Combine multiple images into one via php and Imagick
Combining multiple images into one through php and Imagick
In web development, sometimes we need to combine multiple images into one to facilitate display and save page loading time. In this article, we will introduce how to use php and the Imagick library to achieve this functionality.
Imagick is a powerful image processing library that provides a wealth of image processing methods and functions. First, we need to install the Imagick extension in php. Next, we will demonstrate how to combine multiple images into one with the following code example.
<?php // 创建一个新的Imagick对象 $combinedImage = new Imagick(); // 将多个图片添加到Imagick对象中 $images = ['image1.jpg', 'image2.jpg', 'image3.jpg']; foreach ($images as $image) { $imagePath = 'path/to/images/' . $image; // 创建一个新的Imagick对象来添加图片 $imageObject = new Imagick($imagePath); // 调整图片大小 $imageObject->resizeImage(800, 600, Imagick::FILTER_LANCZOS, 1); // 添加图片到合成图像中 $combinedImage->addImage($imageObject); } // 合并图片 $combinedImage->resetIterator(); $combinedImage->appendImages(true); // 设置输出格式 $combinedImage->setImageFormat('jpg'); // 输出合成后的图片 header('Content-Type: image/jpeg'); echo $combinedImage; ?>
In the above code example, we first create a new Imagick object $combinedImage
to store the combined image. Next, we add multiple images to the Imagick object through a loop. Before adding the images, we resized each image. Here we adjust the image to 800x600 pixels, you can adjust it according to actual needs.
After completing the addition of images, we use the appendImages(true)
method to merge all images into one. Parameter true
means merging pictures vertically. You can also use the false
parameter to perform horizontal merging. Finally, we set the output format to jpg and output the synthesized image to the browser.
This code example is just a simple example and you can extend it according to your needs. For example, you can add more pictures, adjust the order of pictures, specify the merging method, and more.
To summarize, through php and the Imagick library, we can easily combine multiple images into one. This not only improves page loading efficiency, but also facilitates unified processing of images. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of Combine multiple images into one via php and Imagick. For more information, please follow other related articles on the PHP Chinese website!
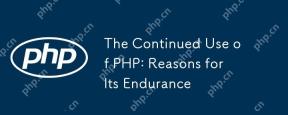
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
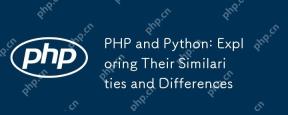
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
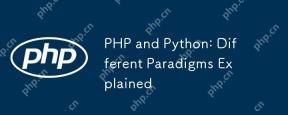
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
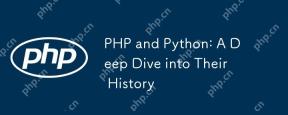
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
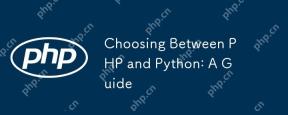
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
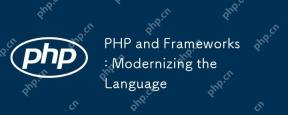
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
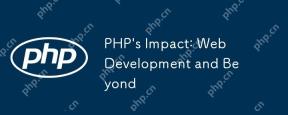
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
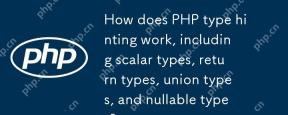
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
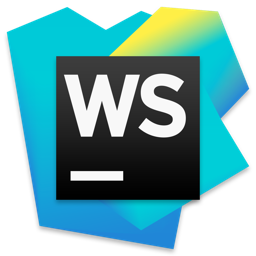
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
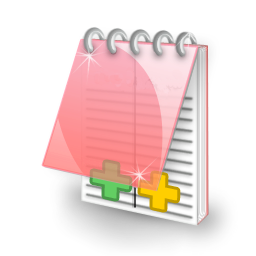
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software