How to use Vue for code analysis and debugging
How to use Vue for code analysis and debugging
In the Vue development process, code analysis and debugging are very important links. It helps us find potential problems and improve the quality of our code. This article will introduce how to use Vue for code analysis and debugging, with code examples.
1. Vue Devtools
Vue Devtools is a very powerful browser plug-in that can interact with Vue applications and provide many useful debugging functions. The following are the steps to use Vue Devtools:
- Install the Vue Devtools plugin. You can search for "Vue Devtools" in the Chrome browser plug-in market and install it. After the installation is complete, you can find the Vue Devtools option in the developer tools panel of your browser.
- Enable Vue Devtools in your Vue application. In the development environment, Vue Devtools is automatically enabled by default. If you are using Vue Devtools in a production environment, you need to enable it manually. Before your Vue instance is initialized, add the following code:
Vue.config.devtools = true;
- Open your Vue application and refresh the browser. You should now be able to see the Vue Devtools option in your browser's developer tools panel. By clicking on the option, you can enter the Vue Devtools interface.
- In Vue Devtools, you can see the various components, props, data, computed and other information of the Vue application. You can also view the component tree, event list, listeners, and component performance information. Using this information, you can better understand the state of your application at runtime and troubleshoot problems through debugging.
2. Code analysis of Vue CLI
Vue CLI is a powerful tool that can help us quickly build Vue applications. In addition to this, Vue CLI also provides some tools for code analysis.
- Install Vue CLI. You can install it by running the following command in the terminal:
npm install -g @vue/cli
- Run code analysis in your Vue project. Enter your project directory in the terminal, and then run the following command:
vue-cli-service build --report
This will generate an analysis report file named "report.html", showing the details of your code package, Including dependencies, file size, number of modules, etc. By analyzing the report, you can find the parts causing performance problems and optimize them.
3. Use Vue Devtools for performance analysis
Vue Devtools can be used not only for debugging, but also for performance analysis. It provides a performance panel that can help you find potential performance bottlenecks.
- Open Vue Devtools and switch to the performance panel.
- Perform some operations in your Vue application, such as clicking buttons, switching pages, etc. Vue Devtools will record the performance metrics of each operation.
- In the performance panel, you can see information such as the time taken for each operation, the number of component updates, and the number of DOM updates. You can also view the performance indicators of each component, such as initialization time, update time, etc.
By analyzing the data of the performance panel, you can find performance bottlenecks and take measures to optimize, such as using v-if/v-show to reduce unnecessary DOM operations, using v-for key attribute to improve the efficiency of list rendering, etc.
In summary, code analysis and debugging are very important for the development of Vue applications. By using Vue Devtools and the Vue CLI's profiling tools, we can better understand the runtime state of our application and troubleshoot potential issues. At the same time, performance analysis can help us find performance bottlenecks and optimize them. I hope this article can help you better use Vue for code analysis and debugging.
Code example:
<template> <div> <button @click="increaseCount">Click me</button> <p>{{ count }}</p> </div> </template> <script> export default { data() { return { count: 0, }; }, methods: { increaseCount() { this.count++; }, }, }; </script>
The above code is a simple Vue component, including a button and a counter. By clicking the button, the counter is incremented. You can view the status and events of this component and debug it in Vue Devtools.
The above is the detailed content of How to use Vue for code analysis and debugging. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.

Vue.js and React each have their own advantages: Vue.js is suitable for small applications and rapid development, while React is suitable for large applications and complex state management. 1.Vue.js realizes automatic update through a responsive system, suitable for small applications. 2.React uses virtual DOM and diff algorithms, which are suitable for large and complex applications. When selecting a framework, you need to consider project requirements and team technology stack.

Vue.js and React each have their own advantages, and the choice should be based on project requirements and team technology stack. 1. Vue.js is community-friendly, providing rich learning resources, and the ecosystem includes official tools such as VueRouter, which are supported by the official team and the community. 2. The React community is biased towards enterprise applications, with a strong ecosystem, and supports provided by Facebook and its community, and has frequent updates.

Netflix uses React to enhance user experience. 1) React's componentized features help Netflix split complex UI into manageable modules. 2) Virtual DOM optimizes UI updates and improves performance. 3) Combining Redux and GraphQL, Netflix efficiently manages application status and data flow.

Vue.js is a front-end framework, and the back-end framework is used to handle server-side logic. 1) Vue.js focuses on building user interfaces and simplifies development through componentized and responsive data binding. 2) Back-end frameworks such as Express and Django handle HTTP requests, database operations and business logic, and run on the server.

Vue.js is closely integrated with the front-end technology stack to improve development efficiency and user experience. 1) Construction tools: Integrate with Webpack and Rollup to achieve modular development. 2) State management: Integrate with Vuex to manage complex application status. 3) Routing: Integrate with VueRouter to realize single-page application routing. 4) CSS preprocessor: supports Sass and Less to improve style development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
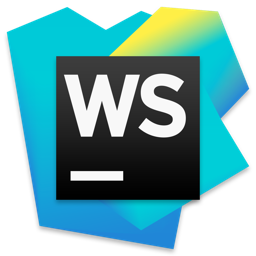
WebStorm Mac version
Useful JavaScript development tools
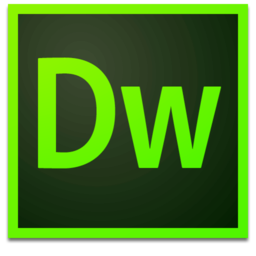
Dreamweaver Mac version
Visual web development tools
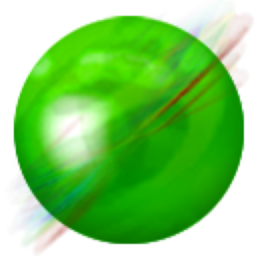
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
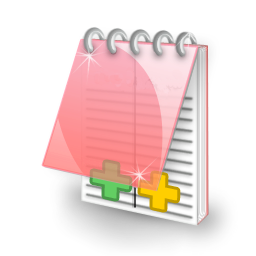
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
