


PHP form validation: password strength verification and rule settings
PHP form verification: password strength verification and rule setting
In modern network applications, user privacy and security are very important. Passwords are the first barrier to protecting user privacy. In order to ensure the security of the user's password, we need to implement password strength verification in the form for user registration or password change. This article will introduce how to use PHP to implement password strength verification and set password rules.
1. The necessity of password verification
In the form for user registration or password modification, password strength verification can effectively prevent users from using weak passwords and improve password security levels. Password strength verification usually includes the following aspects:
- Password length: The password length is usually required to be no less than 8 characters to ensure the complexity of the password.
- Character combination: Requires the password to contain uppercase and lowercase letters, numbers, and special characters to increase the difficulty of guessing the password.
- Avoid common passwords: prohibit users from using common passwords, such as "123456", "password", etc.
- Avoid consecutive characters: Users are prohibited from using consecutive characters, such as "abcdef", "123456", etc.
- Avoid repeated characters: Users are prohibited from using repeated characters, such as "aa", "1111", etc.
2. Password strength verification implementation
The following is a simple password strength verification sample code:
function checkPasswordStrength($password) { $strength = 0; // 校验密码长度 if (strlen($password) >= 8) { $strength += 1; } // 校验字符组合 if (preg_match('/[a-z]/', $password) && preg_match('/[A-Z]/', $password) && preg_match('/[0-9]/', $password) && preg_match('/[!@#$%^&*()-_=+]/', $password)) { $strength += 1; } // 校验是否包含常见密码 $commonPasswords = ['123456', 'password', 'qwerty']; if (!in_array($password, $commonPasswords)) { $strength += 1; } // 校验是否包含连续字符或重复字符 $lowercasePassword = strtolower($password); if (preg_match('/([a-z]){2,}/', $lowercasePassword) || preg_match('/(d){2,}/', $password)) { $strength -= 1; } return $strength; }
In this example, checkPasswordStrength
The function accepts a password as a parameter and returns the password's strength. The function checks whether the password meets the above password strength rules one by one, scores the password's strength based on the satisfaction of the rules, and finally returns the password's strength.
3. Password rule setting
According to specific needs, we can set password rules according to business needs. For example, we can define password rules by limiting password length, setting character combinations, etc. Here is a sample code:
function setPasswordRules() { $rules = [ 'minimum_length' => 8, // 密码最小长度 'require_lowercase' => true, // 是否需要小写字母 'require_uppercase' => true, // 是否需要大写字母 'require_numbers' => true, // 是否需要数字 'require_special_characters' => true, // 是否需要特殊字符 'forbidden_words' => ['password', 'qwerty', '123456'], // 禁止使用的常见密码 ]; return $rules; }
In this example, the setPasswordRules
function returns an array of password rules. We can set the minimum length of the password according to our needs, whether it needs to contain lowercase letters, uppercase letters, numbers, special characters, etc., as well as common passwords that need to be prohibited.
4. Application Example
The following is an application example showing how to implement password strength verification and rule settings through PHP on the registration page:
// 密码强度校验 $password = $_POST['password']; $strength = checkPasswordStrength($password); if ($strength < 2) { echo "密码强度不够,请重新设置密码"; exit; } // 密码规则设置 $rules = setPasswordRules(); if (strlen($password) < $rules['minimum_length']) { echo "密码长度过短,请重新设置密码"; exit; } if ($rules['require_lowercase'] && !preg_match('/[a-z]/', $password)) { echo "密码必须包含小写字母,请重新设置密码"; exit; } if ($rules['require_uppercase'] && !preg_match('/[A-Z]/', $password)) { echo "密码必须包含大写字母,请重新设置密码"; exit; } if ($rules['require_numbers'] && !preg_match('/[0-9]/', $password)) { echo "密码必须包含数字,请重新设置密码"; exit; } if ($rules['require_special_characters'] && !preg_match('/[!@#$%^&*()-_=+]/', $password)) { echo "密码必须包含特殊字符,请重新设置密码"; exit; } if (in_array($password, $rules['forbidden_words'])) { echo "密码不允许使用常见密码,请重新设置密码"; exit; } echo "密码设置成功";
In this example, we first obtain The password entered by the user, and the password strength is obtained through the checkPasswordStrength
function. Then, based on the password rules obtained by the setPasswordRules
function, we check whether the passwords comply with the rules one by one. If the password is not strong enough or does not comply with the rules, a corresponding error message will be output. If the password passes the verification, the prompt message "Password set successfully" will be output.
