


Practical exploration of Java Baidu Translation API to realize mutual translation between Chinese and Czech
Introduction: With the development of globalization, exchanges and cooperation between different countries have become increasingly frequent. Language is an important tool for people to communicate, so language translation services have become particularly important. Baidu Translation API is an open platform based on machine translation, providing multi-lingual translation services. This article will use Java programming language to realize practical exploration of mutual translation between Chinese and Czech by calling Baidu Translation API.
1. Obtain the application ID and key of Baidu Translation API
Before using Baidu Translation API, we first need to apply for an application on Baidu Translation Open Platform and obtain the application ID and key key. The specific steps are as follows:
1. Visit Baidu Translation Open Platform (http://api.fanyi.baidu.com/), register a developer account, log in and enter the "My Application" page.
2. Click "Create Application", fill in the application name and description and other relevant information, and click OK to complete the creation.
3. On the "My Application" page, find the application you just created and click "API Information" to get the application ID and key.
2. Add dependencies on Baidu Translation API
To use Baidu Translation API in a Java project, we need to add the corresponding dependencies first. In the project's pom.xml file, add the following dependencies:
<dependency> <groupId>com.baidu.aip</groupId> <artifactId>java-sdk</artifactId> <version>4.7.0</version> </dependency>
3. Implement Chinese translation into Czech
Below we use a simple example to demonstrate how to achieve Chinese translation into Czech . First, we need to write a Java class named BaiduTranslator, the code is as follows:
import com.baidu.aip.translation.AipTranslation; import org.json.JSONObject; public class BaiduTranslator { // 设置APPID/AK/SK public static final String APP_ID = "your_app_id"; public static final String API_KEY = "your_api_key"; public static final String SECRET_KEY = "your_secret_key"; public static void main(String[] args) { // 初始化一个AipTranslation AipTranslation client = new AipTranslation(APP_ID, API_KEY, SECRET_KEY); // 设置可选参数 JSONObject options = new JSONObject(); options.put("from", "zh"); options.put("to", "cs"); // 翻译中文文本 String text = "你好,世界"; JSONObject result = client.translate(text, options); // 输出翻译结果 System.out.println(result.toString(2)); } }
In this class, we first set the application ID and key of Baidu Translator API. Then, we initialize an AipTranslation object and set the source language of the translation to Chinese ("zh") and the target language to Czech ("cs"). Next, we call the translate method, passing in the Chinese text to be translated and optional parameters for translation. Finally, we output the translation results.
Run this program and you will get the following output:
{ "error_code": 0, "error_msg": "SUCCESS", "from": "zh", "to": "cs", "trans_result": [ { "dst": "Ahoj, světe" } ] }
As can be seen from the output, the input Chinese text "Hello, World" is translated into Czech "Ahoy, svete".
4. Translate Czech to Chinese
Let’s translate Czech to Chinese. We only need to make simple modifications to the above code and set the source language and target language to Czech and Chinese. The modified code is as follows:
import com.baidu.aip.translation.AipTranslation; import org.json.JSONObject; public class BaiduTranslator { // 设置APPID/AK/SK public static final String APP_ID = "your_app_id"; public static final String API_KEY = "your_api_key"; public static final String SECRET_KEY = "your_secret_key"; public static void main(String[] args) { // 初始化一个AipTranslation AipTranslation client = new AipTranslation(APP_ID, API_KEY, SECRET_KEY); // 设置可选参数 JSONObject options = new JSONObject(); options.put("from", "cs"); options.put("to", "zh"); // 翻译捷克语文本 String text = "Ahoj, svete"; JSONObject result = client.translate(text, options); // 输出翻译结果 System.out.println(result.toString(2)); } }
Similarly, running the program will get the following output Result:
{ "error_code": 0, "error_msg": "SUCCESS", "from": "cs", "to": "zh", "trans_result": [ { "dst": "你好,世界" } ] }
As can be seen from the output result, the input Czech text "Ahoj, svete" is translated into Chinese "Hello, world".
Summary:
This article uses the Java programming language to achieve mutual translation between Chinese and Czech by calling Baidu Translation API. Through simple code examples, we can see the ease of use and accuracy of Baidu Translation API. I hope this article will be helpful to developers who want to use Baidu Translation API for language translation.
The above is the detailed content of Practical exploration of Java Baidu translation API to realize mutual translation between Chinese and Czech. For more information, please follow other related articles on the PHP Chinese website!
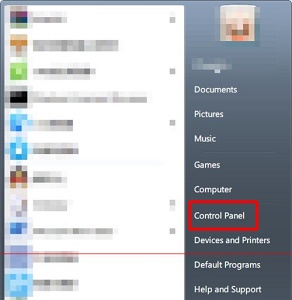
有些朋友可能会在安装系统时不小心设置成了英文,结果所有界面都变成了英文,看都看不懂。其实我们可以在控制面板中设置语言,将语言更改为中文,下面就一起来看一下更改的方法吧。win7如何更改语言为中文1、首先点击屏幕左下角的按钮,然后选择“ControlPanel”2、找到“Clock,Language,andRegion”下的“Changedispalylanguage”3、点击下方“English”就可以在下拉菜单中选择简体中文了。4、确定之后点击“Logoffnow”注销并重启电脑。5、回来之后
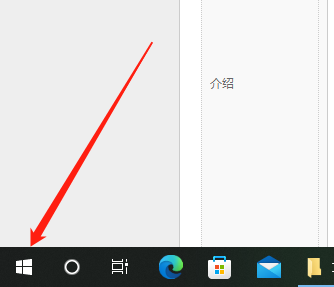
有时候我们再刚刚入手安装好电脑系统之后发现系统时英文的,遇到这种情况我们就需要把电脑的语言改成中文,那么win10系统里面该怎么把电脑的语言改成中文呢,现在就给大家带来具体的操作方法。win10电脑语言怎么改成中文1、打开电脑点击左下角的开始按键。2、点击左侧的设置选项。3、打开的页面选择“时间和语言”4、打开后,再点击左侧的“语言”5、在这里就可以设置你要的电脑语言。
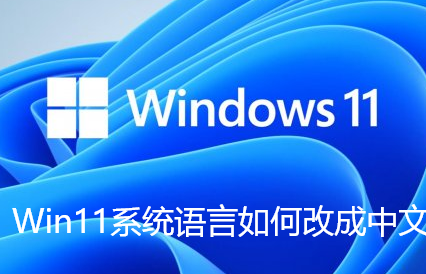
Win11系统语言如何改成中文?近期有用户刚给电脑安装了最新的Win11系统,但是在使用中发现系统语言为英文,自己使用起来很吃力,为此有没有什么方法可以将系统语言改成中文呢?方法很简单,下面我们来看看这篇Win11系统语言设置为中文的方法吧。 Win11系统语言设置为中文的步骤 1、首先我们进入齿轮按钮的settings,然后找到其中的Time打开时间和语言。 2、在时间和语言中点击左边栏的Language选项,然后在右侧点击Addalanguage。 3、接着在上方搜索框输入chi
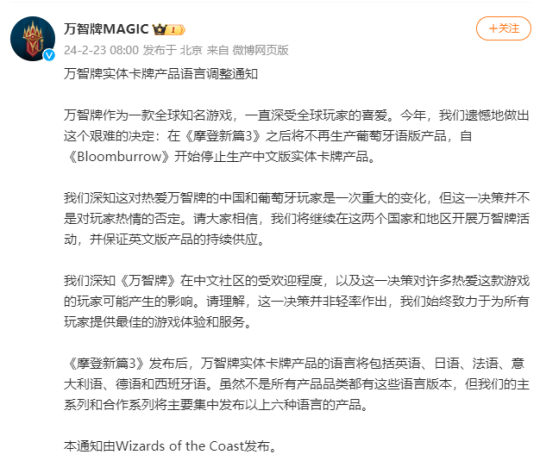
2月23日消息,官方威世智今日宣布,自《摩登新篇3》之后将不再生产葡萄牙语版产品,自《Bloomburrow》开始停止生产中文版实体卡牌产品。完整公告如下:万智牌实体卡牌产品语言调整通知万智牌作为一款全球知名游戏,一直深受全球玩家的喜爱。今年,我们遗憾地做出这个艰难的决定:在《摩登新篇3》之后将不再生产葡萄牙语版产品,自《Bloomburrow》开始停止生产中文版实体卡牌产品。我们深知这对热爱万智牌的中国和葡萄牙玩家是一次重大的变化,但这一决策并不是对玩家热情的否定。请大家相信,我们将继续在这两
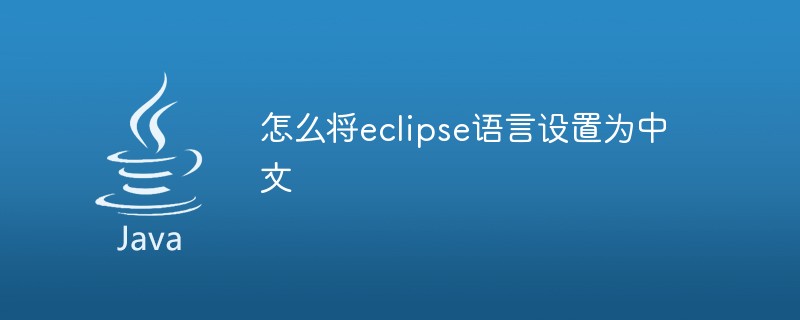
eclipse语言设置为中文的方法:1、打开浏览器找到语言包下载地址,并将最新的安装包地址复制;2、打开eclipse,点击“help”,然后点击安装新的插件;3、点击“Add”,在Location中粘帖网址;4、在下拉菜单中找到简体中文包,进行勾选,点击Next等待安装;5、重启eclipse即可。
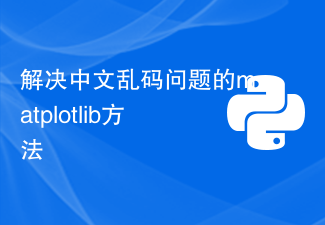
解决matplotlib中文乱码问题的方法,需要具体代码示例Matplotlib是一个常用的用于数据可视化的Python库,可以生成各种图表和图形。然而,对于中文用户来说,经常会遇到一个问题,就是生成的图表中的中文字符显示乱码。这个问题可以通过一些简单的方法来解决。本文将介绍一些常见的解决方法,并附上相关的代码示例,帮助读者解决这个烦人的问题。方法一:设置字
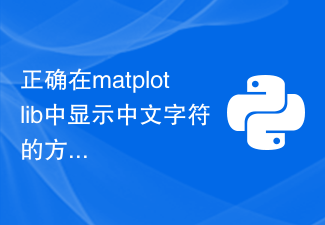
在matplotlib中正确地显示中文字符,是很多中文用户常常遇到的问题。默认情况下,matplotlib使用的是英文字体,无法正确显示中文字符。为了解决这个问题,我们需要设置正确的中文字体,并将其应用到matplotlib中。下面是一些具体的代码示例,帮助你正确地在matplotlib中显示中文字符。首先,我们需要导入需要的库:importmatplot
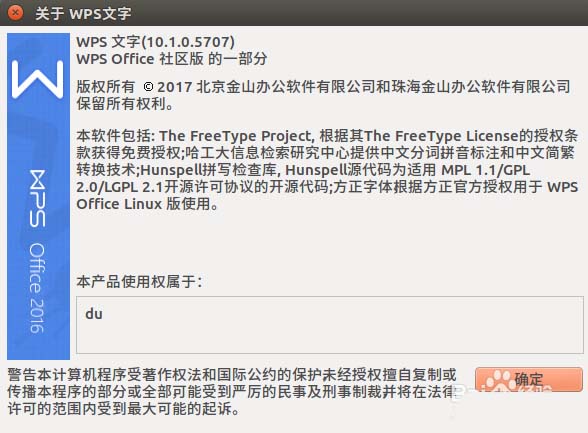
虽然Linux有LibreOffice,但是对微软的office兼容不是很好,有些排版会出现问题。而几年前,金山也开发了Linux版的WPS,不过在Ubuntu上使用,无法直接输入中文,这咋弄才可以让WPS正常输入中文呢1、打开WPS的文档,右上角的输入法已经是中文了,但是实际输入的时候,只能输入英文字母,出不了中文2、在终端输入:sudogedit/usr/bin/wps3、从第二行加上:exportXMODIFIERS="@im=fcitx"exportQT_IM_MODULE=&


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
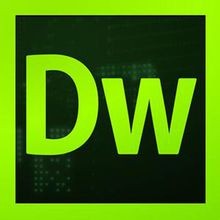
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
