


Use Slim framework middleware to realize the function of generating and scanning QR codes
Introduction:
In modern society, QR codes have become a widely used method of information transmission. Many apps and websites offer QR code generation and scanning capabilities. This article will introduce how to use the middleware of the Slim framework to realize the generation and scanning functions of QR codes.
Install the Slim framework:
First, we need to install the Slim framework. Execute the following command in the terminal:
composer require slim/slim
Generate QR code:
We will use the endroid/qrcode library to generate QR code. Execute the following command in the terminal to install the library:
composer require endroid/qrcode
Implement the QR code generation function:
Create a new PHP file, named index.php, and add the following code to the file:
require 'vendor/autoload.php'; use PsrHttpMessageServerRequestInterface as Request; use PsrHttpMessageResponseInterface as Response; use EndroidQrCodeQrCode; $app = new SlimApp; $app->get('/qrcode/generate/{text}', function (Request $request, Response $response, $args) { $text = $args['text']; $qrCode = new QrCode($text); $qrCode->setSize(300); $response->getBody()->write($qrCode->writeString()); return $response; }); $app->run();
The above code creates a Slim application and defines a GET route with the path /qrcode/generate/{text}, where {text} is the text content of the QR code to be generated. In the routing processing function, we first get the text content from the URL parameter, then use the endroid/qrcode library to create a QrCode instance, set its size to 300 pixels, and output the generated QR code as a string into the response body .
Scan QR code:
To implement the function of scanning QR code, we need to add another route and corresponding processing function. Continue to add the following code to the index.php file:
$app->post('/qrcode/scan', function (Request $request, Response $response, $args) { $qrcodeImage = $request->getBody(); // 在这里处理扫描二维码的逻辑 return $response; });
The above code creates a POST route with the path /qrcode/scan, which is used to receive scanned QR code image data. In the routing processing function, we obtain the image data in the request body through the $request->getBody() method, and then process this image data in the function, such as saving it to the server, parsing and analyze.
Notes:
In actual use, we can handle routing in more detail, such as adding logic such as request verification and permission control. In addition, in order to make the QR code scanning function more complete, third-party libraries can be combined to implement QR code decoding and analysis. This article only provides a basic example.
Summary:
