Use Python to implement data synchronization between XML and database
Introduction:
In the actual development process, it is often necessary to synchronize XML data with database data. XML is a commonly used data exchange format, and database is an important tool for storing data. This article will introduce how to use Python to achieve data synchronization between XML and database, and give code examples.
1. Basic concepts of XML and database
XML (Extensible Markup Language) is an extensible markup language used to describe the structure and content of data. It is characterized by being easy to read and understand, and has good cross-platform functionality. A database is a tool for storing and managing structured data that can be quickly queried and modified.
2. Data model of XML and database
XML describes data in the form of tags and uses elements and attributes to represent the structure and relationship of the data. A database uses tables, columns, and rows of data to organize and store data. When synchronizing data between XML and database, XML data needs to be mapped to the data model of the database.
3. Python implements data synchronization between XML and database
Python is a popular programming language that is concise and easy to read, and can easily handle XML and database operations. Below is an example of using Python to synchronize data between XML and a database.
-
Import related libraries
import xml.etree.ElementTree as ET import sqlite3
-
Parse XML files
def parse_xml(file_path): tree = ET.parse(file_path) root = tree.getroot() return root
-
Connect to database
def connect_database(db_path): conn = sqlite3.connect(db_path) cursor = conn.cursor() return conn, cursor
-
Create database table
def create_table(cursor): cursor.execute('''CREATE TABLE IF NOT EXISTS students (id INT PRIMARY KEY NOT NULL, name TEXT NOT NULL, age INT NOT NULL, grade CHAR(50));''')
-
Insert data into the database
def insert_data(cursor, id, name, age, grade): cursor.execute('''INSERT INTO students (id, name, age, grade) VALUES (?, ?, ?, ?)''', (id, name, age, grade))
-
Read XML data and insert into the database
