


Building a distributed cache system using Java and Redis: how to speed up data access
Building a distributed cache system using Java and Redis: How to speed up data access
In modern software development, efficient data access is the key to ensuring system performance and user experience. As a common solution, the distributed cache system can significantly increase the reading speed of data and reduce the pressure on the database. This article will introduce how to use Java and Redis to build a simple but efficient distributed cache system, and provide code examples for reference and practice.
1. What is a distributed cache system?
The distributed cache system is a solution that stores data in memory. It caches commonly used data in the cache to provide more accurate data. Fast read speeds and lower latency. It can effectively reduce the pressure on the database and improve the overall performance of the system.
2. Why use Redis as a distributed cache system
Redis is an open source, high-performance in-memory database that supports multiple data structures. It has the following advantages, making it an ideal choice for building a distributed cache system:
- High performance: Redis uses a memory-based data storage method, which has very fast read and write speeds and can support high concurrency. access requirements.
- Multiple data structure support: Redis not only supports simple Key-Value structures, but also supports more complex data structures, such as List, Set, Hash, etc., making it more efficient when processing various types of data. flexible.
- Persistence support: Redis can persist data to disk to ensure data reliability after system failure or restart.
- Distributed support: Redis provides distributed features such as clustering and master-slave replication, which can horizontally expand the capacity and performance of the system.
3. Use Java to connect to Redis
To use Redis in Java, we can use Jedis as the connection tool between Java and Redis. The following is a simple code example showing how to connect to Redis and perform read and write operations:
import redis.clients.jedis.Jedis; public class RedisExample { public static void main(String[] args) { // 连接Redis服务器 Jedis jedis = new Jedis("localhost", 6379); // 向Redis中写入数据 jedis.set("key", "value"); // 从Redis中读取数据 String value = jedis.get("key"); System.out.println("value: " + value); // 关闭连接 jedis.close(); } }
4. Build a distributed cache system
When building a distributed cache system, we can use Redis Acts as a cache server and uses Java as the middle layer for applications to interact with Redis. The following is a simple example that shows how to use Java and Redis to build a simple distributed cache system:
import redis.clients.jedis.Jedis; public class DistributedCache { private Jedis jedis; public DistributedCache() { // 连接Redis服务器 jedis = new Jedis("localhost", 6379); } public void put(String key, String value) { // 缓存数据到Redis中 jedis.set(key, value); } public String get(String key) { // 从Redis中获取缓存数据 return jedis.get(key); } public void remove(String key) { // 从Redis中移除缓存数据 jedis.del(key); } public void close() { // 关闭连接 jedis.close(); } }
The above example code implements a simple distributed cache system, in which the put method is used to put data Put it into the cache, the get method is used to obtain cached data, and the remove method is used to remove cached data.
In practical applications, we can expand the functions of the distributed cache system as needed, such as adding cache expiration time, supporting distributed clusters, etc.
5. Summary
This article introduces how to use Java and Redis to build a simple but efficient distributed cache system. By using Redis as the cache server, the data reading speed can be significantly improved. , and reduce the pressure on the database. I hope this article can help you understand the principles and construction of a distributed cache system, and I also hope that the sample code can play a role in practice.
The above is the detailed content of Building a distributed cache system using Java and Redis: how to speed up data access. For more information, please follow other related articles on the PHP Chinese website!
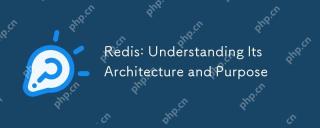
Redis is a memory data structure storage system, mainly used as a database, cache and message broker. Its core features include single-threaded model, I/O multiplexing, persistence mechanism, replication and clustering functions. Redis is commonly used in practical applications for caching, session storage, and message queues. It can significantly improve its performance by selecting the right data structure, using pipelines and transactions, and monitoring and tuning.
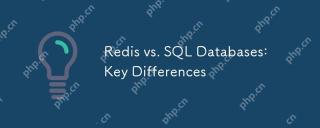
The main difference between Redis and SQL databases is that Redis is an in-memory database, suitable for high performance and flexibility requirements; SQL database is a relational database, suitable for complex queries and data consistency requirements. Specifically, 1) Redis provides high-speed data access and caching services, supports multiple data types, suitable for caching and real-time data processing; 2) SQL database manages data through a table structure, supports complex queries and transaction processing, and is suitable for scenarios such as e-commerce and financial systems that require data consistency.
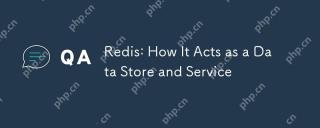
Redisactsasbothadatastoreandaservice.1)Asadatastore,itusesin-memorystorageforfastoperations,supportingvariousdatastructureslikekey-valuepairsandsortedsets.2)Asaservice,itprovidesfunctionalitieslikepub/submessagingandLuascriptingforcomplexoperationsan
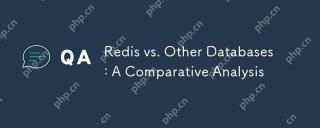
Compared with other databases, Redis has the following unique advantages: 1) extremely fast speed, and read and write operations are usually at the microsecond level; 2) supports rich data structures and operations; 3) flexible usage scenarios such as caches, counters and publish subscriptions. When choosing Redis or other databases, it depends on the specific needs and scenarios. Redis performs well in high-performance and low-latency applications.
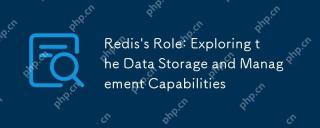
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
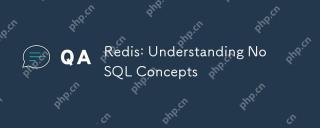
Redis is a NoSQL database suitable for efficient storage and access of large-scale data. 1.Redis is an open source memory data structure storage system that supports multiple data structures. 2. It provides extremely fast read and write speeds, suitable for caching, session management, etc. 3.Redis supports persistence and ensures data security through RDB and AOF. 4. Usage examples include basic key-value pair operations and advanced collection deduplication functions. 5. Common errors include connection problems, data type mismatch and memory overflow, so you need to pay attention to debugging. 6. Performance optimization suggestions include selecting the appropriate data structure and setting up memory elimination strategies.
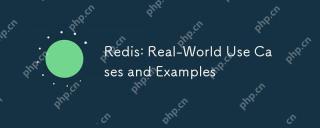
The applications of Redis in the real world include: 1. As a cache system, accelerate database query, 2. To store the session data of web applications, 3. To implement real-time rankings, 4. To simplify message delivery as a message queue. Redis's versatility and high performance make it shine in these scenarios.
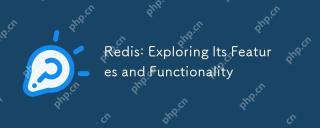
Redis stands out because of its high speed, versatility and rich data structure. 1) Redis supports data structures such as strings, lists, collections, hashs and ordered collections. 2) It stores data through memory and supports RDB and AOF persistence. 3) Starting from Redis 6.0, multi-threaded I/O operations have been introduced, which has improved performance in high concurrency scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment
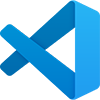
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor
