Using PHP and XML to implement web crawler data analysis
Using PHP and XML to implement web crawler data analysis
Introduction:
With the rapid development of the Internet, there are massive data resources in the network. Data is important for analysis and research in many fields. As a common data collection tool, web crawlers can help us automatically crawl the required data from web pages. This article will introduce how to use PHP and XML to implement a web crawler and analyze the captured data.
1. Implementation of PHP web crawler
1. Step analysis
The implementation of PHP web crawler mainly includes the following steps:
(1) Obtain the HTML source code of the target web page;
(2) Parse the HTML source code and filter out the required data;
(3) Save the data.
2. Get the HTML source code
We can use PHP’s cURL extension library to get the HTML source code of the target web page, as shown below:
function getHtml($url){ $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $output = curl_exec($ch); curl_close($ch); return $output; }
3. Parse HTML and filter data
After obtaining the HTML source code, we need to use the DOMDocument extension library to parse the HTML and filter out the required data. The following is a simple example:
// 加载HTML源码 $html = getHtml("http://www.example.com"); // 创建DOMDocument对象并加载HTML $dom = new DOMDocument(); @$dom->loadHTML($html); // 获取标题 $title = $dom->getElementsByTagName("title")->item(0)->nodeValue; // 获取所有链接 $links = $dom->getElementsByTagName("a"); foreach($links as $link){ echo $link->getAttribute("href")." "; }
4. Save data
After filtering out the required data, we can choose to save the data to a database or XML file for subsequent analysis. Here we choose to save the data to an XML file, as shown below:
function saveDataToXML($data){ $dom = new DOMDocument("1.0", "UTF-8"); // 创建根节点 $root = $dom->createElement("data"); $dom->appendChild($root); // 创建数据节点 foreach($data as $item){ $node = $dom->createElement("item"); // 添加子节点,以及节点内容 $title = $dom->createElement("title", $item['title']); $node->appendChild($title); $link = $dom->createElement("link", $item['link']); $node->appendChild($link); $root->appendChild($node); } // 保存XML文件 $dom->save("data.xml"); }
2. Use XML for data analysis
1. Load the XML file
Before performing data analysis, we first need to load XML file and convert it into a DOMDocument object. The example is as follows:
$dom = new DOMDocument("1.0", "UTF-8"); @$dom->load("data.xml");
2. Parse XML data
After loading the XML file, we can use the DOMXPath extension library to parse the XML data to obtain the The data. The following is a simple example:
$xpath = new DOMXPath($dom); // 获取所有item节点 $items = $xpath->query("/data/item"); // 遍历item节点,输出title和link节点内容 foreach($items as $item){ $title = $item->getElementsByTagName("title")->item(0)->nodeValue; $link = $item->getElementsByTagName("link")->item(0)->nodeValue; echo "Title: ".$title." "; echo "Link: ".$link." "; }
3. Perform data analysis
After parsing the required data, we can perform various data analysis operations according to actual needs, such as counting the occurrence of a certain keyword frequency, data visualization, etc.
Conclusion:
By using PHP and XML, we can implement a simple web crawler and analyze the captured data. Using PHP's cURL extension library can easily obtain the HTML source code of the target web page, the DOMDocument extension library can help us parse HTML and XML data, and XPath can help us quickly locate and filter out the required data. In this way, we can make better use of network data resources and provide convenient data analysis methods for actual application scenarios.
Reference materials:
- PHP official documentation: http://php.net/manual/en/
- DOMDocument official documentation: http://php. net/manual/en/class.domdocument.php
- DOMXPath official document: http://php.net/manual/en/class.domxpath.php
The above is the detailed content of Using PHP and XML to implement web crawler data analysis. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
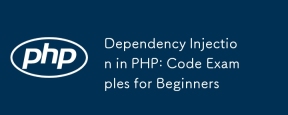
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
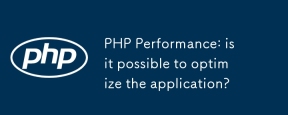
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
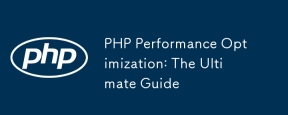
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
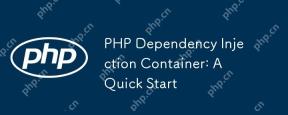
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
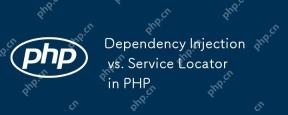
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
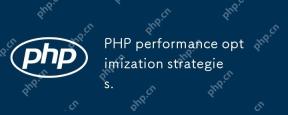
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
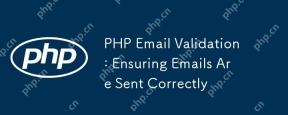
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
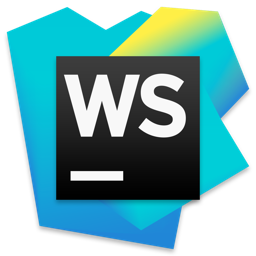
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
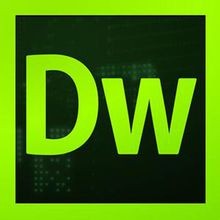
Dreamweaver CS6
Visual web development tools
