


Learn database functions in Go language and implement read and write operations in Redis cluster
Learn the database functions in Go language and implement read and write operations in Redis cluster
Introduction:
Database is an indispensable part of today's Internet applications, and Go language is a simple and efficient way to develop programming language and also has good database operation capabilities. This article will introduce how to use database functions in Go language and implement read and write operations in Redis cluster.
1. Database functions in Go language
The operations on the database in Go language are mainly implemented through the database/sql package. This package provides basic database operation functions, including connecting to the database, executing SQL statements, processing result sets, etc.
-
Connecting to the database
In the Go language, we can connect to the database through the Open function in the database/sql package. This function accepts two parameters: the driver name of the database and the connection information of the database, for example:import "database/sql" import _ "github.com/go-sql-driver/mysql" db, err := sql.Open("mysql", "root:password@tcp(127.0.0.1:3306)/database") if err != nil { log.Fatal(err) } defer db.Close()
In the above code, we use the mysql driver to connect to the local MySQL database. The user name is root, the password is password, and the database name for database.
-
Execute SQL statements
After connecting to the database, we can execute SQL statements through functions such as db.Exec or db.Query. The Exec function is used to execute SQL statements that do not return a result set, such as insert, update, delete, etc.; the Query function is used to execute SQL statements that return a result set, such as query operations. An example is as follows:stmt, err := db.Prepare("INSERT INTO users(email, password) VALUES(?, ?)") if err != nil { log.Fatal(err) } defer stmt.Close() result, err := stmt.Exec("test@example.com", "password123") if err != nil { log.Fatal(err) } lastInsertID, err := result.LastInsertId() if err != nil { log.Fatal(err) } fmt.Println(lastInsertID)
In the above code, we use the Prepare function to precompile an insert statement and bind the parameters to?, and then execute the insert statement through the Exec function, and the return result is stored in the result variable . The auto-increment ID of the inserted data can be obtained through result.LastInsertId().
-
Processing result sets
When executing a SQL statement that returns a result set, we can use the Rows function to obtain the query results. Then each piece of data is processed by looping through the result set. The example is as follows:rows, err := db.Query("SELECT id, email FROM users") if err != nil { log.Fatal(err) } defer rows.Close() for rows.Next() { var id int var email string err := rows.Scan(&id, &email) if err != nil { log.Fatal(err) } fmt.Println(id, email) }
In the above code, we executed a query statement through the db.Query function and stored the result in the rows variable. Then traverse each piece of data through rows.Next, and save the data to the corresponding variable through rows.Scan.
2. Implement read and write operations in Redis cluster
Redis is a high-performance key-value storage database. Redis can be operated in Go language through the go-redis/redis package . Next we will use this package to implement read and write operations in the Redis cluster.
-
Connecting to the Redis cluster
In the Go language, we can connect to the Redis cluster through the redis.NewClusterClient function. An example is as follows:import "github.com/go-redis/redis/v8" cluster := redis.NewClusterClient(&redis.ClusterOptions{ Addrs: []string{"node1:6379", "node2:6379", "node3:6379"}, }) defer cluster.Close()
In the above code, we created a redis.ClusterClient object and specified the node address of the Redis cluster through the ClusterOptions parameter.
-
Write data to Redis cluster
After connecting to the Redis cluster, we can use the Set function to write data to the cluster. An example is as follows:err := cluster.Set(context.Background(), "key", "value", 0).Err() if err != nil { log.Fatal(err) }
In the above code, we write a piece of key-value data to the Redis cluster through the cluster.Set function. The expiration time is 0, which means it will never expire.
-
Reading data from the Redis cluster
After connecting to the Redis cluster, we can use the Get function to read data from the cluster. An example is as follows:val, err := cluster.Get(context.Background(), "key").Result() if err != nil { log.Fatal(err) } fmt.Println(val)
In the above code, we read the value corresponding to the key from the Redis cluster through the cluster.Get function and print it out.
Summary:
This article introduces the database functions in learning Go language and implements the read and write operations of Redis cluster. Through database functions, we can easily connect to the database, execute SQL statements, and process result sets. Through the go-redis/redis package, we can easily interact with the Redis cluster to achieve efficient storage and read operations. I hope this article can help readers better understand the database functions in Go language and apply them to actual projects.
The above is the detailed content of Learn database functions in Go language and implement read and write operations in Redis cluster. For more information, please follow other related articles on the PHP Chinese website!
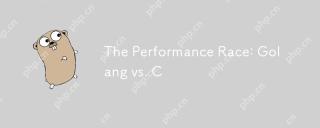
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
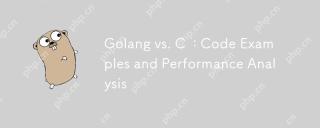
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
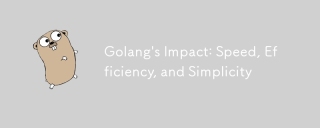
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
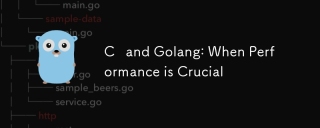
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
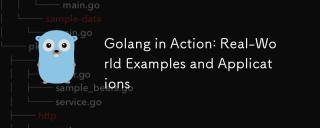
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
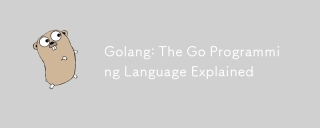
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
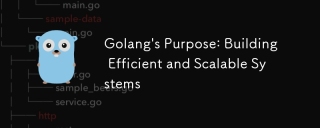
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
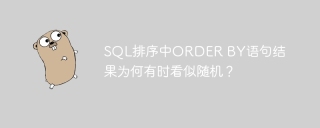
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
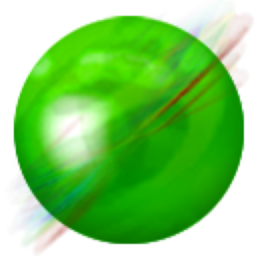
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
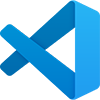
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft