-empty string is returned, the session has been started. Both methods can effectively check the session state, and choosing which method to use depends on the PHP version and personal preferences.
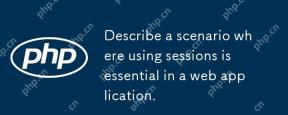
Sessionsarevitalinwebapplications,especiallyfore-commerceplatforms.Theymaintainuserdataacrossrequests,crucialforshoppingcarts,authentication,andpersonalization.InFlask,sessionscanbeimplementedusingsimplecodetomanageuserloginsanddatapersistence.
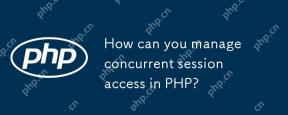
Managing concurrent session access in PHP can be done by the following methods: 1. Use the database to store session data, 2. Use Redis or Memcached, 3. Implement a session locking strategy. These methods help ensure data consistency and improve concurrency performance.
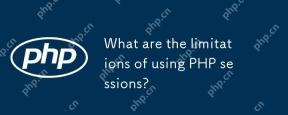
PHPsessionshaveseverallimitations:1)Storageconstraintscanleadtoperformanceissues;2)Securityvulnerabilitieslikesessionfixationattacksexist;3)Scalabilityischallengingduetoserver-specificstorage;4)Sessionexpirationmanagementcanbeproblematic;5)Datapersis
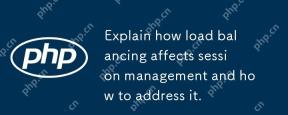
Load balancing affects session management, but can be resolved with session replication, session stickiness, and centralized session storage. 1. Session Replication Copy session data between servers. 2. Session stickiness directs user requests to the same server. 3. Centralized session storage uses independent servers such as Redis to store session data to ensure data sharing.
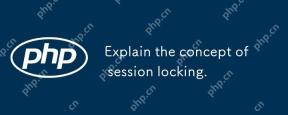
Sessionlockingisatechniqueusedtoensureauser'ssessionremainsexclusivetooneuseratatime.Itiscrucialforpreventingdatacorruptionandsecuritybreachesinmulti-userapplications.Sessionlockingisimplementedusingserver-sidelockingmechanisms,suchasReentrantLockinJ
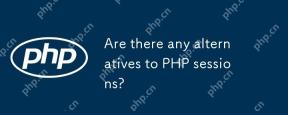
Alternatives to PHP sessions include Cookies, Token-based Authentication, Database-based Sessions, and Redis/Memcached. 1.Cookies manage sessions by storing data on the client, which is simple but low in security. 2.Token-based Authentication uses tokens to verify users, which is highly secure but requires additional logic. 3.Database-basedSessions stores data in the database, which has good scalability but may affect performance. 4. Redis/Memcached uses distributed cache to improve performance and scalability, but requires additional matching
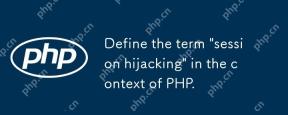
Sessionhijacking refers to an attacker impersonating a user by obtaining the user's sessionID. Prevention methods include: 1) encrypting communication using HTTPS; 2) verifying the source of the sessionID; 3) using a secure sessionID generation algorithm; 4) regularly updating the sessionID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
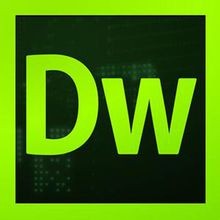
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
