How to use Java to implement the site map function of CMS system
How to use Java to implement the site map function of CMS system
With the rapid development of the Internet, more and more websites use CMS systems to manage and display content. As a technical means to assist website content management, the site map function has gradually become an indispensable part of the CMS system. This article will introduce how to use Java to implement the site map function of the CMS system and provide corresponding code examples.
Site map, as the name suggests, is to display the overall structure of the website in the form of a map. It can help webmasters better manage the content of the website, allowing users to intuitively understand the structure and content classification of the website. In a CMS system, a site map usually contains the following information: links to the homepage of the website, links to each column (category), links to articles (pages), etc.
To implement the site map function of the CMS system, you first need to obtain the structure and content information of the website. In Java, you can use the third-party crawler framework jsoup to obtain the HTML content of the website, and then parse out the link and content classification information. The following is a sample code for obtaining website links and content classification information:
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements; public class SiteMapGenerator { public static void main(String[] args) { // 网站URL String url = "http://www.example.com"; try { // 发送HTTP请求获取网页内容 Document doc = Jsoup.connect(url).get(); // 解析网页,获取所有链接 Elements links = doc.select("a[href]"); // 遍历链接,输出链接地址和文本 for (Element link : links) { String href = link.attr("href"); String text = link.text(); System.out.println(href + " - " + text); } } catch (Exception e) { e.printStackTrace(); } } }
Through the above code, we can obtain all links in the website and filter out links to columns and articles as needed. Next, we can generate a sitemap based on this information.
When generating a site map, the obtained links need to be organized according to a certain level and structure. You can use Java data structures to implement this function, such as using HashMap to represent columns (categories) and corresponding article link lists. The following is a sample code to generate a sitemap:
import java.util.HashMap; import java.util.Map; public class SiteMapGenerator { public static void main(String[] args) { // 构建站点地图 Map<String, String[]> siteMap = new HashMap<>(); siteMap.put("栏目1", new String[]{"文章1", "文章2"}); siteMap.put("栏目2", new String[]{"文章3", "文章4"}); // ... // 生成站点地图的XML字符串 String xml = generateSiteMapXML(siteMap); // 输出站点地图 System.out.println(xml); } private static String generateSiteMapXML(Map<String, String[]> siteMap) { // 使用StringBuilder拼接XML字符串 StringBuilder sb = new StringBuilder(); sb.append("<?xml version="1.0" encoding="UTF-8"?> "); sb.append("<siteMap> "); // 遍历栏目,生成XML节点 for (Map.Entry<String, String[]> entry : siteMap.entrySet()) { String category = entry.getKey(); String[] articles = entry.getValue(); sb.append(" <category> "); sb.append(" <name>").append(category).append("</name> "); // 遍历文章,生成XML节点 for (String article : articles) { sb.append(" <article>").append(article).append("</article> "); } sb.append(" </category> "); } sb.append("</siteMap>"); return sb.toString(); } }
With the above code, we can convert the sitemap into an XML format string and output it to the console. In practical applications, the XML string can be saved as a file or returned to the client as an HTTP response.
To sum up, we can obtain the structure and content classification information of the website by using Java's crawler framework jsoup, and use Java's data structure and string splicing technology to generate a site map. In this way, the site map function of the CMS system can be realized. Of course, in practical applications, issues such as site map updates and automatic generation also need to be considered, but the sample code provided in this article has provided a basic framework and ideas for implementing the site map function.
The above is the detailed content of How to use Java to implement the site map function of CMS system. For more information, please follow other related articles on the PHP Chinese website!
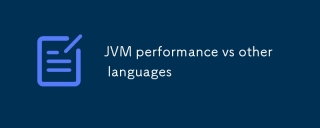
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
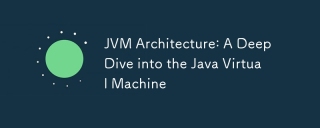
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
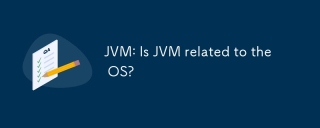
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
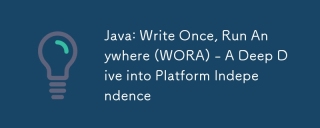
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
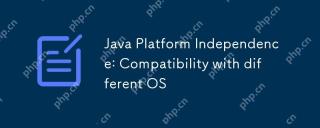
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
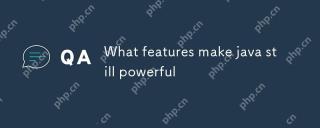
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
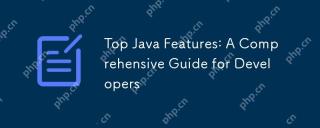
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
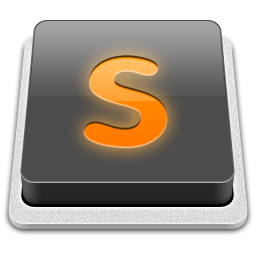
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
