


How to use the binarySearch() method of the Arrays class in Java to search for elements in an ordered array
How to use the binarySearch() method of the Arrays class in Java to search for elements in an ordered array
When facing a large amount of data, we often need to perform search operations. For sorted arrays, we can use the binary search algorithm to improve search efficiency. In Java, we can use the binarySearch() method of the Arrays class to achieve this function.
The binarySearch() method is a static method provided by the Arrays class, which can search for the position of a specified element in an ordered array. This method has two overloaded forms: one accepts a specified element and an ordered array as parameters and returns the index of the element in the array; the other accepts a specified element, an ordered array, a starting position and Taking an end position as a parameter, returns the index of the element within the specified range.
The following is a sample code that uses the binarySearch() method to search for elements:
import java.util.Arrays; public class BinarySearchExample { public static void main(String[] args) { int[] array = {1, 3, 5, 7, 9, 11, 13, 15}; int key = 9; // 使用Arrays类的binarySearch()方法在有序数组中搜索元素 int index = Arrays.binarySearch(array, key); // 输出搜索到的元素的索引 System.out.println("元素" + key + "的索引为:" + index); } }
In the above code, we define an ordered array array and an element key to be searched. Then we use the binarySearch() method of the Arrays class to search for key in the ordered array and save the result in the variable index. Finally, we output the search results to the console.
After running the above code, the console will output: "The index of element 9 is: 4". This means that element 9 has index 4 in the array.
When using the binarySearch() method, you need to pay attention to the following points:
- The array must be ordered, otherwise the result may be unpredictable.
- If there are multiple identical elements in the array, the binarySearch() method cannot guarantee which element's index is returned. You can determine whether the correct element has been found by using the index returned by the binarySearch() method and comparing it to adjacent elements.
- If the specified element does not exist in the array, the binarySearch() method will return a negative number, indicating the position where the element should be inserted. In this case, you can use "~index" to get the position where the insertion should be, where index is a negative number.
Summary
The binarySearch() method of the Arrays class is a fast and easy way to perform binary search in Java. This method allows you to efficiently search for a specified element in a sorted array. When using the binarySearch() method, note that the array must be ordered, and you also need to pay attention to the results returned by the method.
I hope this article will help you understand how to use the binarySearch() method of the Arrays class to search for elements in an ordered array. If there are any shortcomings, please correct me.
The above is the detailed content of How to use the binarySearch() method of the Arrays class in Java to search for elements in an ordered array. For more information, please follow other related articles on the PHP Chinese website!
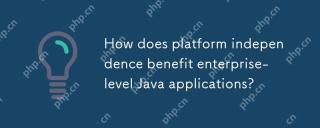
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
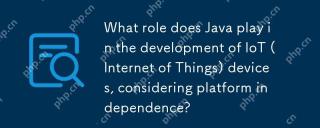
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
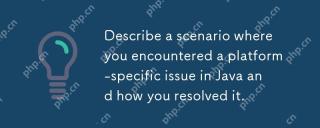
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
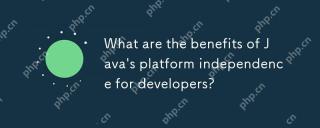
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
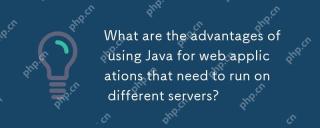
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
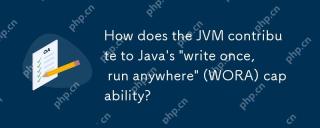
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
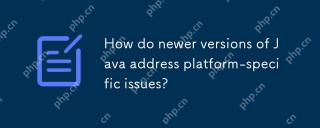
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
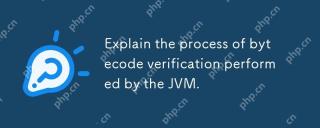
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
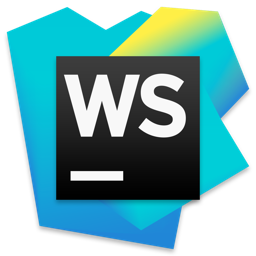
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
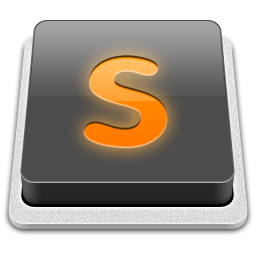
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
