


How to use the read() function to read file contents in Python 2.x
How to use the read() function to read the contents of a file in Python 2.x
In early versions of Python 2.x, the read() function can be used to easily read the contents of a file. The read() function is a built-in method of Python, which is used to read characters of a specified length from a file. The following will introduce how to use the read() function and some precautions.
First, we need to open a file. Files can be opened using the open() function, as shown below:
f = open("file.txt", "r")
The above code will open a file named file.txt and set it to read-only mode ("r"). You can adjust the mode as needed.
Then, we can use the read() function to read the contents of the file. The read() function can accept an optional length parameter to specify the number of characters to read. If the length parameter is not specified, the entire contents of the file will be read by default.
The following is an example of using the read() function to read the content of a file:
f = open("file.txt", "r") content = f.read() print(content) f.close()
The above code will open the file.txt file and assign its content to the variable content. Finally, use the print statement to print out the contents of the file. Please note that after reading the file contents, we need to close the file using the close() function.
In addition, the read() function also has an optional parameter size, which is used to specify the number of characters to be read. Here is an example that demonstrates how to read the first 10 characters of a file:
f = open("file.txt", "r") content = f.read(10) print(content) f.close()
The above code will read the first 10 characters of the file and print them out.
It should be noted that when using the read() function to read the file content, the file pointer will move backward. That is, after reading the file contents, the file pointer will point to the end of the file. If you want to read the file contents again or perform other operations on the file, you need to reopen the file.
In addition, there are some other functions that can be used with the read() function. For example, the readline() function can be used to read the contents of a file line, and the readlines() function can be used to read the contents of a file line by line into a list.
When using the read() function to read the file content, you need to pay attention to the encoding format of the file. If the file encoding format is UTF-8, you can directly use the read() function to read. If the file encoding format is not UTF-8, garbled characters may occur. In this case, it can be solved by specifying the encoding format of the file, as shown below:
f = open("file.txt", "r", encoding="gbk") content = f.read() print(content) f.close()
The above code will use the GBK encoding format to read the contents of the file.txt file.
To summarize, using the read() function in Python 2.x can easily read the contents of a file. We can read the entire contents of the file by specifying the number of characters or not. It should be noted that after reading the file contents, remember to close the file to release system resources. In addition, it can also be used with other functions to implement more file reading operations. Finally, pay attention to the encoding format of the file to avoid garbled characters.
The above is the detailed content of How to use the read() function to read file contents in Python 2.x. For more information, please follow other related articles on the PHP Chinese website!
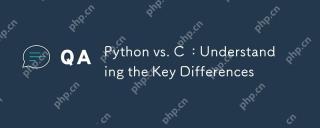
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
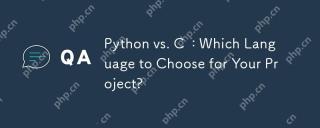
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
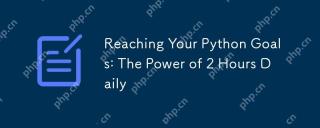
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
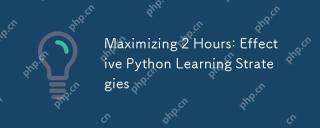
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
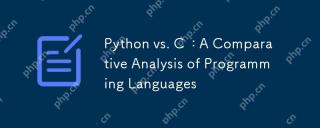
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
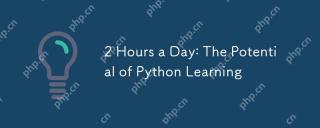
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
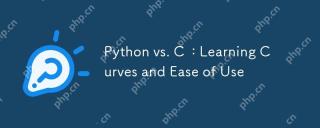
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
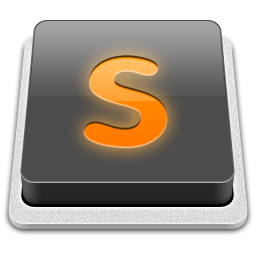
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor