How to use Go language for code reusability design
How to use Go language for code reusability design
Today, we will explore how to use Go language for code reusability design. Reusability is a key concept that helps us write code that is more elegant, easier to maintain, and easier to extend. In this article, we will discuss some design principles for reusability and give some code examples that implement these principles using Go language.
- Encapsulation (Encapsulation)
Encapsulation is an important concept in object-oriented programming and the key to achieving code reusability. Encapsulation refers to encapsulating data and methods for operating on data, hiding the internal implementation and providing a simple and easy-to-use interface to the outside world. In Go language, we can use structures to implement encapsulation.
package main import "fmt" type Person struct { name string age int } func (p Person) SayHello() { fmt.Println("Hello, my name is", p.name) } func main() { p := Person{name: "John", age: 25} p.SayHello() }
In the above example, we defined a structure named Person, which has two fields name and age. By defining methods on the structure, we can let the Person type have a SayHello() method and use the fields of the structure in this method. In this way, we achieve encapsulation of data and operations, thereby improving code reusability.
- Composition
Composition is a technique that combines existing types to form a new type. Using composition, we can take advantage of the functionality of existing types and implement new functionality by extending or modifying them. In Go language, we can use structure embedding to achieve composition.
package main import "fmt" type Animal struct { name string } func (a Animal) Eat() { fmt.Println(a.name, "is eating") } type Dog struct { Animal breed string } func main() { d := Dog{Animal: Animal{name: "Tom"}, breed: "Labrador"} d.Eat() }
In the above example, we defined a structure named Animal and defined an Eat() method for it. Then, we use structure embedding to embed the Animal type into the Dog type, so that the Dog type obtains the Eat() method of the Animal type. In this way, we can call the Eat() method in the Dog type without repeatedly implementing it.
- Interface (Interface)
An interface is a way of abstracting different types into a unified type. It defines a set of methods. Using interfaces, we can operate on objects without caring about the specific type. In the Go language, interfaces are very important, they can help us achieve code reusability.
package main import "fmt" type Animal interface { Eat() } type Dog struct { name string } func (d Dog) Eat() { fmt.Println(d.name, "is eating") } func Feed(a Animal) { a.Eat() } func main() { d := Dog{name: "Tom"} Feed(d) }
In the above example, we defined an Animal interface, which contains an Eat() method. Then, we define a Dog type and implement the Eat() method on this type. Finally, we define a Feed() function that accepts a parameter of Animal interface type and calls its Eat() method. In this way, we can use the Feed() function to feed objects of different types without caring about the specific types.
Through encapsulation, composition and interfaces, we can achieve code reusability in the Go language. These design principles can help us write code that is clearer, more flexible, and easier to maintain. In actual development, we should follow these principles and design our code based on actual needs to improve code reusability and scalability.
The above is the detailed content of How to use Go language for code reusability design. For more information, please follow other related articles on the PHP Chinese website!
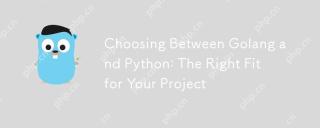
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
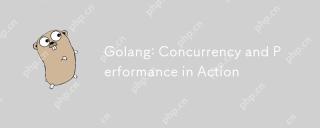
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
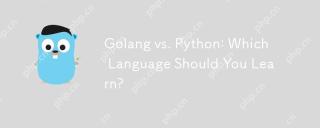
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
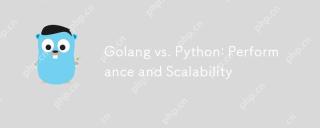
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
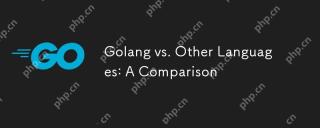
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
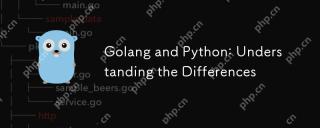
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
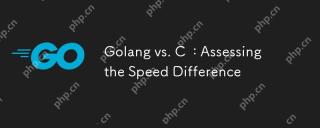
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
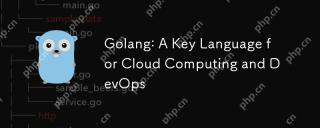
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
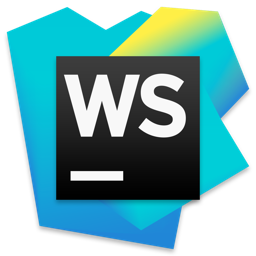
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.