


How to use hashlib module for hash algorithm calculation in Python 3.x
How to use the hashlib module for hash algorithm calculation in Python 3.x
Overview:
The hash algorithm is an algorithm that maps data of any length into a fixed-length unique identifier. In Python, we can use the hashlib module to perform hash algorithm calculations. This article will introduce how to use the hashlib module to perform hash algorithm calculations in Python 3.x and provide corresponding code examples.
hashlib module introduction:
The hashlib module is a module in the Python standard library, which provides the implementation of various hash algorithms. Using the hashlib module, you can easily perform various common hash algorithm calculations, such as MD5, SHA1, etc.
Steps to use hashlib for hash algorithm calculation:
-
Import hashlib module:
First, you need to import the hashlib module in order to use the hash algorithm function in it .import hashlib
-
Create hash object:
Select the appropriate hash algorithm type as needed, and then use the functions in the hashlib module to create the hash object.hash_object = hashlib.new('hash_algorithm')
Among them, 'hash_algorithm' is the name of the hash algorithm, common ones include md5, sha1, sha256, etc.
-
Update hash object:
Continuously update the data to be hashed to generate the correct hash value.hash_object.update(data)
Among them, data is the data to calculate the hash value, which can be a string, byte string, etc.
-
Calculate the hash value:
Use the hexdigest() method of the hash object to calculate the hash value.hash_value = hash_object.hexdigest()
At this time, hash_value is the calculated hash value, which is a string.
Specific example:
Next, we take calculating the MD5 hash value of a string as an example to demonstrate how to use the hashlib module to calculate the hash algorithm.
import hashlib def calculate_md5(string): # 创建hash对象 hash_object = hashlib.new('md5') # 更新hash对象 hash_object.update(string.encode('utf-8')) # 计算哈希值 hash_value = hash_object.hexdigest() # 返回结果 return hash_value if __name__ == "__main__": string = "Hello, hashlib!" md5_hash_value = calculate_md5(string) print("MD5 hash value of", string, "is:", md5_hash_value)
In the above example, we first imported the hashlib module and defined a function named calculate_md5(). In the calculate_md5() function, we first create an MD5 hash object using hashlib.new('md5'), then use the update() method to update the hash object, calculate the MD5 hash value of the string, and use hexdigest () method obtains the string representation of the hash value. Finally, call the calculate_md5() function in the main program and print out the calculated MD5 hash value.
Conclusion:
The hashlib module provides a convenient interface that allows us to easily perform hash algorithm calculations in Python 3.x. By using the hashlib module, we can calculate various common hash algorithms and obtain the corresponding hash values. Whether it is calculating the hash value of a file or hashing a password, the hashlib module provides us with a very convenient method.
To summarize, this article introduces the steps of using the hashlib module to perform hash algorithm calculations in Python 3.x, and gives specific code examples. I hope this article can help readers better understand how to use the hashlib module and flexibly use hash algorithms in actual development.
The above is the detailed content of How to use hashlib module for hash algorithm calculation in Python 3.x. For more information, please follow other related articles on the PHP Chinese website!
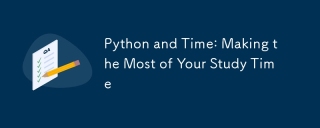
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
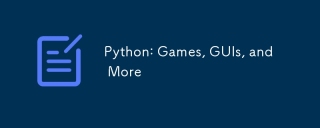
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
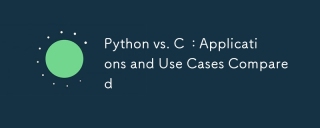
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
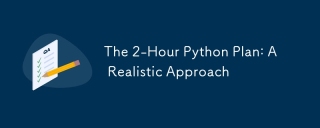
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
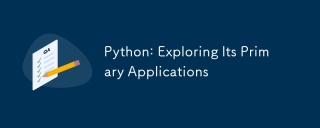
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
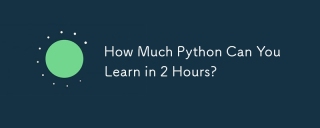
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
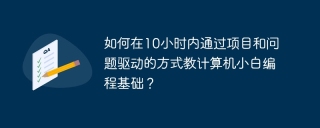
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
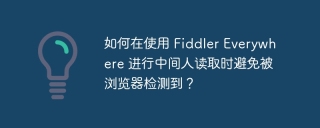
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),