PHP and OAuth: Implementing Google Sign-in Integration
OAuth is an open standard for authorization, which allows users to authorize access to their data on other websites through third-party applications. For developers, using OAuth can enable users to log in to third-party platforms, obtain user information, and access user data. In this article, we will focus on how to use OAuth to implement the integration of Google Login.
Google provides the OAuth 2.0 protocol to support users to access its services. To implement Google login integration, you first need to register a Google developer account and create a Google API project. Next, we will explain how to implement Google login integration with the following steps.
- Create an OAuth client ID
In the Google API console, create an OAuth client ID. In order to successfully create a client ID, you need to provide some necessary information, such as application name, redirect URL, etc. After creation, a client ID and client secret are generated, which will be used in subsequent steps. - Set Redirect URL
In the Google API console, make sure the correct redirect URL is set. The redirect URL is the URL address that the user will be redirected to after successful login so that we can obtain the authorization code and access token. - Get authorization code
To obtain the authorization code, you need to construct a Google OAuth authentication URL, which contains some parameters, such as client ID, redirect URL, application access scope, etc. Users will be redirected to this URL for login and authorization. Here is a sample code:
$authUrl = 'https://accounts.google.com/o/oauth2/auth'; $client_id = 'YOUR_CLIENT_ID'; $redirect_uri = 'YOUR_REDIRECT_URI'; $scope = 'email profile'; $response_type = 'code'; $url = $authUrl . '?' . http_build_query([ 'client_id' => $client_id, 'redirect_uri' => $redirect_uri, 'scope' => $scope, 'response_type' => $response_type, ]); header("Location: $url"); exit();
- Get access token
After the user successfully logs in and authorizes, he will be redirected to the redirect URL we provided with an authorization code. We need to use this authorization code to get the access token. Here is a sample code:
$tokenUrl = 'https://www.googleapis.com/oauth2/v4/token'; $client_id = 'YOUR_CLIENT_ID'; $client_secret = 'YOUR_CLIENT_SECRET'; $redirect_uri = 'YOUR_REDIRECT_URI'; $code = $_GET['code']; $data = [ 'code' => $code, 'client_id' => $client_id, 'client_secret' => $client_secret, 'redirect_uri' => $redirect_uri, 'grant_type' => 'authorization_code', ]; $options = [ 'http' => [ 'header' => "Content-type: application/x-www-form-urlencoded ", 'method' => 'POST', 'content' => http_build_query($data), ], ]; $context = stream_context_create($options); $response = file_get_contents($tokenUrl, false, $context); $token = json_decode($response, true); $access_token = $token['access_token'];
- Get user information
After obtaining the access token, we can use the token to get user information such as the user's email and name . The following is a sample code:
$userInfoUrl = 'https://www.googleapis.com/oauth2/v2/userinfo'; $options = [ 'http' => [ 'header' => "Authorization: Bearer $access_token ", ], ]; $context = stream_context_create($options); $response = file_get_contents($userInfoUrl, false, $context); $userInfo = json_decode($response, true); $email = $userInfo['email']; $name = $userInfo['name'];
Through the above five steps, we can integrate Google login using PHP and OAuth. You can obtain the authorization code after the user successfully logs in and use the authorization code to obtain the access token. With the help of access token we can get user information and use it in our application.
While this is just a basic example, it demonstrates how to integrate Google login using PHP and OAuth. OAuth also supports other platforms, such as Facebook, Twitter, etc. By using OAuth, we can easily implement login integration of various third-party platforms, and obtain user information and access to user data.
The above is the detailed content of PHP and OAuth: Implementing Google Login Integration. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
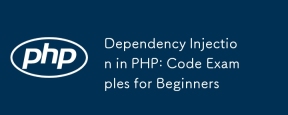
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
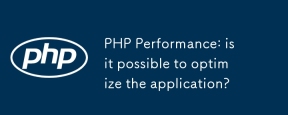
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
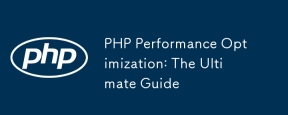
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
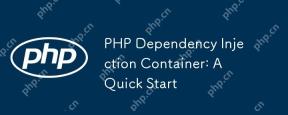
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
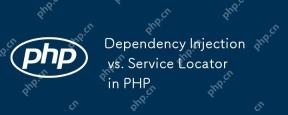
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
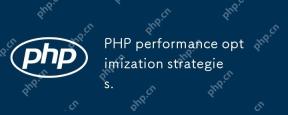
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
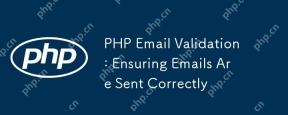
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
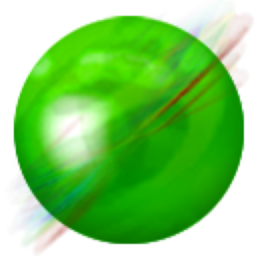
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
