


Interaction between Redis and Golang: How to achieve fast data storage and retrieval
Interaction between Redis and Golang: How to achieve fast data storage and retrieval
Introduction:
With the rapid development of the Internet, data storage and retrieval have become important needs in various application fields. In this context, Redis has become an important data storage middleware, and Golang has become the choice of more and more developers because of its efficient performance and simplicity of use. This article will introduce readers to how to interact with Golang through Redis to achieve fast data storage and retrieval.
1. Introduction to Redis
Redis is an in-memory database that supports different data structures, including strings, hash tables, lists, sets, ordered sets and bitmaps. Redis has fast read and write speeds and efficient memory management, making it a top choice for storage and caching solutions.
2. Golang’s Redis client library
In Golang, we can use a third-party Redis client library to interact with Redis. Among them, the more commonly used ones are go-redis, redigo, etc. This article uses go-redis as an example to introduce.
-
Install go-redis
Before using go-redis, we first need to install this library. It can be installed through the following command:go get github.com/go-redis/redis/v8
-
Connect to Redis
When using go-redis, we first need to establish a connection to Redis. This can be achieved through the following code:import ( "context" "github.com/go-redis/redis/v8" ) func main() { ctx := context.TODO() client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 设置密码 DB: 0, // 选择数据库 }) pong, err := client.Ping(ctx).Result() if err != nil { panic(err) } fmt.Println(pong) }
In the above code, we create a connection with Redis through the redis.NewClient function, and test whether the connection is normal through the client.Ping method.
- Storing and retrieving data
After establishing the connection, we can store and obtain data through the methods provided by go-redis. The following are examples of commonly used methods:
a. Store string:
err := client.Set(ctx, "key", "value", 0).Err() if err != nil { panic(err) }
b. Get string:
value, err := client.Get(ctx, "key").Result() if err == redis.Nil { fmt.Println("key does not exist") } else if err != nil { panic(err) } else { fmt.Println("key", value) }
c. Store hash table:
err := client.HSet(ctx, "hash", "field", "value").Err() if err != nil { panic(err) }
d. Get the hash table:
value, err := client.HGet(ctx, "hash", "field").Result() if err == redis.Nil { fmt.Println("field does not exist") } else if err != nil { panic(err) } else { fmt.Println("field", value) }
3. Usage example
The following is a sample code that uses Golang and Redis to implement caching:
import ( "context" "fmt" "time" "github.com/go-redis/redis/v8" ) func main() { ctx := context.TODO() client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 设置密码 DB: 0, // 选择数据库 }) // 查询缓存 articleID := "123" cacheKey := fmt.Sprintf("article:%s", articleID) cacheValue, err := client.Get(ctx, cacheKey).Result() if err == redis.Nil { // 缓存不存在,从数据库中读取数据 article, err := getArticleFromDB(articleID) if err != nil { panic(err) } // 将数据存入缓存 err = client.Set(ctx, cacheKey, article, 10*time.Minute).Err() if err != nil { panic(err) } // 使用从数据库中读取的数据 fmt.Println("Article:", article) } else if err != nil { panic(err) } else { // 使用缓存数据 fmt.Println("Article:", cacheValue) } } func getArticleFromDB(articleID string) (string, error) { // 模拟从数据库中读取数据 // 这里可以是实际数据库的查询操作 return "This is the article content.", nil }
In the above code , through a simple example, shows how to use Golang and Redis to store and obtain data. First, we query whether the cached data exists. If it does not exist, the data is read from the database and stored in the cache. If it exists, the data in the cache is used directly. In this way, we can achieve fast data storage and retrieval.
Conclusion:
This article introduces how to implement the interaction between Golang and Redis through the go-redis library to achieve fast data storage and retrieval. Readers can modify and extend the sample code according to their actual needs to meet their own project needs. By rationally utilizing the characteristics of Redis and Golang, we can improve the efficiency of data processing and improve application performance.
Reference:
- go-redis official documentation: https://pkg.go.dev/github.com/go-redis/redis/v8
- Redis official documentation: https://redis.io/documentation
The above is the detailed content of Interaction between Redis and Golang: How to achieve fast data storage and retrieval. For more information, please follow other related articles on the PHP Chinese website!
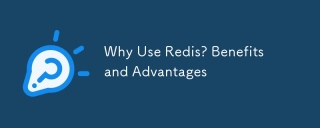
Redis is a powerful database solution because it provides fast performance, rich data structures, high availability and scalability, persistence capabilities, and a wide range of ecosystem support. 1) Extremely fast performance: Redis's data is stored in memory and has extremely fast read and write speeds, suitable for high concurrency and low latency applications. 2) Rich data structure: supports multiple data types, such as lists, collections, etc., which are suitable for a variety of scenarios. 3) High availability and scalability: supports master-slave replication and cluster mode to achieve high availability and horizontal scalability. 4) Persistence and data security: Data persistence is achieved through RDB and AOF to ensure data integrity and reliability. 5) Wide ecosystem and community support: with a huge ecosystem and active community,
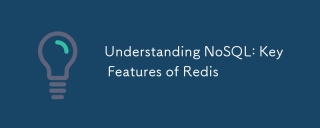
Key features of Redis include speed, flexibility and rich data structure support. 1) Speed: Redis is an in-memory database, and read and write operations are almost instantaneous, suitable for cache and session management. 2) Flexibility: Supports multiple data structures, such as strings, lists, collections, etc., which are suitable for complex data processing. 3) Data structure support: provides strings, lists, collections, hash tables, etc., which are suitable for different business needs.
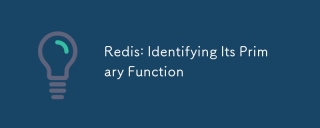
The core function of Redis is a high-performance in-memory data storage and processing system. 1) High-speed data access: Redis stores data in memory and provides microsecond-level read and write speed. 2) Rich data structure: supports strings, lists, collections, etc., and adapts to a variety of application scenarios. 3) Persistence: Persist data to disk through RDB and AOF. 4) Publish subscription: Can be used in message queues or real-time communication systems.

Redis supports a variety of data structures, including: 1. String, suitable for storing single-value data; 2. List, suitable for queues and stacks; 3. Set, used for storing non-duplicate data; 4. Ordered Set, suitable for ranking lists and priority queues; 5. Hash table, suitable for storing object or structured data.
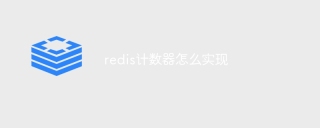
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.

Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
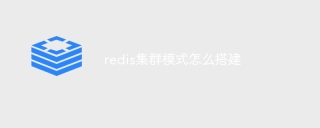
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make

To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
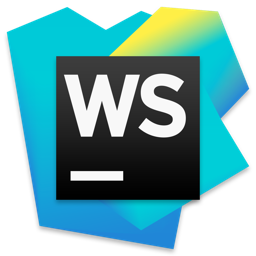
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.