


Use PHP and XML to implement user registration and verification functions
Use PHP and XML to implement user registration and verification functions
In modern website development, user registration and verification are indispensable functions. This article will introduce how to use PHP and XML to implement user registration and verification functions, so that the website can effectively manage user information.
First, we need to create an XML file to store user information. We can create a file named "users.xml" in the root directory of the project. The structure of the file is as follows:
<users> <user> <id>1</id> <username>john123</username> <password>password123</password> </user> <user> <id>2</id> <username>jane456</username> <password>password456</password> </user> </users>
Next, we need to write PHP code to handle the logic of user registration and verification. First, we can create a file called "register.php" to handle user registration requests. The following is a code example:
<?php // 获取POST请求中的用户名和密码 $username = $_POST['username']; $password = $_POST['password']; // 加载用户数据 $users = simplexml_load_file('users.xml'); // 检查用户名是否已经存在 foreach ($users->user as $user) { if ($user->username == $username) { echo "用户名已存在,请选择其他用户名。"; exit; } } // 生成新的用户ID $newId = count($users->user) + 1; // 在XML中添加新用户 $newUser = $users->addChild('user'); $newUser->addChild('id', $newId); $newUser->addChild('username', $username); $newUser->addChild('password', $password); // 保存XML文件 $users->asXML('users.xml'); // 注册成功 echo "注册成功!"; ?>
The logic of the above code is as follows:
- Get the user name and password in the POST request;
- Load user data (users.xml file );
- Check whether the user name already exists;
- If the user name already exists, output the corresponding prompt information and end the script;
- Generate a new user ID;
- Add new user information in XML;
- Save the XML file;
- Output a prompt message indicating successful registration.
Next, we can create a file named "login.php" to handle user login requests. The following is a code example:
<?php // 获取POST请求中的用户名和密码 $username = $_POST['username']; $password = $_POST['password']; // 加载用户数据 $users = simplexml_load_file('users.xml'); // 验证用户名和密码是否匹配 foreach ($users->user as $user) { if ($user->username == $username && $user->password == $password) { echo "登录成功!"; exit; } } // 验证失败 echo "用户名或密码错误,请重试。"; ?>
The logic of the above code is as follows:
- Get the user name and password in the POST request;
- Load user data (users.xml file );
- Verify whether the username and password match;
- If the match is successful, output a prompt message indicating successful login and end the script;
- If the match fails, output the corresponding prompt information.
To sum up, by using PHP and XML, we can realize the functions of user registration and verification, and manage user information conveniently. Through reasonable data structures and simple code logic, we can effectively build a safe and reliable user system. Hope this article helps you!
The above is the detailed content of Use PHP and XML to implement user registration and verification functions. For more information, please follow other related articles on the PHP Chinese website!
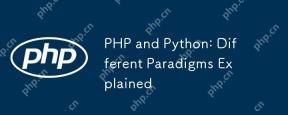
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
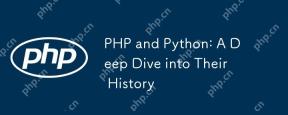
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
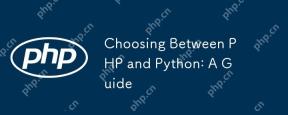
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
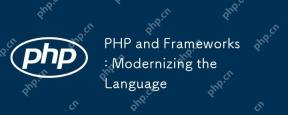
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
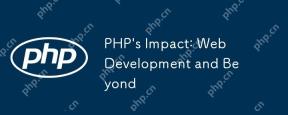
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
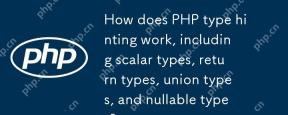
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
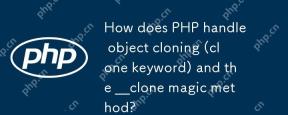
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
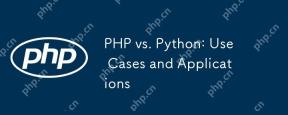
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
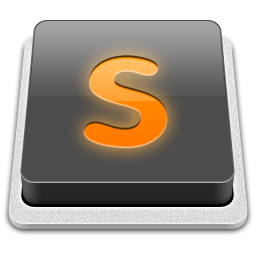
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor