


How to optimize database index through thinkorm to improve query efficiency
How to optimize database index through thinkorm to improve query efficiency
Introduction:
When conducting large-scale data queries, the optimization of database index is the key to improving query efficiency. This article will introduce how to optimize database indexes through the thinkorm framework to improve query efficiency. At the same time, we will provide some code examples to demonstrate how to use indexes in thinkorm.
- Understanding database indexes:
A database index is a data structure used to quickly find specific data in a database. It is similar to the table of contents of a book, allowing you to quickly locate the required data. Indexes greatly increase the query speed of the database, but also increase storage and maintenance costs. - Select the appropriate field to create an index:
When using thinkorm to create a table, we can create an index by setting the index property of the field. Usually, selecting fields that are frequently used in queries to create indexes can improve query efficiency. For example, for the users table, you can choose to index based on the user ID.
use thinkmigrationdbColumn; use thinkmigrationdbTable; class CreateUsersTable extends Migration { public function up() { $table = $this->table('users'); $table->addColumn('id', 'integer', ['signed' => false]) ->addColumn('name', 'string', ['limit' => 50]) ->addColumn('email', 'string', ['limit' => 100, 'null' => true]) ->addIndex(['id'], ['unique' => true]) ->create(); } }
In the above example, we created a unique index for the ID field of the user table, so that the ID can be used to quickly locate the specified user.
- Multi-field index:
In addition to single-field indexes, we can also create multi-field indexes to improve the efficiency of complex queries. For example, in the user table, we may need to query based on name and email address.
use thinkmigrationdbColumn; use thinkmigrationdbTable; class CreateUsersTable extends Migration { public function up() { $table = $this->table('users'); $table->addColumn('name', 'string', ['limit' => 50]) ->addColumn('email', 'string', ['limit' => 100, 'null' => true]) ->addIndex(['name', 'email']) ->create(); } }
In the above example, we created a multi-field index for the name and email fields of the user table, so that we can use it to perform joint queries on name and email.
- Use index for query:
When using thinkorm for query, we can optimize the query efficiency by specifying the index. thinkorm provides corresponding methods to achieve this. For example, suppose we want to query user information based on user ID:
$User = new User(); $user = $User->where('id', 'eq', 1)->useIndex(['id'])->find();
In the above example, we specify the use of the ID index for querying by using the useIndex()
method, so that Improve query efficiency.
- Update and maintain index:
In the database, data is frequently updated and maintained, and the index also needs to be updated and maintained. In the thinkorm framework, we can use migration files to manage the structure and indexes of database tables. Using migration files makes it easier to update and maintain indexes. Here is an example of updating an index:
use thinkmigrationdbColumn; use thinkmigrationdbTable; class UpdateUsersTable extends Migration { public function up() { $table = $this->table('users'); $table->addIndex('email') ->update(); } public function down() { $table = $this->table('users'); $table->removeIndex('email') ->update(); } }
In the above example, we added a new index via the addIndex()
method and removed it via removeIndex()
Method deletes existing index.
Conclusion:
By rationally selecting and using indexes, we can significantly improve the query efficiency of the database. Through the thinkorm framework, we can easily create, use and update indexes. I hope this article can help you optimize database indexes and improve query efficiency. If you are using the thinkorm framework, you are welcome to try the above sample code to optimize your database index.
The above is the detailed content of How to optimize database index through thinkorm to improve query efficiency. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
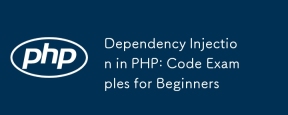
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
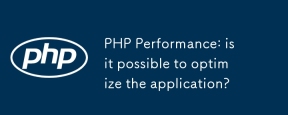
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
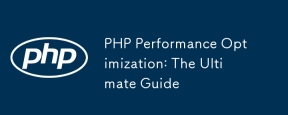
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
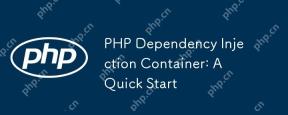
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
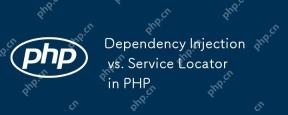
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
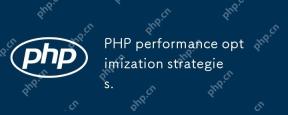
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
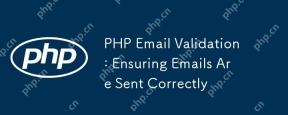
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
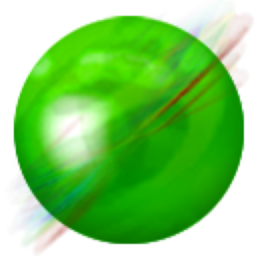
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