Through the above examples, we can implement simple and effective password strength verification and rule settings to improve the security of user passwords.
Summary
This article introduces how to use PHP to implement password strength verification and rule settings. Password strength verification can effectively prevent users from using weak passwords and improve password security. By setting password rules, we can limit the password length, character combinations, etc. according to specific needs to improve password complexity and security. In order to protect users' privacy and security, we should always pay attention to the security of passwords and fully consider user password protection measures during the development process.
The above is the detailed content of PHP form validation: password strength verification and rule settings. For more information, please follow other related articles on the PHP Chinese website!
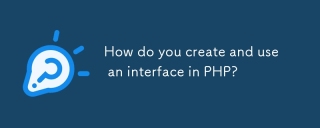
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
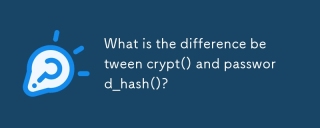
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
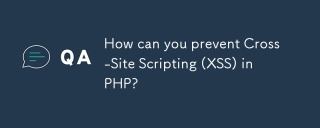
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.
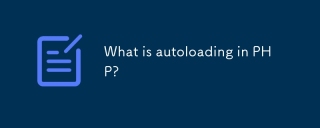
Autoloading in PHP automatically loads class files when needed, improving performance by reducing memory use and enhancing code organization. Best practices include using PSR-4 and organizing code effectively.
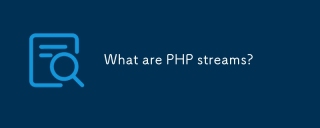
PHP streams unify handling of resources like files, network sockets, and compression formats via a consistent API, abstracting complexity and enhancing code flexibility and efficiency.
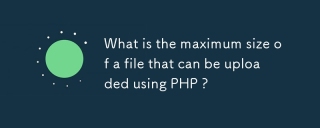
The article discusses managing file upload sizes in PHP, focusing on the default limit of 2MB and how to increase it by modifying php.ini settings.
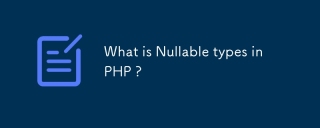
The article discusses nullable types in PHP, introduced in PHP 7.1, allowing variables or parameters to be either a specified type or null. It highlights benefits like improved readability, type safety, and explicit intent, and explains how to declar
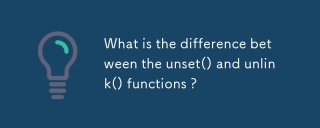
The article discusses the differences between unset() and unlink() functions in programming, focusing on their purposes and use cases. Unset() removes variables from memory, while unlink() deletes files from the filesystem. Both are crucial for effec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
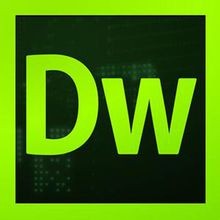
Dreamweaver CS6
Visual web development tools
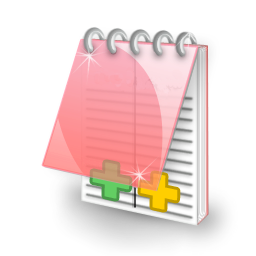
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
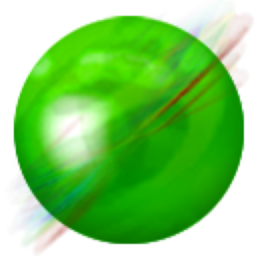
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
