Using Channels to implement the producer-consumer model in Golang
Use Channels to implement the producer-consumer model in Golang
In concurrent programming, the producer-consumer model is a common design pattern used to solve asynchronous communication between producers and consumers. question. Golang provides a powerful Channels concurrency model, making it very simple and efficient to implement the producer-consumer model. In this article, we will introduce how to implement the producer-consumer model using Channels and illustrate it with code examples.
1. Understand the producer-consumer model
The producer-consumer model means that multiple producers concurrently produce data into a shared buffer, and multiple consumers concurrently produce data from Consume data in this buffer. Among them, the producer is responsible for adding data to the buffer, and the consumer is responsible for removing data from the buffer for processing.
A core problem of the producer-consumer model is that when the buffer is empty, the consumer must wait for the producer to generate data; and when the buffer is full, the producer must wait for the consumer to consume data. In order to solve this problem, we can use Channels to achieve synchronization and communication between producers and consumers.
2. Golang Channels
In Golang, Channel is a built-in type used for communication and synchronization between multiple Goroutines. Channel can be used to send and receive data, and the data type needs to be specified when creating it.
You can create a Channel in the following ways:
channel := make(chan <数据类型>)
To send data to the Channel, you can use the operator:
channel <- 数据
From the Channel To receive data in the Channel, you can use the operator:
数据 <- channel
If there is no data to receive in the Channel, the receiving operation will block the current Goroutine until there is data to receive. If the Channel is full, the send operation will block the current Goroutine until space becomes available.
3. Code example
The following is a code example that uses Channels to implement the producer-consumer model.
package main import ( "fmt" "time" ) func producer(ch chan<- int) { for i := 0; i < 5; i++ { ch <- i fmt.Println("生产者生产数据:", i) time.Sleep(time.Second) } close(ch) } func consumer(ch <-chan int) { for { data, ok := <-ch if !ok { fmt.Println("消费者消费完数据,退出") break } fmt.Println("消费者消费数据:", data) time.Sleep(2 * time.Second) } } func main() { ch := make(chan int, 3) go producer(ch) go consumer(ch) time.Sleep(10 * time.Second) }
In the above code, we create a buffer Channel ch
of size 3. The producer function producer
is used to produce data into the Channel and close the Channel through close(ch)
, indicating that the data production is completed. Consumer function consumer
is used to consume data from the Channel until the Channel is closed.
In the main()
function, we created a Goroutine to call the producer and consumer functions respectively, and then let the program run at a certain time through time.Sleep()
Exit after time.
Running the above code, we can see that the producer continuously produces data and sends it to the Channel, and the consumer continuously receives and consumes data from the Channel. The output result is similar to:
生产者生产数据: 0 消费者消费数据: 0 生产者生产数据: 1 消费者消费数据: 1 生产者生产数据: 2 消费者消费数据: 2 ... 消费者消费完数据,退出
4. Summary
Through the introduction of this article, we have understood the concept of the producer-consumer model and learned how to implement this model using Golang's Channels. Using Channels can simplify synchronization and communication issues in concurrent programming and improve program efficiency and readability. I hope the content of this article is helpful to you, and you are welcome to continue learning and exploring more knowledge about concurrent programming in Golang.
The above is the detailed content of Using Channels to implement the producer-consumer model in Golang. For more information, please follow other related articles on the PHP Chinese website!
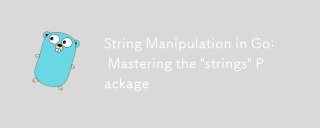
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
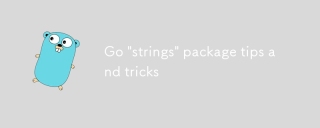
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
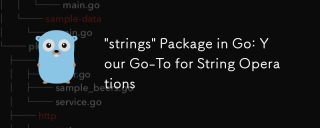
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
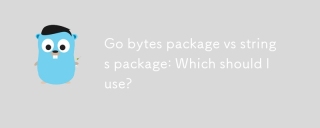
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
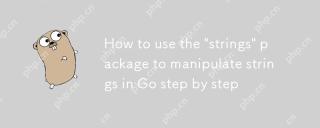
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
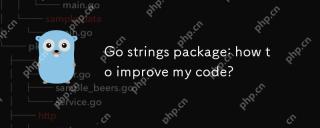
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
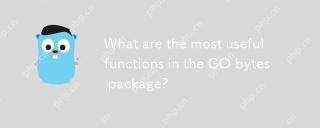
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
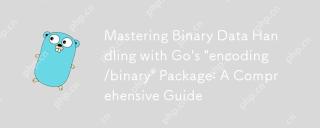
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
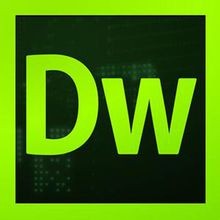
Dreamweaver CS6
Visual web development tools
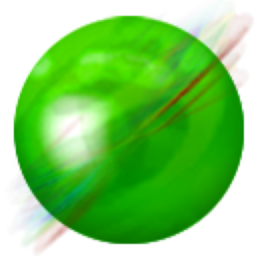
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
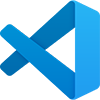
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
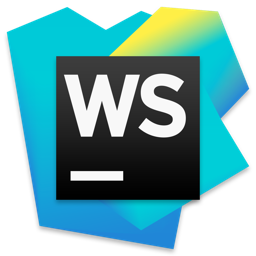
WebStorm Mac version
Useful JavaScript development tools
