


Use java's ArrayList.indexOf() function to obtain the index position of a specified element
In Java programming, ArrayList is a commonly used collection class used to store and operate a set of objects. ArrayList provides many convenient methods to handle elements in the collection, one of which is the indexOf() function. The indexOf() function is used to obtain the index position of the specified element in the ArrayList.
The syntax of the indexOf() function is as follows:
public int indexOf(Object o)
The parameter o represents the element to be searched. This function searches from the beginning of the ArrayList, finds the first matching element and returns its index position. If the element does not exist in the ArrayList, -1 is returned.
The following is a sample code using the indexOf() function:
import java.util.ArrayList; public class IndexOfExample { public static void main(String[] args) { // 创建一个ArrayList并添加一些元素 ArrayList<String> fruits = new ArrayList<>(); fruits.add("apple"); fruits.add("banana"); fruits.add("orange"); fruits.add("grape"); fruits.add("watermelon"); // 查找指定元素的索引位置 int index = fruits.indexOf("orange"); System.out.println("orange的索引位置是:" + index); index = fruits.indexOf("mango"); System.out.println("mango的索引位置是:" + index); } }
In the above code, an ArrayList object fruits is first created and some fruit elements are added. Then, use the indexOf() function to find the index positions of the two elements "orange" and "mango". Finally, print the results to the console.
Run the above code, the output is as follows:
orange的索引位置是:2 mango的索引位置是:-1
As you can see, for the element "orange" that exists in the ArrayList, the indexOf() function returns its index position 2. For the element "mango" that does not exist in the ArrayList, the indexOf() function returns -1.
