How to use Java to implement the image cropping function of CMS system
How to use Java to implement the image cropping function of CMS system
1. Introduction
In the development process of modern web pages and mobile applications, image processing and display are an indispensable part. However, in many cases we need to crop images to fit different size and layout requirements. In this article, we will introduce how to use Java to write code to implement the image cropping function in a simple CMS system.
2. Basic Principle
Picture cropping refers to cutting out the required part from the original picture according to the predefined size and position. The key to achieving image cropping is to achieve the cropping operation by calculating and adjusting the size and position information of the original image.
3. Code Example
The following is a Java-based code example that shows how to use Java's image processing library to perform image cropping operations. In the example, we use the ImageIO class and the BufferedImage class to complete the cropping operation.
import javax.imageio.ImageIO; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; public class ImageCropper { public static void main(String[] args) { String inputImagePath = "input.jpg"; String outputImagePath = "output.jpg"; int cropWidth = 300; int cropHeight = 200; int cropX = 100; int cropY = 50; try { File inputFile = new File(inputImagePath); BufferedImage inputImage = ImageIO.read(inputFile); // 裁剪图像 BufferedImage croppedImage = inputImage.getSubimage(cropX, cropY, cropWidth, cropHeight); // 保存裁剪后的图像 File outputFile = new File(outputImagePath); ImageIO.write(croppedImage, "jpg", outputFile); System.out.println("图片裁剪完成!"); } catch (IOException e) { e.printStackTrace(); } } }
In the above code, we first specify the path of the input image (inputImagePath) and the path of the output image (outputImagePath), and then set the width (cropWidth), height (cropHeight), and start of the cropping area. The abscissa (cropX) and ordinate (cropY) of the starting position.
According to the set parameters, we read the original image from the input path through the read method of the ImageIO class, and obtain the cropped BufferedImage object (croppedImage) using the getSubimage method. Finally, use the write method of the ImageIO class to write the cropped image to the output path.
4. Precautions for use
- Make sure that the relevant Java image processing libraries, such as ImageIO and BufferedImage, have been imported when running the sample code.
- In actual applications, the code should be customized and optimized according to specific project requirements, and functions such as error handling and image size adaptation should be added.
5. Summary
This article introduces how to use Java to implement the image cropping function in the CMS system, and demonstrates the basic image cropping operation through sample code. I hope this article can be helpful to developers who need to implement similar functions. At the same time, it is also recommended that developers customize and optimize according to specific needs in actual applications to improve the stability and scalability of functions.
The above is the detailed content of How to use Java to implement the image cropping function of CMS system. For more information, please follow other related articles on the PHP Chinese website!
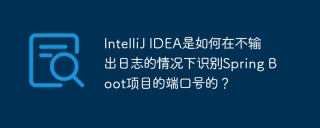
Start Spring using IntelliJIDEAUltimate version...
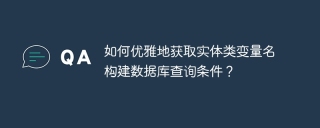
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
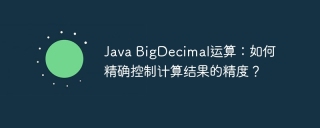
Java...
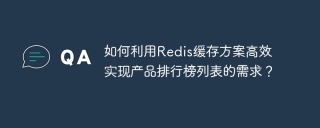
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
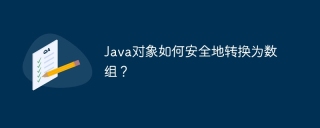
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
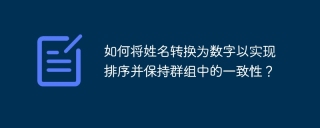
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
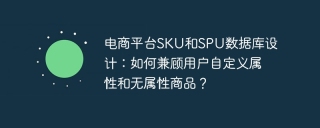
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
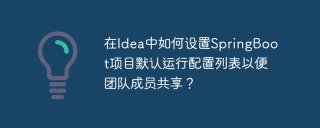
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
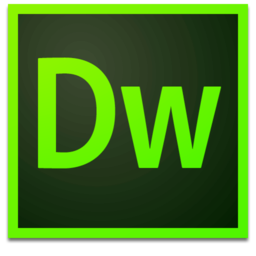
Dreamweaver Mac version
Visual web development tools
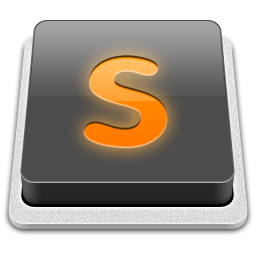
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
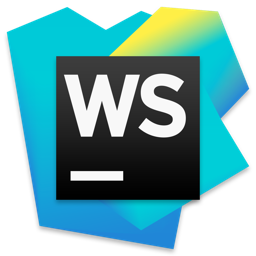
WebStorm Mac version
Useful JavaScript development tools