def sync_data(root, cursor): for student in root.findall('student'): id = student.find('id').text name = student.find('name').text age = student.find('age').text grade = student.find('grade').text insert_data(cursor, id, name, age, grade)
-
Close the database connection
def disconnect_database(conn): conn.commit() conn.close()
-
Perform data synchronization
def sync_xml_to_database(xml_path, db_path): root = parse_xml(xml_path) conn, cursor = connect_database(db_path) create_table(cursor) sync_data(root, cursor) disconnect_database(conn)
IV. Summary
Through the above code examples, we can see that using Python to achieve data synchronization between XML and database is a simple and efficient way. By parsing XML files, connecting to the database, creating tables and inserting data into the database, we can store XML data into the database for query and modification. This approach is not only applicable to Python, but can also be implemented in other programming languages. Therefore, using Python is a good choice for development projects that require synchronization of XML and database data.
References:
- XML introduction: https://en.wikipedia.org/wiki/XML
- Database introduction: https://en.wikipedia .org/wiki/Database
The above is the detailed content of Data synchronization between XML and database using Python. For more information, please follow other related articles on the PHP Chinese website!
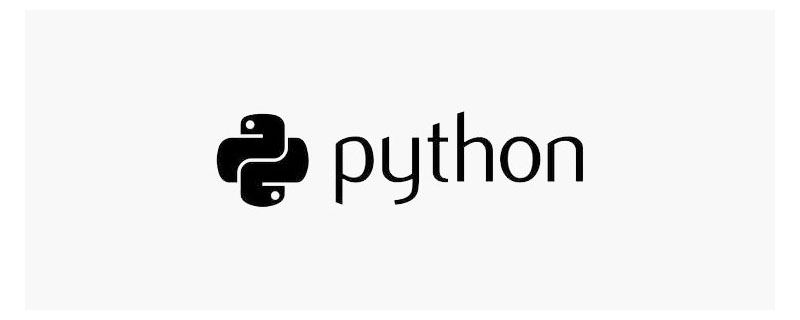
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
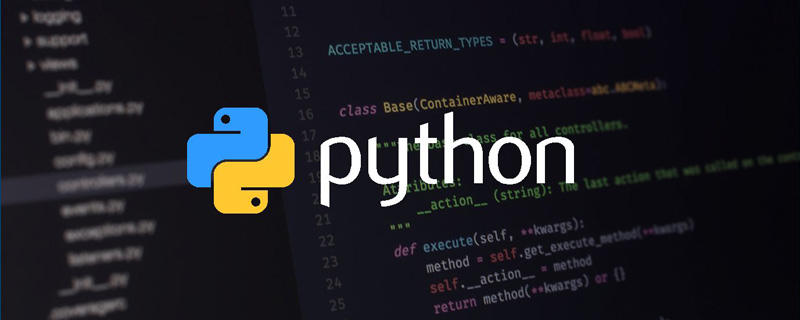
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
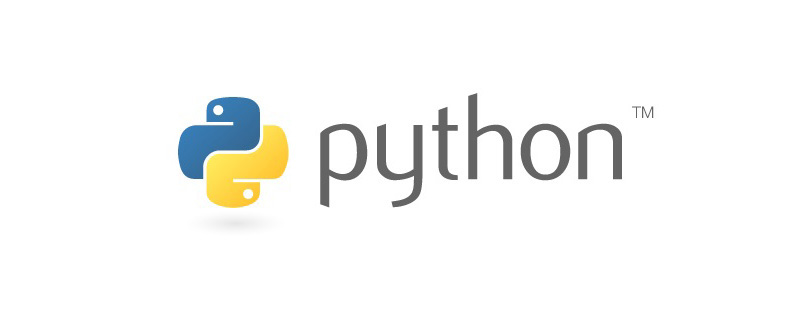
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
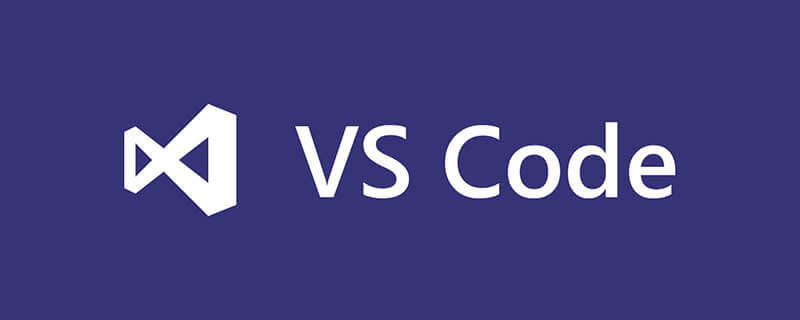
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
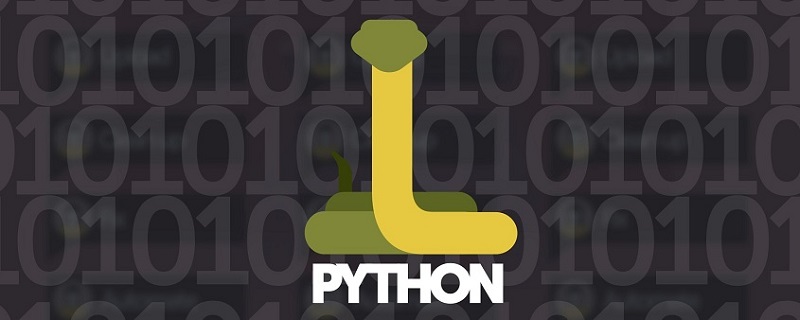
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
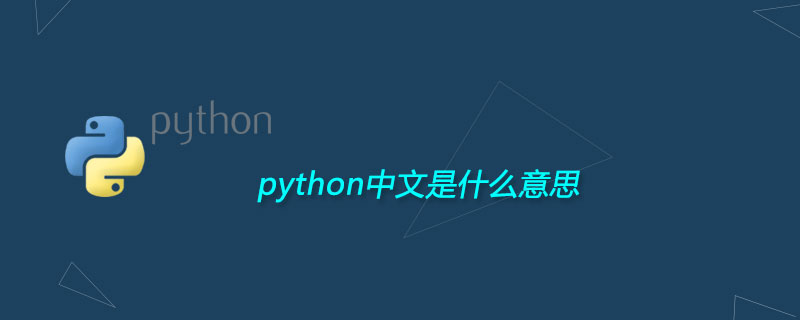
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。
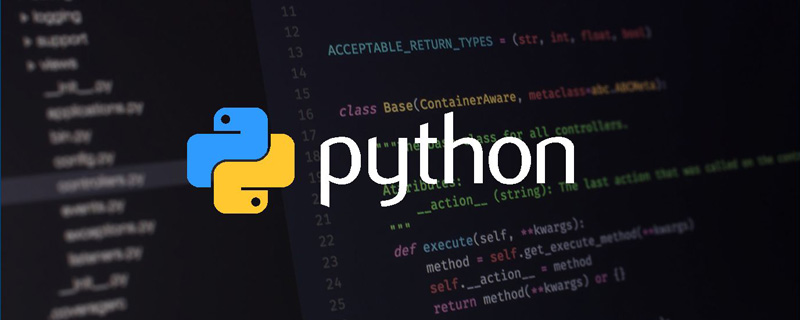
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
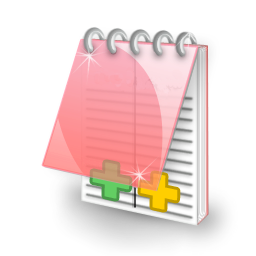
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use
