


Tutorial: Java development steps to implement the geofence monitoring function of Amap
Tutorial: Steps to implement the geofence monitoring function of Amap with Java development
Geofence monitoring is an important function in modern positioning and navigation technology, which can help people monitor and alert specific areas. . In this tutorial, I will introduce how to use Java language to develop the geofence monitoring function of Amap. Below are the implementation steps and sample code.
Step 1: Apply for a Gaode Map developer account
First, we need to register on the official Gaode Map website (https://lbs.amap.com/) and apply for a developer account. After successful registration, we can obtain a developer key (Key) for accessing the API of Amap.
Step 2: Import the necessary dependencies
To use the Amap API in a Java project, we need to import the corresponding dependencies. In this tutorial, we will use AMAP’s Java SDK. You can add the following dependency in the Maven or Gradle configuration file:
<dependency> <groupId>com.amap.api</groupId> <artifactId>amap-java-sdk</artifactId> <version>1.4.0</version> </dependency>
Step 3: Create a geofence
In Amap, we can use the Polygon class to create a polygonal geofence. The following is a sample code:
// 创建地理围栏 Polygon polygon = new Polygon(); polygon.add(new LatLng(39.992806, 116.397238)); polygon.add(new LatLng(39.994439, 116.414496)); polygon.add(new LatLng(39.988628, 116.413819)); polygon.add(new LatLng(39.990234, 116.394844));
In this example, we create a quadrilateral geofence, using the LatLng
class to represent the latitude and longitude coordinates.
Step 4: Set up geofence monitoring
In Amap, we can use the GeoFenceClient
class to set up the geofence monitoring function. The following is a sample code:
// 创建地理围栏客户端 GeoFenceClient fenceClient = new GeoFenceClient(); fenceClient.setActivateAction(GeoFenceClient.GEOFENCE_IN | GeoFenceClient.GEOFENCE_OUT | GeoFenceClient.GEOFENCE_STAYED); // 设置地理围栏回调 fenceClient.createPendingIntent("com.example.geofence.ACTION_GEOFENCE"); // 设置监听器 fenceClient.setGeoFenceListener(new GeoFenceListener() { @Override public void onGeoFenceCreateFinished(List<GeoFence> geoFenceList, int errorCode, String errorMessage) { if (errorCode == GeoFence.ADDGEOFENCE_SUCCESS) { // 地理围栏添加成功 } } }); // 添加地理围栏 fenceClient.addGeoFence(polygon, "customId");
In this example, we create a geofence client GeoFenceClient
and set the trigger action type for monitoring. Then, we set up the callbacks and listeners for the geofence. Finally, we added the geofence we created earlier.
Step 5: Handle geofence trigger events
When the device enters, leaves, or stays within the geofence, we can handle the trigger event through the callback method. Here is a sample code:
// 创建触发事件广播接收器 BroadcastReceiver fenceReceiver = new BroadcastReceiver() { @Override public void onReceive(Context context, Intent intent) { // 处理地理围栏触发事件 String action = intent.getAction(); if (action.equals("com.example.geofence.ACTION_GEOFENCE")) { Bundle bundle = intent.getExtras(); List<GeoFence> geoFenceList = bundle.getParcelableArrayList("geoFenceList"); int status = bundle.getInt("status"); // 处理地理围栏触发事件 } } }; // 注册触发事件广播接收器 registerReceiver(fenceReceiver, new IntentFilter("com.example.geofence.ACTION_GEOFENCE"));
In this example, we create a broadcast receiver fenceReceiver
and handle the geofence trigger event. Then, we registered the broadcast receiver.
So far, we have completed the implementation steps of using Java to develop the geofence monitoring function of Amap. Hope this tutorial will be helpful to you. If you have any questions, please feel free to leave a message. Thanks!
The above is the detailed content of Tutorial: Java development steps to implement the geofence monitoring function of Amap. For more information, please follow other related articles on the PHP Chinese website!
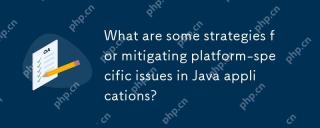
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
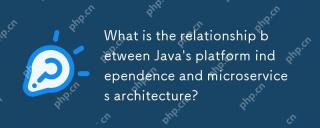
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
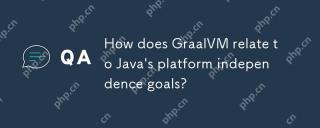
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.
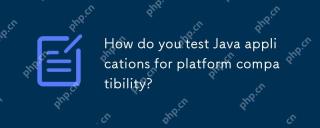
ToeffectivelytestJavaapplicationsforplatformcompatibility,followthesesteps:1)SetupautomatedtestingacrossmultipleplatformsusingCItoolslikeJenkinsorGitHubActions.2)ConductmanualtestingonrealhardwaretocatchissuesnotfoundinCIenvironments.3)Checkcross-pla
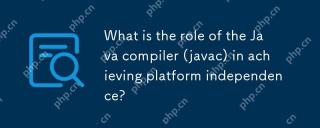
The Java compiler realizes Java's platform independence by converting source code into platform-independent bytecode, allowing Java programs to run on any operating system with JVM installed.
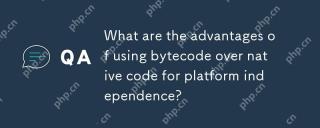
Bytecodeachievesplatformindependencebybeingexecutedbyavirtualmachine(VM),allowingcodetorunonanyplatformwiththeappropriateVM.Forexample,JavabytecodecanrunonanydevicewithaJVM,enabling"writeonce,runanywhere"functionality.Whilebytecodeoffersenh

Java cannot achieve 100% platform independence, but its platform independence is implemented through JVM and bytecode to ensure that the code runs on different platforms. Specific implementations include: 1. Compilation into bytecode; 2. Interpretation and execution of JVM; 3. Consistency of the standard library. However, JVM implementation differences, operating system and hardware differences, and compatibility of third-party libraries may affect its platform independence.
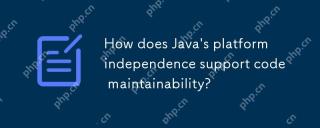
Java realizes platform independence through "write once, run everywhere" and improves code maintainability: 1. High code reuse and reduces duplicate development; 2. Low maintenance cost, only one modification is required; 3. High team collaboration efficiency is high, convenient for knowledge sharing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
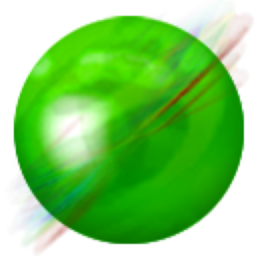
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
