


Zend Framework middleware: Add third-party login and registration support to applications
Zend Framework middleware: Add third-party login and registration support to applications
Overview:
In modern application development, user login and registration functions are essential. In order to provide better user experience and convenience, many applications provide third-party login and registration functions. As a powerful PHP development framework, Zend Framework provides middleware concepts and a series of extension components to easily add third-party login and registration support to applications.
Objective:
The purpose of this article is to introduce the steps of using Zend Framework middleware to add third-party login and registration support to the application, while providing corresponding code samples.
Steps:
Step One: Preparation
First, we need to install the relevant components of Zend Framework. It can be installed through Composer and execute the following command:
composer require zendframework/zend-expressive-authentication zendframework/zend-expressive-authentication-session
After the installation is complete, we can start writing code.
Step 2: Configure middleware
In Zend Framework, we can use the middleware mode to handle HTTP requests. First, we need to create a middleware class to handle the logic of third-party login and registration:
<?php namespace AppMiddleware; use PsrHttpMessageResponseInterface; use PsrHttpMessageServerRequestInterface; use ZendExpressiveSessionSessionMiddleware; class ThirdPartyAuthMiddleware { public function __invoke(ServerRequestInterface $request, ResponseInterface $response, callable $next = null) { // 处理第三方登录和注册逻辑 $response = $next($request, $response); return $response; } }
In the above code, we created a class named ThirdPartyAuthMiddleware and implemented the __invoke method. In this method, we can handle third-party login and registration logic and pass the processed request and response to the next middleware.
Step 3: Add routing and middleware
In Zend Framework, we use routing to map requests to the corresponding processor. We can use Zend Expressive to define routes and attach middleware to the corresponding routes.
First, we need to define the routing and corresponding middleware in the routing configuration file:
// routes.php $app->post('/login', AppHandlerLoginHandler::class, 'login')->pipe(AppMiddlewareThirdPartyAuthMiddleware::class); $app->post('/register', AppHandlerRegisterHandler::class, 'register')->pipe(AppMiddlewareThirdPartyAuthMiddleware::class);
In the above code, we created two routes /login and /register, and set the corresponding The middleware ThirdPartyAuthMiddleware is attached to both routes.
Step 4: Implement third-party login and registration logic
Finally, we need to implement specific third-party login and registration logic. We can use the extension components provided by Zend Framework to implement third-party login and registration functions, such as using the OAuth client library to handle the authentication of the OAuth protocol.
The following is a simple example showing how to use Zend Framework's OAuth1 and OAuth2 client libraries to implement third-party login for Twitter and Facebook:
<?php use ZendAuthenticationResult; use LeagueOAuth1ClientServerTwitter; use LeagueOAuth2ClientProviderFacebook; class ThirdPartyAuth { public function loginWithTwitter() { // 创建Twitter客户端 $twitter = new Twitter([ 'client_id' => 'your_client_id', 'client_secret' => 'your_client_secret', 'redirect_uri' => 'your_redirect_uri', ]); // 获取授权URL $authUrl = $twitter->getAuthorizationUrl(); // 保存OAuth令牌和令牌密钥 $this->session->set('oauth_token', $twitter->getClientCredentials()['identifier']); $this->session->set('oauth_token_secret', $twitter->getClientCredentials()['secret']); // 重定向用户到Twitter登录页面 header('Location: ' . $authUrl); exit; } public function loginWithFacebook() { // 创建Facebook客户端 $facebook = new Facebook([ 'clientId' => 'your_client_id', 'clientSecret' => 'your_client_secret', 'redirectUri' => 'your_redirect_uri', ]); // 获取授权URL $authUrl = $facebook->getAuthorizationUrl(); // 保存OAuth状态标识 $this->session->set('oauth2state', $facebook->getState()); // 重定向用户到Facebook登录页面 header('Location: ' . $authUrl); exit; } }
In the above code, we use Zend Framework's OAuth1 and OAuth2 client libraries create Twitter and Facebook clients respectively and obtain the authorization URL. By redirecting the user to the corresponding authorization URL, the user can log in and authorize our application.
Summary:
This article describes how to use Zend Framework middleware to add third-party login and registration support to applications. By writing custom middleware and using related extension components, we can easily handle third-party login and registration logic and provide better user experience and convenience.
The above is the content of this article. I hope it will be helpful for you to understand Zend Framework middleware and how to add third-party login and registration support to applications. If you have any questions or need further information, please feel free to leave a message.
The above is the detailed content of Zend Framework middleware: Add third-party login and registration support to applications. For more information, please follow other related articles on the PHP Chinese website!
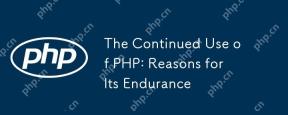
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
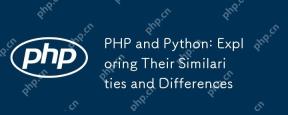
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
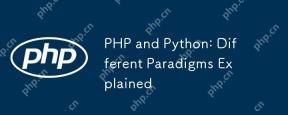
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
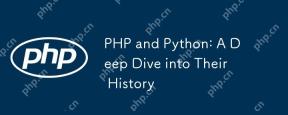
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
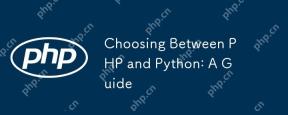
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
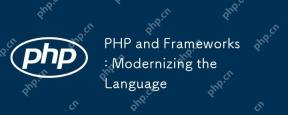
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
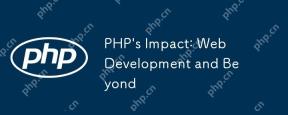
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
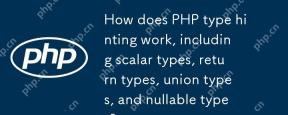
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
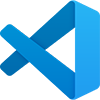
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
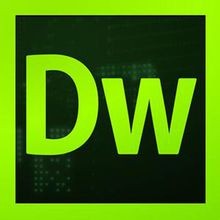
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.