XML data serialization and deserialization in Python
XML (Extensible Markup Language) is a format used to store and transmit data and is widely used Used in a variety of different fields. In Python, we can use the built-in xml library to serialize and deserialize XML data. This article will introduce how to use the xml library in Python to serialize and deserialize XML data, and provide relevant code examples.
XML serialization is the process of converting Python objects into XML format. The XML format enables data exchange between different systems and applications and is easy to read and parse. The xml library in Python provides the ElementTree module, which can conveniently perform serialization and deserialization operations on XML data.
First, we need to import the ElementTree module of the xml library:
import xml.etree.ElementTree as ET
Next, we can use the Element object of the ElementTree module to create an XML element. An element can be created by giving the element's name and attributes:
root = ET.Element("root") root.set("version", "1.0")
We can then use the SubElement method to create a sub-element under the root element:
child = ET.SubElement(root, "child") child.text = "Hello, World!"
By setting the element's attributes and text Content, we can create a simple XML structure. Next, we can use the ElementTree object to serialize the XML structure into a string:
xml_str = ET.tostring(root, encoding="utf-8").decode("utf-8") print(xml_str)
By calling the tostring method and specifying the encoding format, we can serialize the XML structure into a string and print it out. The output is as follows:
<root version="1.0"><child>Hello, World!</child></root>
In this example, we create a root element "root" and a child element "child", and set the text content of the child element.
Next, let’s look at how to deserialize XML data. Let's say we have an XML file that contains some data. We can use the parse method of the ElementTree module to parse an XML file and convert it into an Element object:
tree = ET.parse("data.xml") root = tree.getroot()