Using the middleware function of the Slim framework, we can easily implement the generation and scanning functions of QR codes. The route that generates the QR code receives a text parameter, generates a QR code image based on the parameter, and returns the image to the client in the form of a string. The route that scans the QR code receives an image data, which we can further analyze and decode in the processing function.
Reference link:
- Slim framework official documentation: https://www.slimframework.com/docs/
- endroid/qrcode official documentation: https:/ /github.com/endroid/qr-code
The above is the detailed content of Use Slim framework middleware to implement QR code generation and scanning functions. For more information, please follow other related articles on the PHP Chinese website!
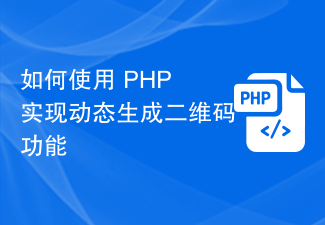
如何使用PHP实现动态生成二维码功能二维码(QRCode)被广泛应用于各个领域,它可以存储大量信息且易于扫描。在网页应用中,我们经常需要动态生成二维码,以便为用户提供便捷的操作方式。本文将介绍如何使用PHP实现动态生成二维码的功能。一、安装和配置PHPQRCode库为了方便生成二维码,我们可以使用PHPQRCode库。首先,我们需要
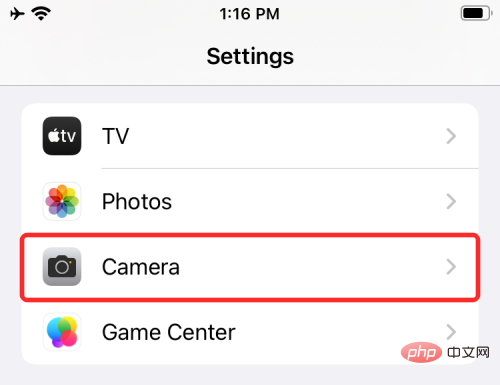
先决条件:在您的iPhone上启用二维码扫描默认情况下,所有运行iOS11的iPhone都启用了扫描QR码的功能。因此,您需要确保您的iPhone已更新到最新的可用版本,至少iOS11才能能够原生扫描QR码。在继续执行以下任何方法之前,您必须确保在iPhone上启用了该功能。您可以通过打开“设置”应用并点击“相机”部分在iPhone上启用QR码扫描。在下一个屏幕上,启用“扫描QR码”切换。这应该会打开该功能,以便您可以使用以下任何方法扫描并从QR码中提取
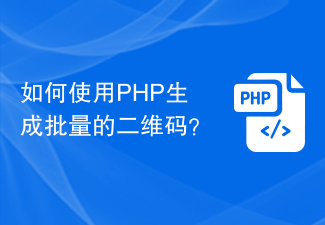
如何使用PHP生成批量的二维码?随着互联网技术的不断发展,二维码已经成为了一种非常普遍的信息传递工具。二维码可以存储大量的信息,并且可以快速扫描识别,因此在各行各业中得到了广泛的应用。在很多情况下,我们需要批量生成大量的二维码,比如用于商品标签、活动门票等。PHP是一种广泛应用于web开发的脚本语言,具有灵活、简单易用的特点。下面,我们将介绍如何使用PHP生
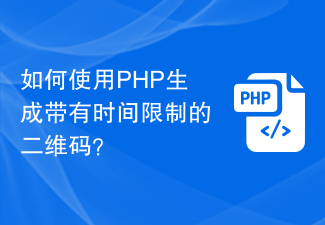
如何使用PHP生成带有时间限制的二维码?随着移动支付和电子门票的普及,二维码成为了一种常见的技术。在很多场景中,我们可能需要生成一种带有时间限制的二维码,即使在一定时间后,该二维码也将失效。本文将介绍如何使用PHP生成带有时间限制的二维码,并提供代码示例供参考。安装PHPQRCode库要使用PHP生成二维码,我们需要先安装PHPQRCode库。这个库
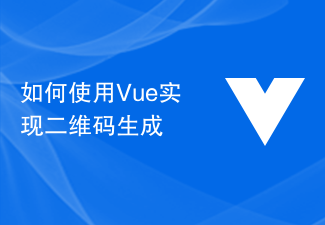
二维码是现代社会中广泛使用的一种信息编码方式,Vue是一款前端框架,如何使用Vue实现二维码生成呢?一、了解二维码生成的原理二维码的生成原理是将一段文本或一段URL地址转换成一张图片,在这张图片中编码了文本或URL地址的信息。二维码生成可以使用第三方库,本文介绍如何使用Qrcode.js库来生成二维码。Qrcode.js是一款轻量级、无依赖的二维码生成库。二
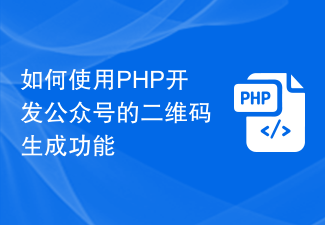
如何使用PHP开发公众号的二维码生成功能当今社交媒体的盛行使得公众号成为企业与用户互动的重要渠道之一。为了吸引更多用户关注公众号,企业常常会使用二维码来方便用户扫码关注。本文将介绍如何使用PHP开发公众号的二维码生成功能,并提供具体的代码示例。获取二维码生成地址在使用PHP开发公众号的二维码生成功能之前,我们首先需要获取二维码生成地址。可以通过微信公众平台提
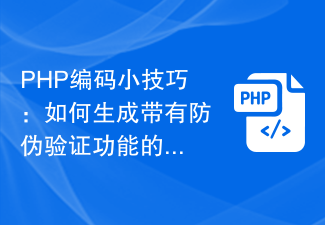
PHP编码小技巧:如何生成带有防伪验证功能的二维码?随着电子商务和互联网的发展,二维码越来越被广泛应用于各行各业。而在使用二维码的过程中,为了确保产品的安全性和防止伪造,为二维码添加防伪验证功能是十分重要的一环。本文将介绍如何使用PHP生成带有防伪验证功能的二维码,并附上相应代码示例。在开始之前,我们需要准备以下几个必要的工具和库:PHPQRCode:PHP
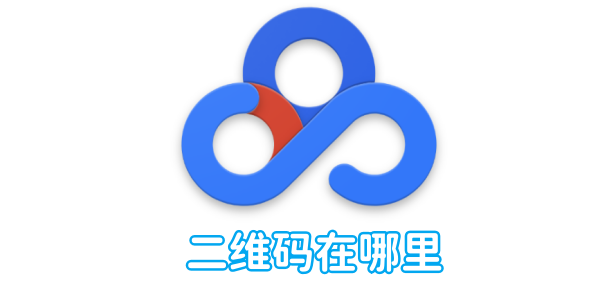
百度网盘二维码在哪里?百度网盘APP中是可以扫描二维码来使用的,但是多数的小伙伴不知道在哪来打开二维码扫一扫,接下来就是小编为用户带来的百度网盘二维码打开方法图文教程,感兴趣的用户快来一起看看吧!百度网盘二维码在哪里1、首先打开百度网盘APP,主页面右下角【我的】专区;2、然后在我的专区页面,点击左上角【头像】图标;3、之后在个人信息界面,选择【我的二维码】服务选择;4、最后即可看到专属于自己的二维码,还可以保存到相册中。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
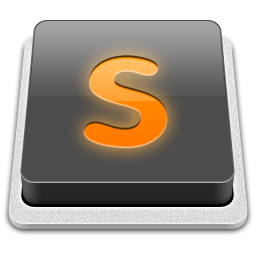
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.