How to dynamically expand and shrink data in MySQL?
How to dynamically expand and reduce data in MySQL?
MySQL is a commonly used relational database management system, and many applications use MySQL as the underlying database. In actual use, we often need to dynamically expand and shrink the database to cope with the increase or decrease in data volume. This article will introduce the method of dynamic expansion and reduction of data in MySQL and provide corresponding code examples.
- Extended fields of database tables
When you need to add new fields to an existing database table, you can use the ALTER TABLE statement to achieve this.
For example, suppose there is a table named person, which contains three fields: id, name and age, as shown below:
CREATE TABLE person (
id INT,
name VARCHAR(100),
age INT
);
Now we need to add a new field sex in the person table, which can be achieved through the following ALTER TABLE statement:
ALTER TABLE person
ADD COLUMN sex VARCHAR(10);
After running the above SQL statement, the structure of the person table will become:
id INT,
name VARCHAR(100),
age INT,
sex VARCHAR(10)
Note that the ALTER TABLE statement can also be used to modify the attributes of fields, such as modifying field types, adding constraints, etc.
- Reduce the fields of the database table
When you need to delete a field from the database table, you can use the DROP COLUMN clause of the ALTER TABLE statement to achieve this.
For example, suppose you need to delete the sex field from the person table, you can use the following ALTER TABLE statement:
ALTER TABLE person
DROP COLUMN sex;
After running the above SQL statement, the structure of the person table will become:
id INT,
name VARCHAR(100),
age INT
Note that the ALTER TABLE statement The DROP COLUMN clause can only delete one field. If you need to delete multiple fields, you need to use multiple DROP COLUMN clauses.
- Expand the number of rows in the database table
When you need to insert more data into the database table, you can use the INSERT INTO statement to achieve this.
For example, suppose you need to insert a new record into the person table, which can be achieved through the following INSERT INTO statement:
INSERT INTO person (id, name, age, sex)
VALUES (1, 'John', 25, 'Male');
After running the above SQL statement, a new record will be added to the person table:
id=1, name=' John', age=25, sex='Male'
Note that the INSERT INTO statement can also insert multiple records at one time, just specify multiple sets of parameters in the VALUES clause.
- Reduce the number of rows in the database table
When you need to delete certain records from the database table, you can use the DELETE FROM statement to achieve this.
For example, if you want to delete all records with female gender from the person table, you can use the following DELETE FROM statement:
DELETE FROM person
WHERE sex = 'Female' ;
After running the above SQL statement, all female records in the person table will be deleted.
Note that the DELETE FROM statement can also delete records based on other conditions. You only need to specify the corresponding conditions in the WHERE clause.
To sum up, MySQL provides a flexible way to dynamically expand and reduce data. By using the ALTER TABLE statement to expand or reduce the fields of the table, and using the INSERT INTO and DELETE FROM statements to expand or reduce the number of rows in the table, we can adjust the structure and content of the database according to actual needs.
I hope this article will be helpful for you to dynamically expand and reduce data in MySQL. If you have any questions or doubts, please leave a message for discussion.
The above is the detailed content of How to dynamically expand and shrink data in MySQL?. For more information, please follow other related articles on the PHP Chinese website!
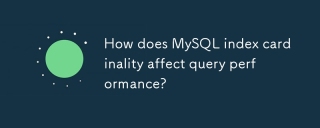
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
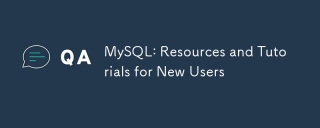
The MySQL learning path includes basic knowledge, core concepts, usage examples, and optimization techniques. 1) Understand basic concepts such as tables, rows, columns, and SQL queries. 2) Learn the definition, working principles and advantages of MySQL. 3) Master basic CRUD operations and advanced usage, such as indexes and stored procedures. 4) Familiar with common error debugging and performance optimization suggestions, such as rational use of indexes and optimization queries. Through these steps, you will have a full grasp of the use and optimization of MySQL.
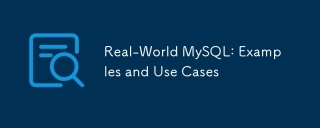
MySQL's real-world applications include basic database design and complex query optimization. 1) Basic usage: used to store and manage user data, such as inserting, querying, updating and deleting user information. 2) Advanced usage: Handle complex business logic, such as order and inventory management of e-commerce platforms. 3) Performance optimization: Improve performance by rationally using indexes, partition tables and query caches.
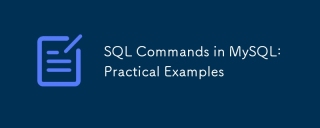
SQL commands in MySQL can be divided into categories such as DDL, DML, DQL, DCL, etc., and are used to create, modify, delete databases and tables, insert, update, delete data, and perform complex query operations. 1. Basic usage includes CREATETABLE creation table, INSERTINTO insert data, and SELECT query data. 2. Advanced usage involves JOIN for table joins, subqueries and GROUPBY for data aggregation. 3. Common errors such as syntax errors, data type mismatch and permission problems can be debugged through syntax checking, data type conversion and permission management. 4. Performance optimization suggestions include using indexes, avoiding full table scanning, optimizing JOIN operations and using transactions to ensure data consistency.
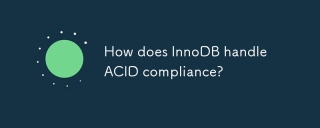
InnoDB achieves atomicity through undolog, consistency and isolation through locking mechanism and MVCC, and persistence through redolog. 1) Atomicity: Use undolog to record the original data to ensure that the transaction can be rolled back. 2) Consistency: Ensure the data consistency through row-level locking and MVCC. 3) Isolation: Supports multiple isolation levels, and REPEATABLEREAD is used by default. 4) Persistence: Use redolog to record modifications to ensure that data is saved for a long time.

MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
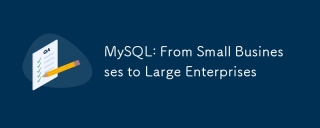
MySQL is suitable for small and large enterprises. 1) Small businesses can use MySQL for basic data management, such as storing customer information. 2) Large enterprises can use MySQL to process massive data and complex business logic to optimize query performance and transaction processing.
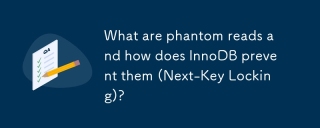
InnoDB effectively prevents phantom reading through Next-KeyLocking mechanism. 1) Next-KeyLocking combines row lock and gap lock to lock records and their gaps to prevent new records from being inserted. 2) In practical applications, by optimizing query and adjusting isolation levels, lock competition can be reduced and concurrency performance can be improved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
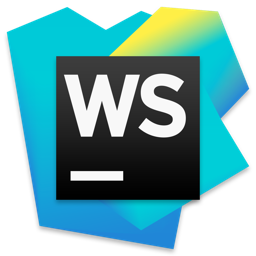
WebStorm Mac version
Useful JavaScript development tools
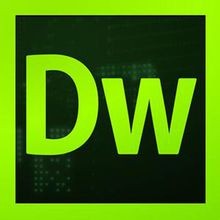
Dreamweaver CS6
Visual web development tools