In addition to strings, the indexOf() function can also search for other types of objects, such as integers, custom objects, etc. Just make sure that the object to be searched is consistent with the element type in the ArrayList.
Summary:
The index position of the specified element in the ArrayList can be easily obtained through the ArrayList.indexOf() function. In actual programming, some element operations can be performed based on the returned index position, such as deletion, replacement, etc. Please note that the indexOf() function only returns the first matching index position. If you need to get all matching index positions, you can use a loop or other methods to process it.
The above is the detailed content of Use java's ArrayList.indexOf() function to get the index position of the specified element. For more information, please follow other related articles on the PHP Chinese website!
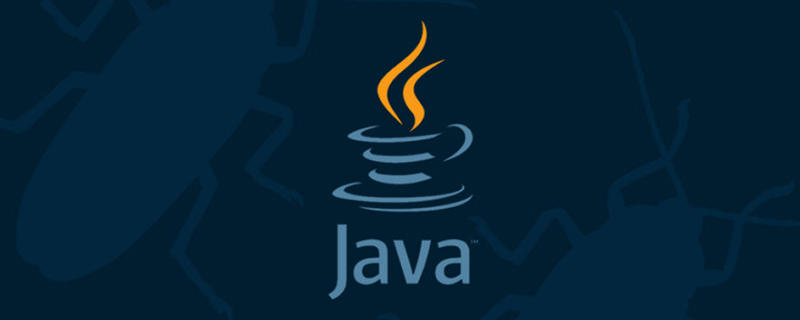
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
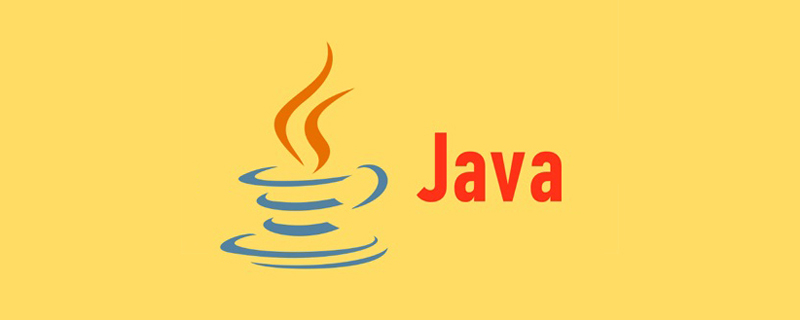
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
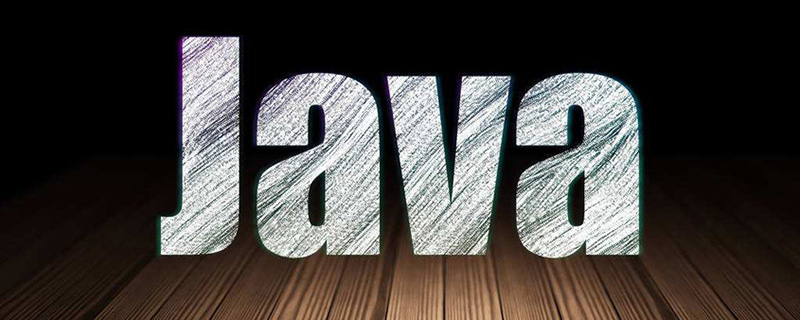
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
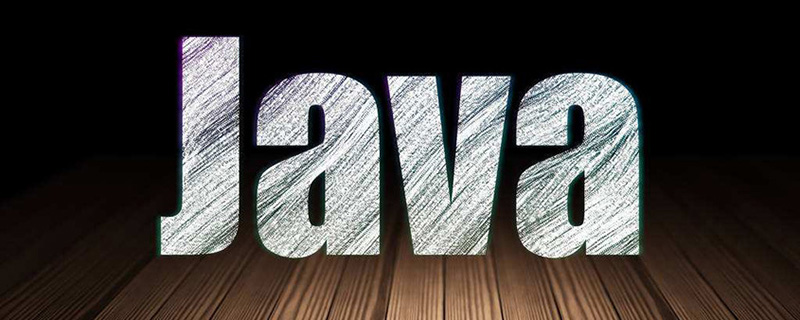
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
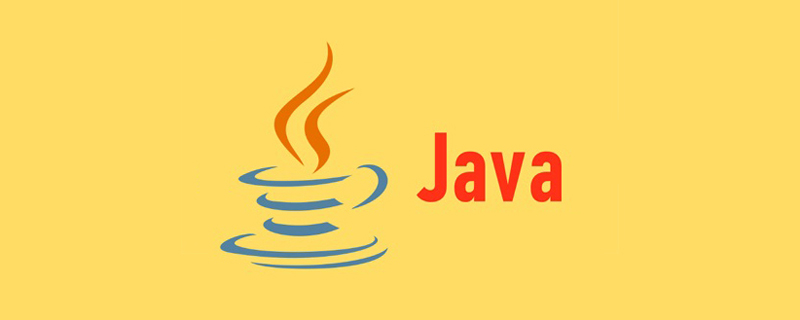
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
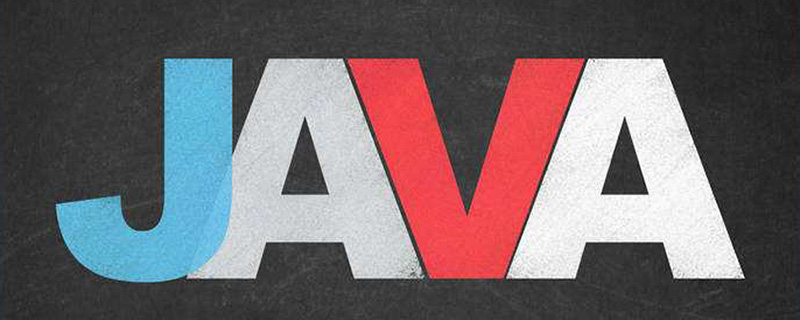
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
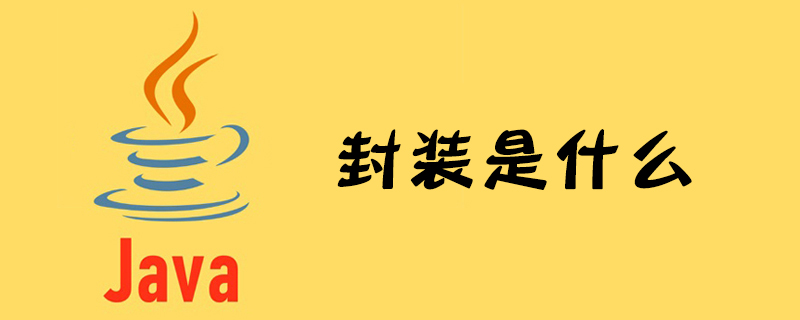
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
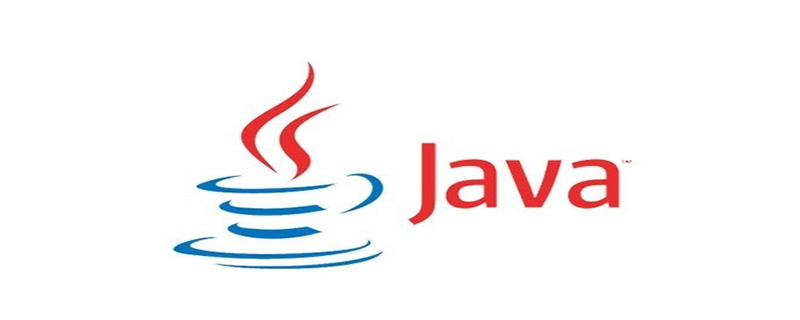
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
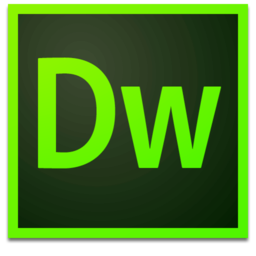
Dreamweaver Mac version
Visual web development tools
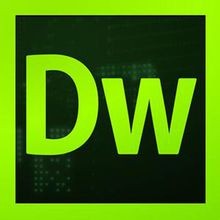
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
