


Use java's HashMap.containsKey() function to determine whether the HashMap contains the specified key
Use Java's HashMap.containsKey() function to determine whether the HashMap contains the specified key
In Java, HashMap is a commonly used data structure that stores data in the form of key-value pairs, where each Each key is unique. When we need to find whether a key exists in a HashMap, we can use the containsKey() function to determine.
containsKey() function is a member function of the HashMap class. Its function is to determine whether the HashMap contains the specified key. Its function signature is as follows:
public boolean containsKey(Object key)
This function accepts a parameter key, which represents the key to be found. If the key is contained in the HashMap, returns true; otherwise, returns false.
The following is a sample code using the containsKey() function:
import java.util.HashMap;
public class Main {
public static void main(String[] args) { // 创建一个HashMap对象并添加一些键值对 HashMap<String, Integer> hashMap = new HashMap<>(); hashMap.put("apple", 1); hashMap.put("banana", 2); hashMap.put("orange", 3); // 判断HashMap中是否含有指定的键 String key = "apple"; if (hashMap.containsKey(key)) { System.out.println(key + " is in the HashMap"); } else { System.out.println(key + " is not in the HashMap"); } key = "pear"; if (hashMap.containsKey(key)) { System.out.println(key + " is in the HashMap"); } else { System.out.println(key + " is not in the HashMap"); } }
}
Run the above code, the output result is as follows:
apple is in the HashMap
pear is not in the HashMap
In the sample code, we first create a HashMap Object hashMap and added three key-value pairs to it using the put() function. Then, we determine whether the HashMap contains the specified key by calling the containsKey() function.
In the first judgment, we set the key to "apple". Because the key exists in the HashMap, the judgment result is true and "apple is in the HashMap" is printed.
In the second judgment, we set the key to "pear". Because the key does not exist in the HashMap, the judgment result is false and "pear is not in the HashMap" is printed.
To summarize, using Java's HashMap.containsKey() function can easily determine whether the HashMap contains the specified key. This can help us quickly find the corresponding value or determine whether the key exists when we need to find a key, improving the efficiency and accuracy of the program.
The above is the detailed content of Use java's HashMap.containsKey() function to determine whether the HashMap contains the specified key. For more information, please follow other related articles on the PHP Chinese website!
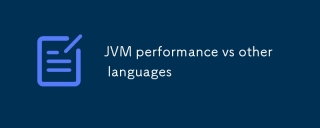
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
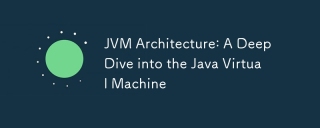
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
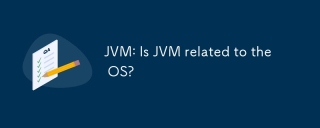
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
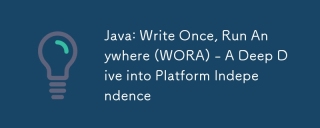
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
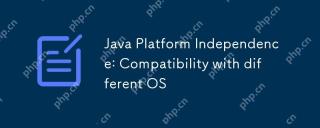
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
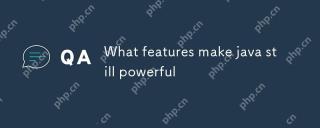
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
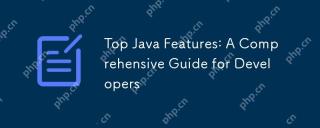
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
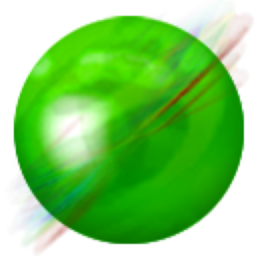
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
