


Step-by-step tutorial: How to use PHP to extend SQLite for database management
Introduction:
SQLite is an open source database engine that supports most SQL standards and provides a lightweight , Server-less database solutions. In PHP, we can use SQLite through extensions to achieve database management. This tutorial will walk you through how to use PHP to extend SQLite for database management.
Step 1: Install the SQLite extension
First, we need to make sure that the SQLite extension is installed in PHP. You can make sure the extension is enabled by uncommenting the following code in the php.ini file:
extension=sqlite3
Save the file and restart the web server for the changes to take effect.
Step 2: Create database
Using SQLite, we can connect and operate the database by using the SQLitePDO class. First, we need to create a database file. You can use the following code to create a database file named "mydatabase.db":
<?php $db = new PDO("sqlite:mydatabase.db"); ?>
This will create a database file named "mydatabase.db" in the current directory Database file. If the file already exists, the existing file is opened.
Step 3: Create table and insert data
Next, let us create a table named "users" and insert some sample data. You can use the following code to create a table and insert data:
<?php // 创建users表格 $sql = "CREATE TABLE users ( id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT, email TEXT )"; $db->exec($sql); // 插入数据 $sql = "INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com')"; $db->exec($sql); $sql = "INSERT INTO users (name, email) VALUES ('Jane Smith', 'jane@example.com')"; $db->exec($sql); $sql = "INSERT INTO users (name, email) VALUES ('Bob Johnson', 'bob@example.com')"; $db->exec($sql); ?>
Step Four: Query the Data
Now, we can execute a query to retrieve the data stored in the database. The following code will retrieve all user data from the "users" table and output it:
<?php // 查询数据 $sql = "SELECT * FROM users"; $result = $db->query($sql); // 输出数据 while ($row = $result->fetch()) { echo "ID: " . $row['id'] . "<br>" . "Name: " . $row['name'] . "<br>" . "Email: " . $row['email'] . "<br><br>"; } ?>