We can parse an XML file into an Element object by calling the parse method and passing in the path to the XML file. We can then get the root element of the XML file using the getroot method.
Next, we can use the properties and methods of the Element object to access and manipulate XML data. For example, we can use the find method to find an element with a specified name:
child = root.find("child") print(child.text)
By calling the find method and passing in the name of the element, we can find an element with a specified name. We can then use the text attribute to get the text content of the element and print it out.
Through the above code examples, you can see that the xml library in Python provides a simple and effective method to serialize and deserialize XML data. Whether you are serializing a Python object into an XML-formatted string or deserializing an XML file into an Element object, it can all be done easily. This will provide us with convenience and flexibility in processing XML data.
To summarize, this article introduces how to use the xml library in Python to serialize and deserialize XML data, and provides corresponding code examples. I hope these examples can help readers better understand and apply relevant knowledge of XML data processing.
The above is the detailed content of XML data serialization and deserialization in Python. For more information, please follow other related articles on the PHP Chinese website!
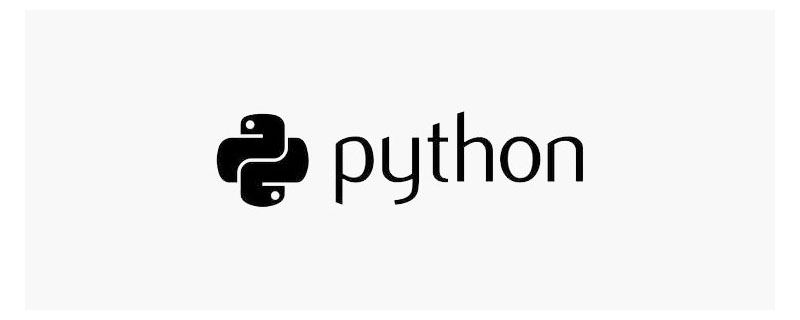
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
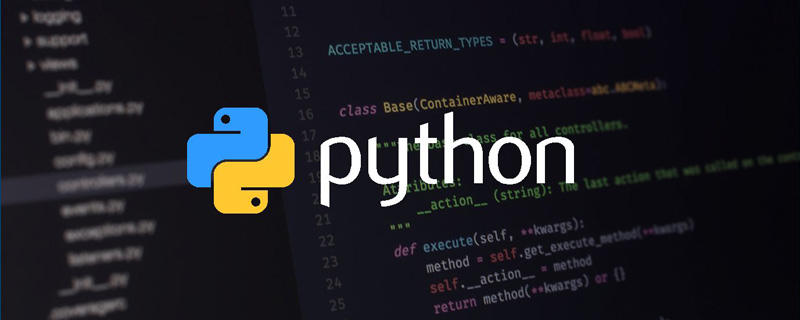
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
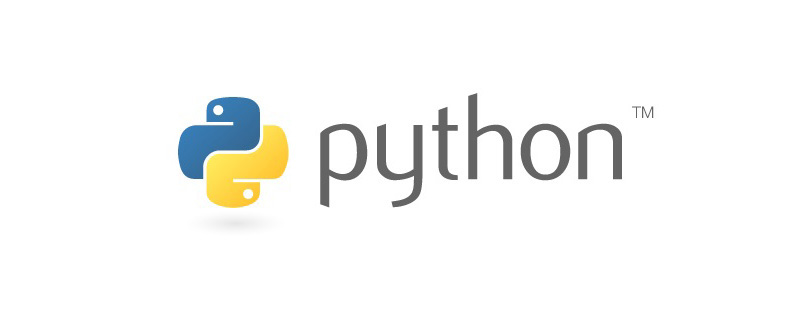
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
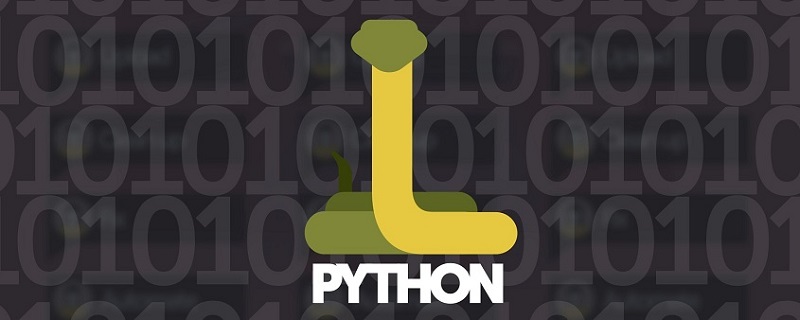
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
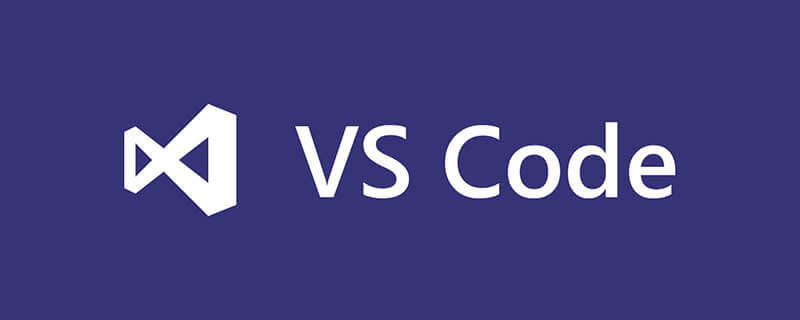
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
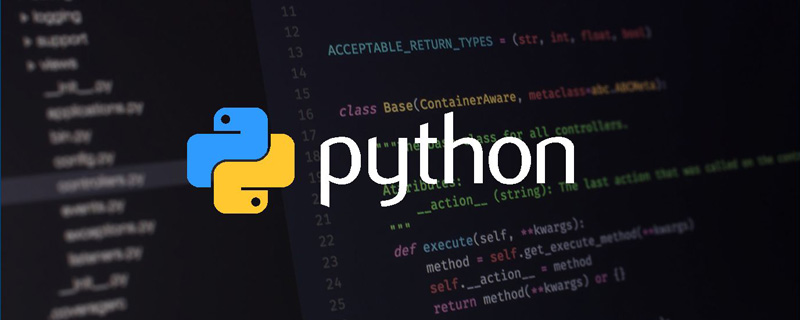
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
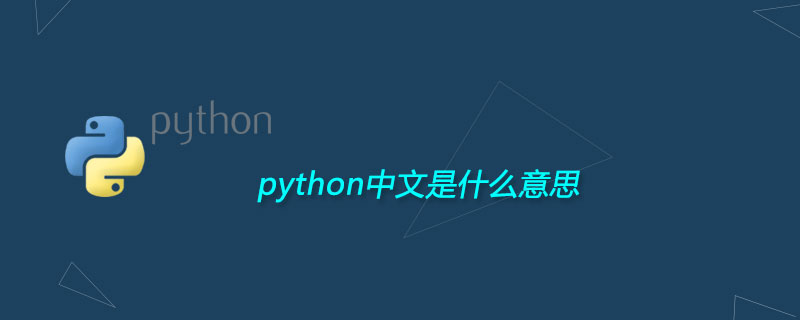
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
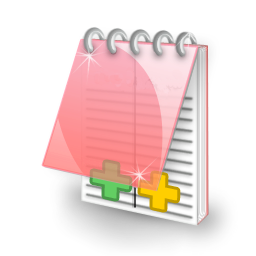
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
