OAuth in PHP: Creating a Secure API Gateway
With the popularity of web applications, more and more developers require the use of third-party APIs to enhance their application functionality. However, protecting APIs from unauthorized access stumps many developers. OAuth (Open Authorization) is a solution that allows applications to access third-party APIs through user authorization while protecting users' private data. This article will introduce how to create a secure API gateway using OAuth in PHP.
- The basic concept of OAuth
OAuth is an open standard that allows one application to access the user resources of another application without exposing the user's username and password. . OAuth uses access tokens to represent authorized application access to APIs. In order to obtain an access token, the application needs to go through an authorization process. The user will be redirected to the authorization server. After verifying the user's identity, the authorization server will issue the authorization token (authorization code) to the application, and the application will then use the authorization token. Exchange access tokens.
- Create a PHP project
First, we need to create a basic PHP project. In the root directory of the project, create a file named index.php
and add the following code:
<?php // 定义API网关的URL define('API_URL', 'https://api.example.com'); // 定义第三方应用的客户端ID和客户端密钥 define('CLIENT_ID', 'your_client_id'); define('CLIENT_SECRET', 'your_client_secret'); // 定义重定向URL define('REDIRECT_URI', 'https://yourdomain.com/callback.php'); // 重定向到授权服务器 header('Location: '.API_URL.'/oauth/authorize?client_id='.CLIENT_ID.'&redirect_uri='.urlencode(REDIRECT_URI).'&response_type=code');
- Implementing the authorization callback
Create a file named callback.php
to receive callback requests from the authorization server and exchange authorization tokens. In this file, add the following code:
<?php // 定义API网关的URL define('API_URL', 'https://api.example.com'); // 定义第三方应用的客户端ID和客户端密钥 define('CLIENT_ID', 'your_client_id'); define('CLIENT_SECRET', 'your_client_secret'); // 定义重定向URL define('REDIRECT_URI', 'https://yourdomain.com/callback.php'); // 获取授权令牌 if (isset($_GET['code'])) { $code = $_GET['code']; // 交换授权令牌 $url = API_URL . '/oauth/token'; $data = [ 'grant_type' => 'authorization_code', 'client_id' => CLIENT_ID, 'client_secret' => CLIENT_SECRET, 'redirect_uri' => REDIRECT_URI, 'code' => $code ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($data)); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); // 处理API响应 $json = json_decode($response, true); if (isset($json['access_token'])) { $access_token = $json['access_token']; // 在这里可以使用访问令牌来访问API // 例如:使用访问令牌调用API的/users接口 $url = API_URL . '/users'; $headers = [ 'Authorization: Bearer ' . $access_token ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($ch); curl_close($ch); // 处理API响应 $json = json_decode($response, true); var_dump($json); } }
- Code Analysis
First, we define the URL of the API gateway, the client ID of the third-party application, and the client key and the redirect URL is given. Then, in the index.php
file, we redirect to the authorization page of the authorization server. Next, in the callback.php
file, we obtain the authorization token passed by the authorization server callback. We then exchange the authorization token for the access token and use the access token to access the API.
- Summary
By using OAuth, we can create a secure API gateway that allows applications to access third-party APIs while protecting users' private data. This article demonstrates how to create a secure API gateway using OAuth in PHP and provides relevant code examples. I hope this article can help developers better understand and apply OAuth.
The above is the detailed content of OAuth in PHP: Create a secure API gateway. For more information, please follow other related articles on the PHP Chinese website!
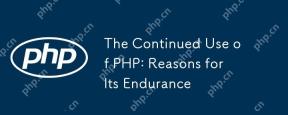
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
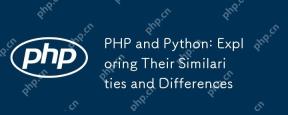
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
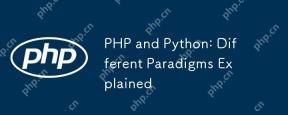
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
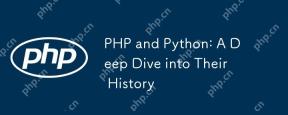
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
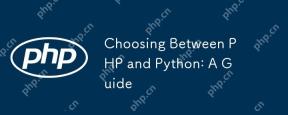
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
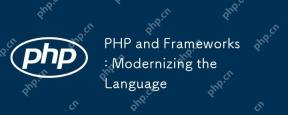
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
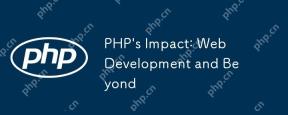
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
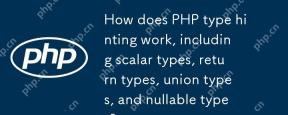
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
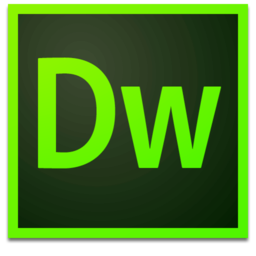
Dreamweaver Mac version
Visual web development tools
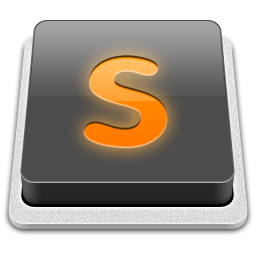
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
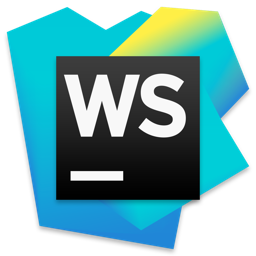
WebStorm Mac version
Useful JavaScript development tools