PHP and SQLite: How to handle images and multimedia files
PHP and SQLite: How to handle images and multimedia files
Introduction:
In modern websites and applications, processing images and multimedia files are very common tasks. PHP is a popular server-side scripting language, while SQLite is a lightweight embedded database engine. This article will explain how to use PHP and SQLite together to effectively process images and multimedia files.
1. Create a SQLite database
First, we need to create a SQLite database to store information related to images and multimedia files. Databases can be created using the SQLite command line tool or SQLite's PHP extension library.
The following is an example of using the SQLite command line tool to create a database:
$ sqlite3 media.db SQLite version 3.31.1 20... Enter ".help" for usage hints. sqlite> CREATE TABLE media ( id INTEGER PRIMARY KEY, filename TEXT NOT NULL, type TEXT NOT NULL, filepath TEXT NOT NULL ); sqlite> .quit
In the above example, we created a database named media.db
, And created a table named media
, which contains id
, filename
, type
and filepath
Four columns.
2. Upload images and multimedia files
Next, we need to write PHP code to process the uploaded images and multimedia files. First, we need an HTML form that users can use to upload files. The following is a simple form example:
<form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="file"> <input type="submit" value="Upload"> </form>
In the above form, we used enctype="multipart/form-data"
to support file upload. name="file"
defines the form field name for uploading files.
Next, we need to write a PHP script named upload.php
to process the uploaded file. The following is a simple script example:
<?php if(isset($_FILES['file'])) { $file = $_FILES['file']; $filename = $file['name']; $filetype = $file['type']; $filesize = $file['size']; $tmpname = $file['tmp_name']; $filepath = 'uploads/'. $filename; move_uploaded_file($tmpname, $filepath); // 插入文件信息到SQLite数据库 $db = new SQLite3('media.db'); $stmt = $db->prepare("INSERT INTO media (filename, type, filepath) VALUES (:filename, :type, :filepath)"); $stmt->bindValue(':filename', $filename, SQLITE3_TEXT); $stmt->bindValue(':type', $filetype, SQLITE3_TEXT); $stmt->bindValue(':filepath', $filepath, SQLITE3_TEXT); $stmt->execute(); echo "文件上传成功!"; } ?>
In the above code, we use $_FILES['file']
to access related information of the uploaded file, such as file name, type, size and temporary path. We then move the file to the specified directory on the server and insert the file information into the SQLite database.
3. Display images and multimedia files
Finally, we need to write code to retrieve file information from the SQLite database and display images and multimedia files on the web page. The following is a simple PHP script example:
<?php $db = new SQLite3('media.db'); $stmt = $db->prepare("SELECT * FROM media"); $result = $stmt->execute(); while($row = $result->fetchArray(SQLITE3_ASSOC)) { $filepath = $row['filepath']; $filetype = $row['type']; if($filetype === 'image/jpeg' || $filetype === 'image/png') { echo "<img src='$filepath' alt=''>"; } elseif($filetype === 'audio/mpeg') { echo "<audio controls><source src='$filepath' type='audio/mpeg'></audio>"; } elseif($filetype === 'video/mp4') { echo "<video controls><source src='$filepath' type='video/mp4'></video>"; } } ?>
In the above code, we retrieve all the file information from the SQLite database and based on the file type, use HTML's <img alt="PHP and SQLite: How to handle images and multimedia files" >
, <audio></audio>
or <video></video>
tags to display images and multimedia files.
Conclusion:
By using PHP and SQLite together, we can effectively process images and multimedia files. We can upload files to a specified directory on the server and store the file information in a SQLite database. We can then retrieve the file information from the database and display the images and multimedia files on the web page.
The above are examples of PHP and SQLite applications on how to process images and multimedia files. By using these technologies, we can easily implement the management and display functions of images and multimedia files in websites or applications.
The above is the detailed content of PHP and SQLite: How to handle images and multimedia files. For more information, please follow other related articles on the PHP Chinese website!
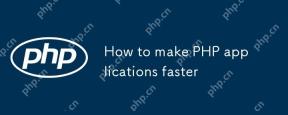
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
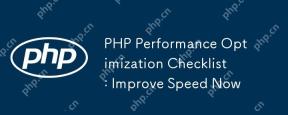
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
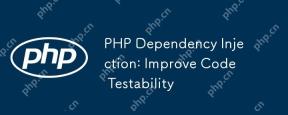
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
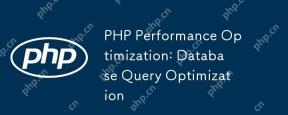
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
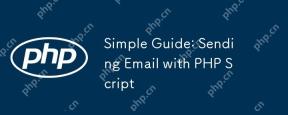
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
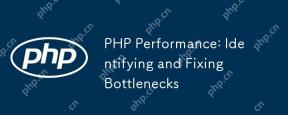
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
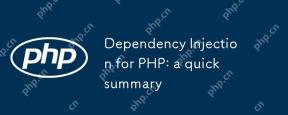
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
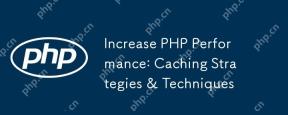
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
