


How to use machine learning libraries in Java to achieve intelligent data analysis and prediction?
How to use the machine learning library in Java to achieve intelligent data analysis and prediction?
With the rapid development of artificial intelligence and machine learning, more and more companies and research institutions are beginning to pay attention to and apply machine learning technology. As a widely used programming language, Java has also developed many mature machine learning libraries, such as Weka, DL4J, etc. This article will introduce how to use the machine learning library in Java to achieve intelligent data analysis and prediction, and provide detailed instructions with code examples.
First of all, we need to clarify some basic concepts of machine learning. Machine learning is a method of achieving tasks by learning models from data. It is mainly divided into supervised learning, unsupervised learning and reinforcement learning. In supervised learning, we need to have a set of data with known results, that is, labeled data, from which we can learn a model to predict unknown data. Unsupervised learning achieves tasks by discovering hidden patterns and structures in data without labeled data. Reinforcement learning achieves tasks through interactive learning between the agent and the environment.
Next, we will introduce several key steps to use the machine learning library in Java to achieve intelligent data analysis and prediction.
- Data preprocessing
Before performing machine learning, we need to preprocess the original data. This includes operations such as data cleaning, feature selection, and feature scaling. For example, for text data, we can first perform operations such as word segmentation, stop word removal, and part-of-speech restoration. The Weka library provides some common data preprocessing functions, such as standardization, dimensionality reduction, and discretization.
The following is a sample code for data preprocessing using the Weka library:
import weka.core.Instances; import weka.filters.Filter; import weka.filters.unsupervised.attribute.Normalize; import weka.filters.unsupervised.attribute.StringToWordVector; public class DataPreprocessing { public static void main(String[] args) throws Exception { // 读取数据 Instances data = new Instances(new FileReader("data.arff")); // 文本数据预处理,将文本转化为词向量 StringToWordVector filter = new StringToWordVector(); filter.setInputFormat(data); Instances vectorizedData = Filter.useFilter(data, filter); // 特征标准化 Normalize normalize = new Normalize(); normalize.setInputFormat(vectorizedData); Instances normalizedData = Filter.useFilter(vectorizedData, normalize); // 输出预处理后的数据 System.out.println(normalizedData); } }
- Model training
After preprocessing the data, we can use the machine learning library Provides algorithms to train the model. The Weka library provides many commonly used classification, regression and clustering algorithms, such as decision trees, support vector machines and K-means.
The following is a sample code for training a model using the Weka library:
import weka.core.Instances; import weka.classifiers.Classifier; import weka.classifiers.Evaluation; import weka.classifiers.functions.LinearRegression; public class ModelTraining { public static void main(String[] args) throws Exception { // 读取训练数据 Instances trainingData = new Instances(new FileReader("train.arff")); trainingData.setClassIndex(trainingData.numAttributes() - 1); // 构建线性回归模型 Classifier model = new LinearRegression(); model.buildClassifier(trainingData); // 评估模型 Evaluation evaluation = new Evaluation(trainingData); evaluation.evaluateModel(model, trainingData); // 输出模型的评估指标 System.out.println(evaluation.toSummaryString()); } }
- Model prediction
After the model training is completed, we can use the trained model to predict the unknown data for prediction. For classification problems, we can use models to predict the categories of data; for regression problems, we can use models to predict numerical results.
The following is a sample code for model prediction using the Weka library:
import weka.core.Instances; import weka.classifiers.Classifier; import weka.classifiers.functions.LinearRegression; public class ModelPrediction { public static void main(String[] args) throws Exception { // 读取测试数据 Instances testData = new Instances(new FileReader("test.arff")); testData.setClassIndex(testData.numAttributes() - 1); // 加载训练好的模型 Classifier model = (Classifier) weka.core.SerializationHelper.read("model.model"); // 对测试数据进行预测 for (int i = 0; i < testData.numInstances(); i++) { double prediction = model.classifyInstance(testData.instance(i)); System.out.println("预测结果:" + prediction); } } }
In summary, we can use the machine learning library in Java to achieve intelligent data analysis and prediction . This article briefly introduces key steps such as data preprocessing, model training, and model prediction, and explains it with the code examples provided by the Weka library. By learning and applying machine learning technology, we can better utilize data to generate valuable information and insights, thereby improving the intelligence of decision-making.
The above is the detailed content of How to use machine learning libraries in Java to achieve intelligent data analysis and prediction?. For more information, please follow other related articles on the PHP Chinese website!
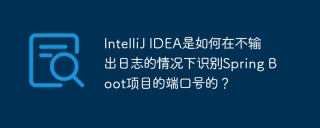
Start Spring using IntelliJIDEAUltimate version...
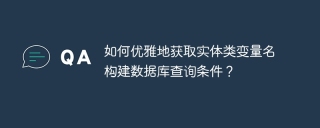
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
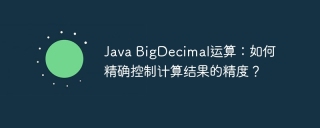
Java...
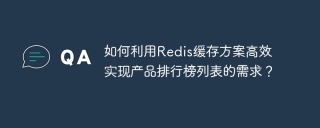
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
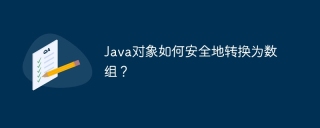
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
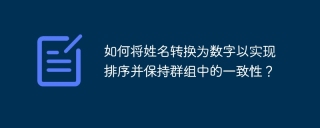
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
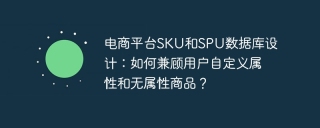
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
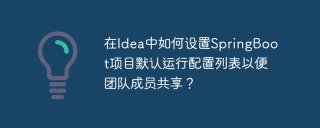
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
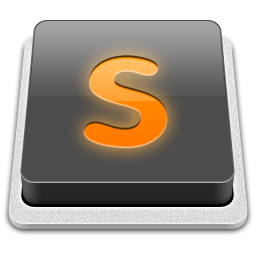
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor