How to use Go language for code security audit
In today's Internet era, code security audit is particularly important. With the rapid advancement of technology, hacker attacks are becoming more and more intelligent and complex. In order to protect the security of your own systems, networks and data, code security auditing has become an indispensable task. As an efficient, easy-to-use, and safe programming language, Go language is chosen by more and more companies and developers. This article will introduce how to use Go language for code security auditing, and provide some code examples to help readers better understand.
1. The importance of code security audit
Before conducting code security audit, we first need to understand the importance of code security audit. Through security audits of code, we can discover and repair potential loopholes and security risks, reduce the risk of being attacked by hackers, and improve the security and reliability of the system. Code security audit mainly includes inspection of input verification, output encoding, error handling, sensitive information protection, etc. in the code.
2. Steps to conduct code security audit using Go language
- Collecting code
First, we need to collect the code to be security audited. It can be code written by yourself, or code obtained from the open source community or other channels. - Analyze code structure
Analyze the collected code, understand the structure and logical relationship of the code, and find out the parts that may have security issues. Generally speaking, parts of the code related to user input, HTTP requests, database operations, file operations, etc. are prone to security risks. - Security vulnerability detection
Use the security scanning tools provided by the Go language, such as gosec, go-staticcheck, etc., to scan the code and detect possible security vulnerabilities. These tools can help us quickly discover potential problems in the code, such as unvalidated user input, buffer overflow, SQL injection, cross-site scripting attacks, etc. - Code specification check
Use static code analysis tools, such as golint, gofmt, etc., to check the code to ensure that the code complies with the specifications and best practices of the Go language. Good code specifications can reduce the probability of code errors and improve the readability and maintainability of the code. - Security Code Review
Conduct a detailed code review of parts of the code that are prone to security issues. Special attention needs to be paid to security-related code snippets such as user input processing, data encryption and decryption, file upload and download, cross-site request forgery, etc. in the code. Identify and fix potential vulnerabilities and security risks by carefully reviewing code. - Unit test and integration test
Write corresponding unit test and integration test cases to test whether the repaired code achieves the expected security effect. Ensure code runs properly under various scenarios and verify possible security vulnerabilities. - Security Vulnerability Repair
Repair the code based on the security vulnerabilities discovered during the audit. Repair methods include but are not limited to input validation, output encoding, error handling, permission control, etc. Ensure the code handles correctly when faced with various attacks.
3. Sample code
The following is a simple sample code to demonstrate how to use Go language for code security auditing.
package main import ( "fmt" "net/http" "os" ) func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } func handler(w http.ResponseWriter, r *http.Request) { filePath := r.URL.Path if filePath == "" { filePath = "index.html" } // 验证文件路径是否合法 if !isValidPath(filePath) { http.Error(w, "Invalid file path", http.StatusBadRequest) return } // 响应文件内容 content, err := readFile(filePath) if err != nil { http.Error(w, "Failed to read file", http.StatusInternalServerError) return } fmt.Fprintf(w, "%s", content) } func isValidPath(filePath string) bool { // 验证文件路径是否包含非法字符 invalidChars := []string{"..", "~", "*", "/./", "//"} for _, char := range invalidChars { if strings.Contains(filePath, char) { return false } } // 验证文件路径是否存在 _, err := os.Stat(filePath) if os.IsNotExist(err) { return false } return true } func readFile(filePath string) ([]byte, error) { file, err := os.Open(filePath) if err != nil { return nil, err } defer file.Close() stat, err := file.Stat() if err != nil { return nil, err } content := make([]byte, stat.Size()) _, err = file.Read(content) if err != nil { return nil, err } return content, nil }
The above sample code shows a simple file server that returns the contents of a specified file through an HTTP request. In the code, we verified the file path to ensure that the file path is legal and there are no security issues such as path traversal. In this way, the risk of being exploited by hackers can be reduced.
4. Summary
Code security audit is an important part of protecting system, network and data security. Using Go language to conduct code security audits can help developers promptly discover and repair potential security vulnerabilities and hidden dangers, and improve the security and reliability of the system. When conducting a code security audit, we need to collect code, analyze the code structure, use security scanning tools to detect vulnerabilities, conduct code specification checks, conduct code security reviews, and conduct unit testing and integration testing. Through the above steps, we can improve the security of the code and reduce the occurrence of security risks.
The above is the detailed content of How to use Go language for code security audit. For more information, please follow other related articles on the PHP Chinese website!
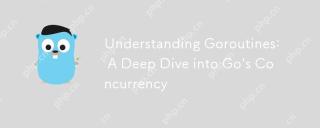
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
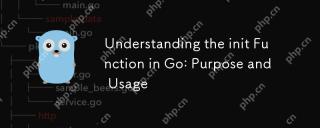
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
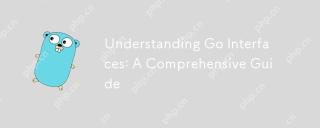
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
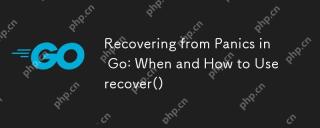
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
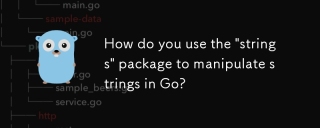
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
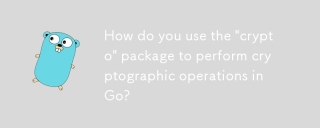
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
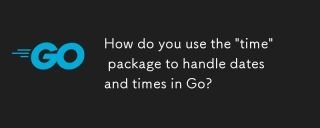
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
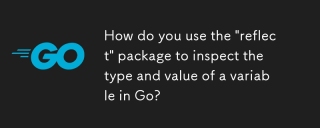
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
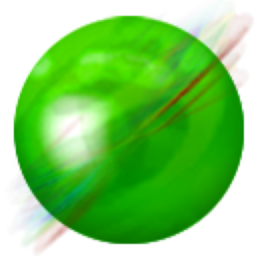
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
