


Quick Start: Use Go language functions to implement simple data cleaning functions
Quick Start: Use Go language functions to implement simple data cleaning functions
Introduction:
Data cleaning is one of the important steps in data processing. It can help us filter out the original data that meets the requirements. of data, remove non-compliant data, and ensure data accuracy and availability. As a simple and efficient programming language, Go language provides a rich function library and powerful grammatical features, which can help us achieve various data processing needs. This article will use Go language functions to implement a simple data cleaning function and give relevant code examples to help readers get started quickly.
Text:
- Requirements Analysis
Before performing data cleaning, we first need to clarify the requirements for data cleaning. For example, we have a dataset that contains names, ages, and genders, and we need to filter out the data for males who are over 18 years old. Based on this requirement, we can start writing code. - Writing data cleaning function
First, we need to create a function to perform data cleaning operations. The following is a sample function to achieve the above requirements:
func cleanData(data []map[string]interface{}) []map[string]interface{} { var cleanedData []map[string]interface{} for _, d := range data { age := d["age"].(int) gender := d["gender"].(string) if age >= 18 && gender == "male" { cleanedData = append(cleanedData, d) } } return cleanedData }
In this function, we traverse the incoming data
parameters and convert the corresponding fields through assertions for the corresponding type. Then, we filter and process the data according to requirements, add qualified data to the cleanedData
array, and finally return cleanedData
.
- Calling the data cleaning function
Next, we need to create a data set to test our data cleaning function. The following is a sample data set:
data := []map[string]interface{}{ {"name": "Alice", "age": 20, "gender": "female"}, {"name": "Bob", "age": 25, "gender": "male"}, {"name": "Charlie", "age": 16, "gender": "male"}, {"name": "Dave", "age": 30, "gender": "male"}, }
We can call the cleanData
function to clean the data and print the cleaned results:
cleanedData := cleanData(data) for _, d := range cleanedData { fmt.Println(d) }
Run the above The code will output the data of males over 18 years old:
map[name:Bob age:25 gender:male] map[name:Dave age:30 gender:male]
- Extensibility of data cleaning
In practical applications, we may face more complex data cleaning requirements. In order to improve the reusability and scalability of the code, we can split the data cleaning functions, and each function is responsible for a specific data processing task. For example, we can encapsulate the logic of age screening and gender screening into two functions respectively:
func filterByAge(age int, data []map[string]interface{}) []map[string]interface{} { var filteredData []map[string]interface{} for _, d := range data { dAge := d["age"].(int) if dAge >= age { filteredData = append(filteredData, d) } } return filteredData } func filterByGender(gender string, data []map[string]interface{}) []map[string]interface{} { var filteredData []map[string]interface{} for _, d := range data { dGender := d["gender"].(string) if dGender == gender { filteredData = append(filteredData, d) } } return filteredData }
In this way, we can combine and call these functions according to specific needs to build a program that meets the requirements. data set.
Summary:
This article implements a simple data cleaning function by using Go language functions, with detailed introductions and examples from requirement analysis to code writing. I hope this article can help readers quickly get started with data cleaning and understand how to use Go language functions to process data. In practical applications, readers can expand and optimize according to specific needs to achieve more complex data cleaning functions.
The above is the detailed content of Quick Start: Use Go language functions to implement simple data cleaning functions. For more information, please follow other related articles on the PHP Chinese website!
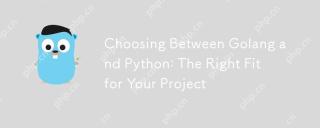
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
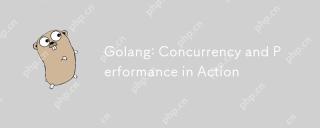
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
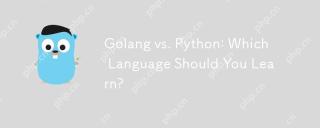
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
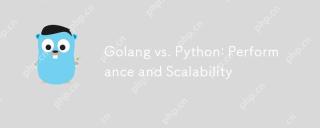
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
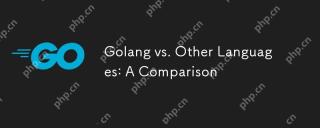
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
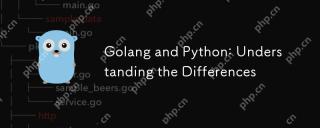
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
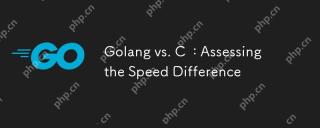
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
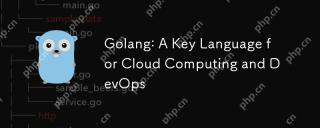
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
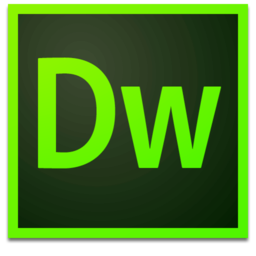
Dreamweaver Mac version
Visual web development tools
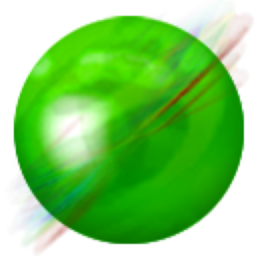
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
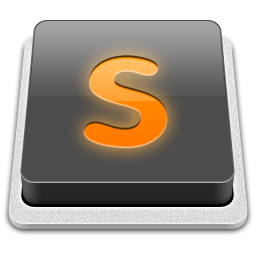
SublimeText3 Mac version
God-level code editing software (SublimeText3)