Step 5: Update data
If you need to update data, you can use the following code to update the data of a specific row:
<?php // 更新数据 $id = 1; $newName = "Updated Name"; $newEmail = "updated@example.com"; $sql = "UPDATE users SET name='$newName', email='$newEmail' WHERE id=$id"; $db->exec($sql); ?>
Step 6: Delete data
If you need to delete data, you can use the following code to delete the data of a specific row:
<?php // 删除数据 $id = 2; $sql = "DELETE FROM users WHERE id=$id"; $db->exec($sql); ?>
Summary:
Through this tutorial, we learned how to pass PHP extends SQLite for database management. We learned how to create a database, create tables, insert data, query data, update data and delete data. SQLite is a powerful yet simple database solution suitable for small projects and rapid development. Hope this tutorial is helpful!
The above is the detailed content of Step-by-step tutorial: How to extend SQLite with php for database management. For more information, please follow other related articles on the PHP Chinese website!
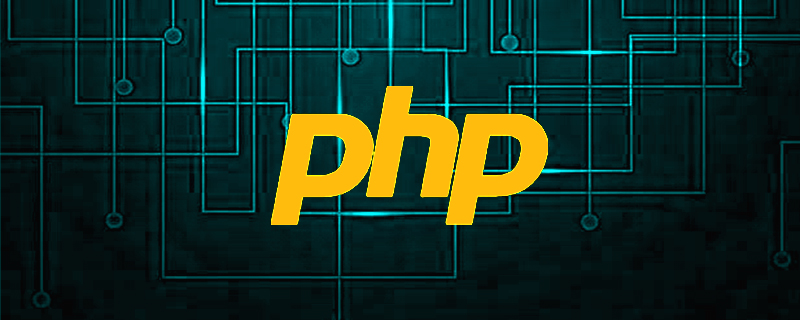
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
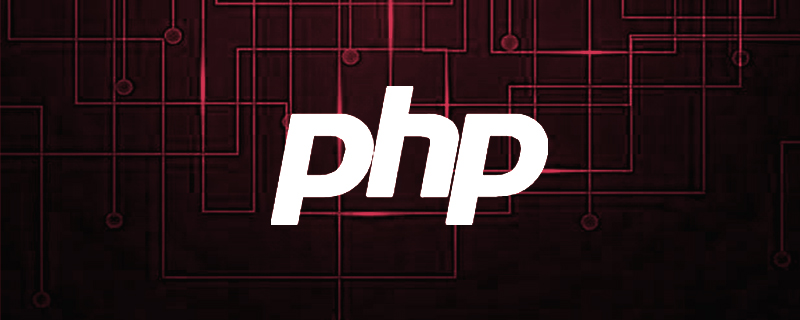
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
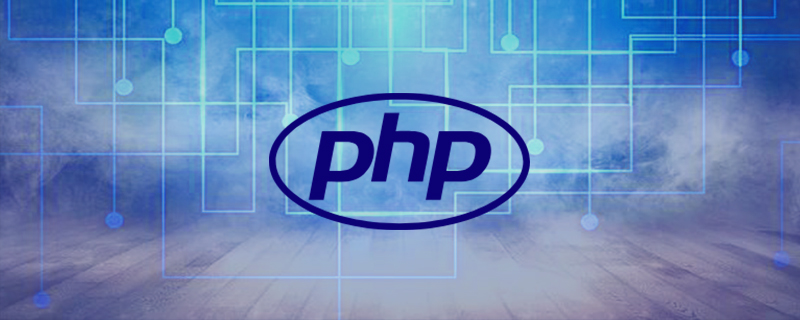
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
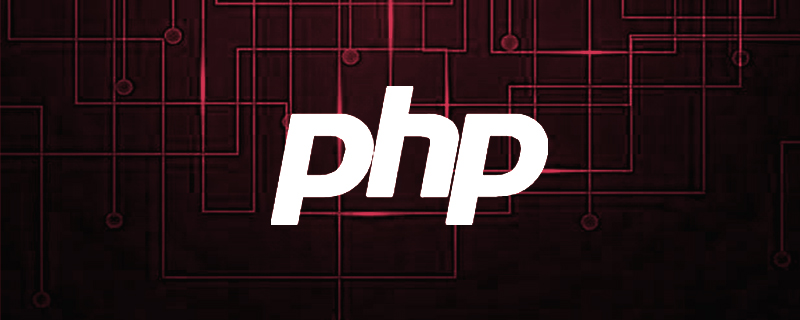
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
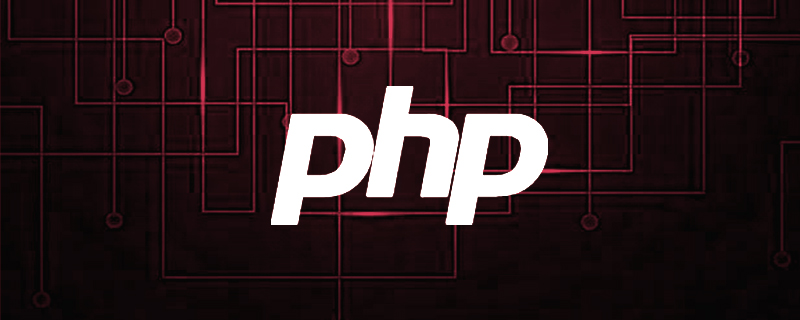
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
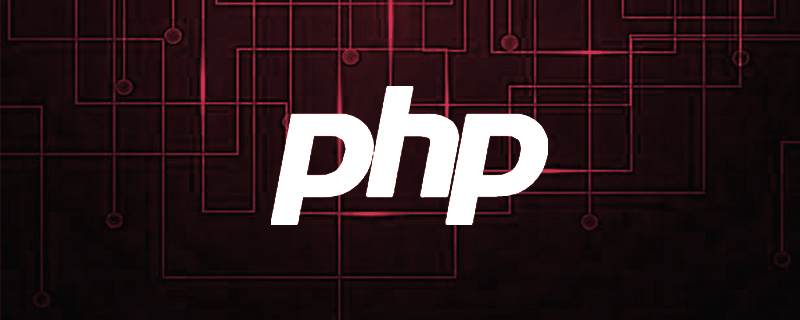
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
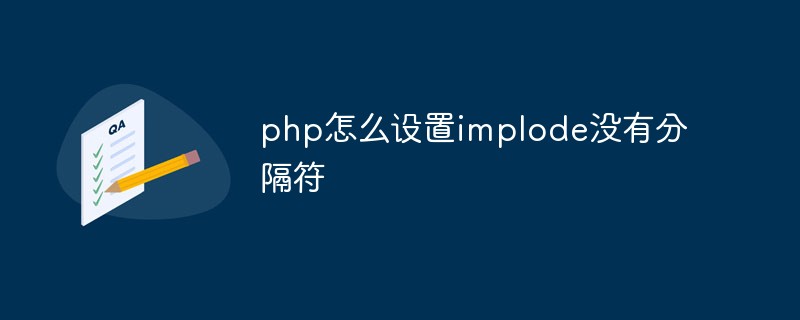
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。
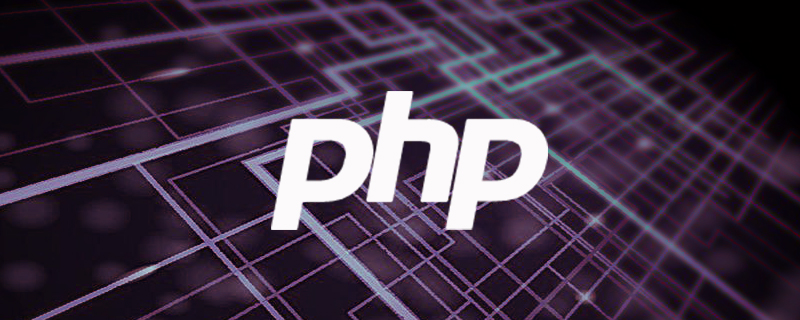
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
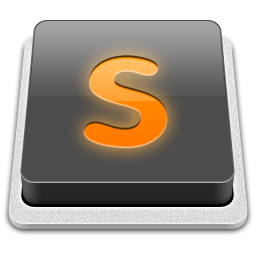
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
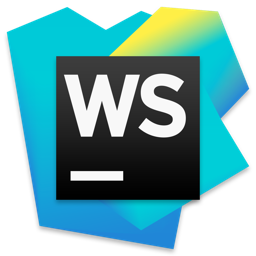
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
