How to use multi-threading to optimize the access efficiency of Java websites?
How to use multi-threading to optimize the access efficiency of Java websites?
With the rapid development of the Internet, Java is a powerful programming language, and more and more websites use Java as a development language. In the process of developing an efficient website, optimizing access efficiency is very important. This article will introduce how to use multi-threading to optimize the access efficiency of Java websites.
In order to better understand the optimization effect of multi-threading, we first need to understand threads in Java. A thread is an independently executed piece of code that has its own program counter, stack, and local variables. In Java, we can create threads by inheriting the Thread class or implementing the Runnable interface. In this article, we will create threads by implementing the Runnable interface.
When optimizing the access efficiency of Java websites, we generally use thread pools to manage threads. Thread pool is a thread reuse mechanism that can avoid frequent creation and destruction of threads, thereby reducing overhead. In Java, you can use the ThreadPoolExecutor class in the Executor framework to implement the thread pool function.
The following is a sample code that demonstrates how to use multi-threading to optimize the access efficiency of a Java website:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class WebSiteAccess { private static final int NUM_THREADS = 10; // 线程池中的线程数量 public static void main(String[] args) { ExecutorService executor = Executors.newFixedThreadPool(NUM_THREADS); // 创建一个固定数量的线程池 for (int i = 0; i < NUM_THREADS; i++) { executor.execute(new WebSiteTask()); // 提交任务到线程池 } executor.shutdown(); // 关闭线程池 } } class WebSiteTask implements Runnable { @Override public void run() { // 此处编写访问网站的代码 // ... } }
In the above code, we create a fixed number of thread pools of 10, and then loop Submit the WebSiteTask task to the thread pool. In WebSiteTask, we can write code to access the website.
By using multi-threads and thread pools, we can achieve the effect of accessing multiple websites at the same time, thus improving the efficiency of website access. In actual applications, the size of the thread pool can be adjusted according to specific needs and resource conditions.
In addition to using thread pools to manage threads, when optimizing the access efficiency of Java websites, you can also consider using concurrent collection classes to improve performance. Java provides some concurrent collection classes that can be used safely in multi-threaded environments, such as ConcurrentHashMap, ConcurrentLinkedQueue, etc. Using these concurrent collection classes can avoid problems such as race conditions and deadlocks under multi-threading.
To sum up, by using multi-threads and thread pools, we can effectively optimize the access efficiency of Java websites. In actual development, it is necessary to select the appropriate thread pool size and concurrent collection class based on specific needs and resource conditions to obtain the best performance optimization effect. I hope this article can be helpful to readers in optimizing the efficiency of Java website access.
The above is the detailed content of How to use multi-threading to optimize the access efficiency of Java websites?. For more information, please follow other related articles on the PHP Chinese website!
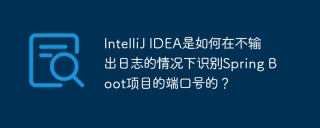
Start Spring using IntelliJIDEAUltimate version...
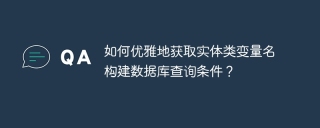
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
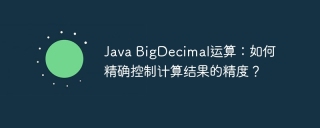
Java...
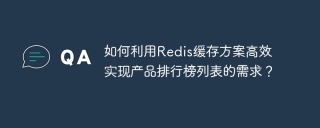
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
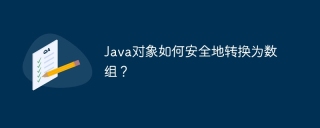
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
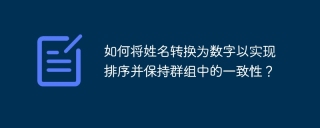
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
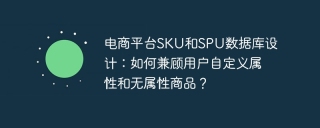
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
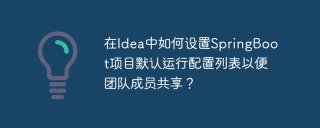
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.