How to implement data binding function in SwiftUI using MySQL
How to use MySQL to implement data binding function in SwiftUI
In SwiftUI development, automatic updating of interface and data can be achieved through data binding, improving user experience. As a popular relational database management system, MySQL can store and manage large amounts of data. This article will introduce how to use MySQL to implement data binding function in SwiftUI. We will utilize Swift's third-party library MySQLConnector, which provides functionality to connect to and query MySQL databases.
First, we need to create a new SwiftUI project in Xcode. Then, use the following command in the terminal to install the MySQLConnector library:
$ swift package init --type library
Next, add the dependency of MySQLConnector in the dependencies section of the Package.swift file:
dependencies: [ .package(url: "https://github.com/PerfectlySoft/MySQLConnector.git", from: "3.0.0"), ],
Then, run the following command to generate Xcode Project File:
$ swift package generate-xcodeproj
Open the generated Xcode project and add the sample code for a MySQL connection and query in your SwiftUI view.
First, we need to create a MySQL connection object:
import MySQLConnector let connection = MySQL() func connectToDatabase() { let host = "localhost" let user = "root" let password = "your_password" let database = "your_database" guard connection.connect(host: host, user: user, password: password, db: database) else { let error = connection.error() print("Failed to connect to database: (error)") return } print("Connected to MySQL database") }
Next, we can execute the query and bind the results to the SwiftUI view. We can create a model object to store the data retrieved from the database:
struct Item: Identifiable { let id: Int let name: String } class ItemListModel: ObservableObject { @Published var items = [Item]() func fetchItems() { guard connection.query(statement: "SELECT * FROM items") else { let error = connection.error() print("Failed to fetch items from database: (error)") return } guard let results = connection.storeResults() else { print("Failed to fetch items from database") return } items.removeAll() while let row = results.next() { guard let id = row[0] as? Int, let name = row[1] as? String else { continue } let item = Item(id: id, name: name) items.append(item) } } }
We can then use data binding in a view in SwiftUI to display the data retrieved from the database:
struct ContentView: View { @ObservedObject var itemListModel = ItemListModel() var body: some View { VStack { Button(action: { self.itemListModel.fetchItems() }) { Text("Fetch Items") } List(itemListModel.items) { item in Text(item.name) } } } }
Finally, connect to the database and display the view in the SwiftUI program entrance App.swift:
@main struct MyApp: App { var body: some Scene { WindowGroup { ContentView() .onAppear(perform: { connectToDatabase() }) } } }
Now, we have completed the function of using MySQL to implement data binding in SwiftUI. When the "Fetch Items" button is clicked, the data in the view will be queried from the database and updated. Automatic binding of data will ensure timely updating of the interface and display of the latest data.
In this way, we can easily implement data binding functions in SwiftUI and interact with the MySQL database. This provides us with a powerful tool to build feature-rich applications and improve the user's interactive experience.
I hope this article can help you understand how to use MySQL to implement data binding functions in SwiftUI, and be able to apply these techniques in your projects. Happy programming!
The above is the detailed content of How to implement data binding function in SwiftUI using MySQL. For more information, please follow other related articles on the PHP Chinese website!
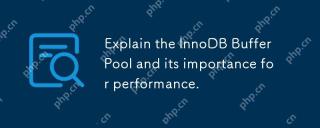
InnoDBBufferPool reduces disk I/O by caching data and indexing pages, improving database performance. Its working principle includes: 1. Data reading: Read data from BufferPool; 2. Data writing: After modifying the data, write to BufferPool and refresh it to disk regularly; 3. Cache management: Use the LRU algorithm to manage cache pages; 4. Reading mechanism: Load adjacent data pages in advance. By sizing the BufferPool and using multiple instances, database performance can be optimized.
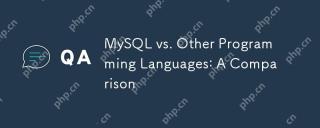
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
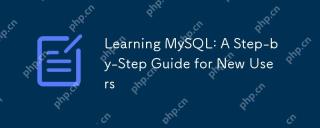
MySQL is worth learning because it is a powerful open source database management system suitable for data storage, management and analysis. 1) MySQL is a relational database that uses SQL to operate data and is suitable for structured data management. 2) The SQL language is the key to interacting with MySQL and supports CRUD operations. 3) The working principle of MySQL includes client/server architecture, storage engine and query optimizer. 4) Basic usage includes creating databases and tables, and advanced usage involves joining tables using JOIN. 5) Common errors include syntax errors and permission issues, and debugging skills include checking syntax and using EXPLAIN commands. 6) Performance optimization involves the use of indexes, optimization of SQL statements and regular maintenance of databases.
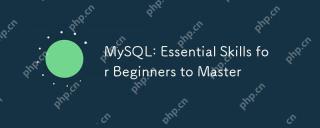
MySQL is suitable for beginners to learn database skills. 1. Install MySQL server and client tools. 2. Understand basic SQL queries, such as SELECT. 3. Master data operations: create tables, insert, update, and delete data. 4. Learn advanced skills: subquery and window functions. 5. Debugging and optimization: Check syntax, use indexes, avoid SELECT*, and use LIMIT.
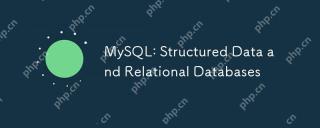
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
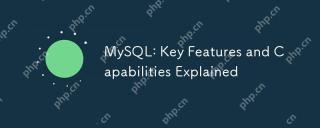
MySQL is an open source relational database management system that is widely used in Web development. Its key features include: 1. Supports multiple storage engines, such as InnoDB and MyISAM, suitable for different scenarios; 2. Provides master-slave replication functions to facilitate load balancing and data backup; 3. Improve query efficiency through query optimization and index use.
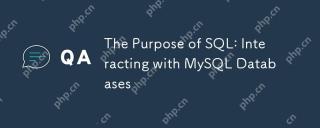
SQL is used to interact with MySQL database to realize data addition, deletion, modification, inspection and database design. 1) SQL performs data operations through SELECT, INSERT, UPDATE, DELETE statements; 2) Use CREATE, ALTER, DROP statements for database design and management; 3) Complex queries and data analysis are implemented through SQL to improve business decision-making efficiency.
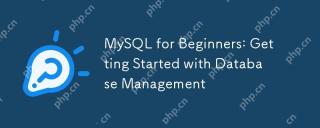
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
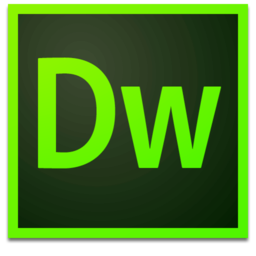
Dreamweaver Mac version
Visual web development tools
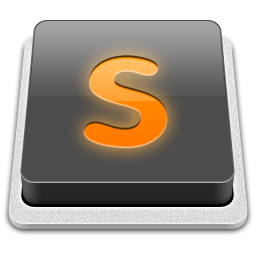
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
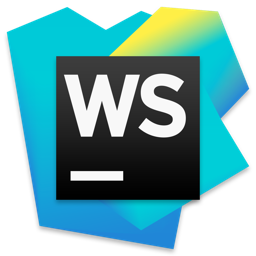
WebStorm Mac version
Useful JavaScript development tools