


How to use network programming functions in Go language to implement HTTP server downloading files?
How to use network programming functions in Go language to implement HTTP server download files?
Network programming plays an important role in modern software development. Using the Go language, we can easily implement an HTTP server so that it can provide file download functions. This article will introduce how to use the network programming functions in the Go language to implement a simple HTTP server to realize the file download function.
- Import required packages
First, we need to import some required packages. In Go language, we can use "net/http" and "os" packages to implement HTTP server and file operations.
package main import ( "fmt" "net/http" "os" )
- Implementing the HTTP server
Next, we will create a processor function to handle HTTP requests from the client. In this example, we will implement a simple download function. The client passes the file name through a GET request, and the server will return the file to the client.
func fileHandler(w http.ResponseWriter, r *http.Request) { // 获取文件名 filename := r.URL.Query().Get("filename") // 打开文件 file, err := os.Open(filename) if err != nil { // 文件不存在或无法打开,返回404 http.NotFound(w, r) return } defer file.Close() // 设置响应头 fileInfo, _ := file.Stat() w.Header().Set("Content-Disposition", "attachment; filename="+filename) w.Header().Set("Content-Type", "application/octet-stream") w.Header().Set("Content-Length", fmt.Sprintf("%d", fileInfo.Size())) // 发送文件给客户端 http.ServeContent(w, r, filename, fileInfo.ModTime(), file) }
In this processor function, we first get the file name passed by the client. Then we open the file and do error checking. If the file does not exist or cannot be opened, we will return a 404 error to the client. If the file opens successfully, we set response headers including the downloaded file name, type, and length. Finally, we use the http.ServeContent
function to send the file content to the client.
- Register processor function
In the main
function, we need to register the processor function and bind it to a path, So that clients can access the service through this path. In this example, we register the handler function on the "/download" path.
func main() { http.HandleFunc("/download", fileHandler) http.ListenAndServe(":8000", nil) }
We use the http.HandleFunc
function to register the processor function on the specified path. Then, we call the http.ListenAndServe
function to listen to the specified port in order to receive requests from the client.
- Run the server
Now that we have completed the implementation of the HTTP server, we can run the server and test the download functionality. Run go run main.go
on the command line to start the server. The server will listen on port 8000 and wait for client requests.
When the client accesses http://localhost:8000/download?filename=test.txt
, the server will try to open the file named test.txt
file and send it to the client. If the file does not exist, the server will return a 404 error.
Through the above code example, we can see that it is very simple to implement an HTTP server to download files using the network programming functions provided by the Go language. Through this example, we can understand the powerful functions of Go language in network programming and how to use these functions to achieve actual application requirements.
The above is the detailed content of How to use network programming functions in Go language to implement HTTP server downloading files?. For more information, please follow other related articles on the PHP Chinese website!
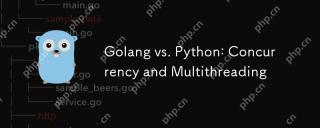
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
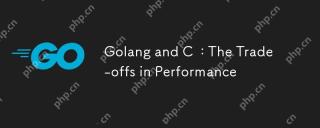
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
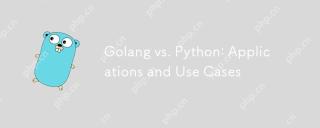
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
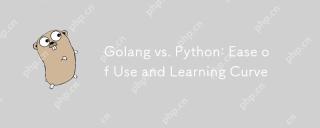
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
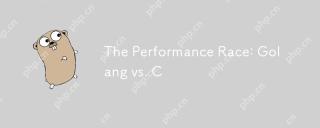
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
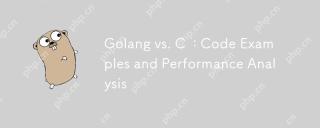
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
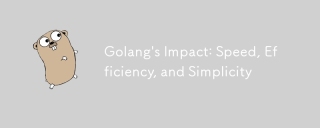
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
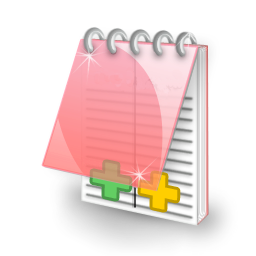
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
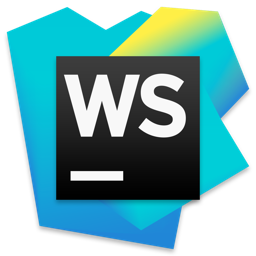
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment