


Learn the type conversion function in Go language and implement the function of converting string to integer
Learn the type conversion function in Go language and implement the function of converting string to integer
In Go language, type conversion is the process of converting one data type to another data type. Go language provides type conversion functions to achieve conversion between different types.
This article will learn the type conversion function in Go and the sample code to realize the function of converting string to integer.
- Use the Atoi() function of the strconv package to convert strings to integers
The strconv package of the Go language provides many functions for type conversion. Among them, the Atoi() function is used to convert strings to integers. The following is the declaration and usage example of the Atoi() function:
func Atoi(s string) (int, error)
The parameter of the Atoi() function is a string s, and the return value is the converted integer and an error. If the conversion is successful, the converted integer value and nil are returned; if the conversion fails, 0 and an error are returned.
Sample code:
package main import ( "fmt" "strconv" ) func main() { str := "12345" num, err := strconv.Atoi(str) if err != nil { fmt.Println("字符串转整数失败:", err) return } fmt.Println("转换后的整数:", num) }
Run the above code, the output result will be:
转换后的整数:12345
- Custom function to convert string to integer
In addition to using the Atoi() function of the strconv package, we can also customize functions to convert strings to integers.
Sample code:
package main import ( "fmt" ) func StringToInt(str string) (int, error) { var num int for i := 0; i < len(str); i++ { if str[i] >= '0' && str[i] <= '9' { num = num*10 + int(str[i]-'0') } else { return 0, fmt.Errorf("字符串中包含非数字字符") } } return num, nil } func main() { str := "12345" num, err := StringToInt(str) if err != nil { fmt.Println("字符串转整数失败:", err) return } fmt.Println("转换后的整数:", num) }
Run the above code, the output result is also:
转换后的整数:12345
The above is to learn the type conversion function in Go language and realize the function of converting string to integer methods and sample code. Hope this article is helpful to you!
The above is the detailed content of Learn the type conversion function in Go language and implement the function of converting string to integer. For more information, please follow other related articles on the PHP Chinese website!
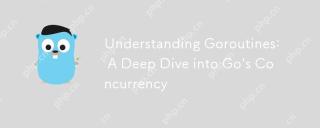
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
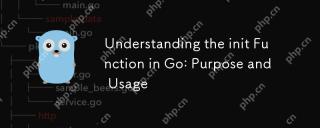
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
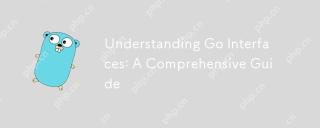
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
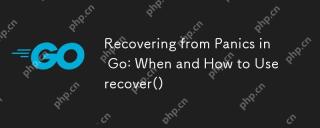
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
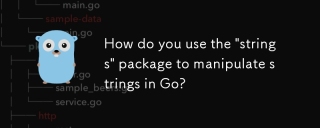
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
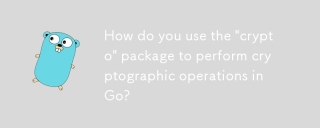
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
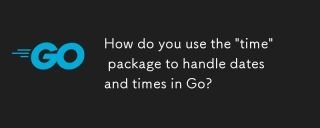
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
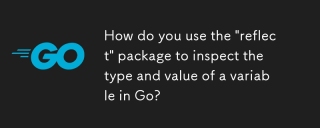
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
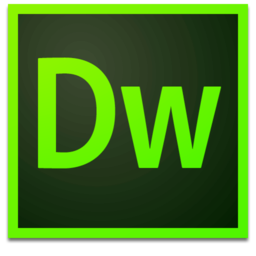
Dreamweaver Mac version
Visual web development tools
