


Java uses the shuffle() function of the Collections class to disrupt the order of elements in the collection.
Java uses the shuffle() function of the Collections class to disrupt the order of elements in the collection
In the Java programming language, the Collections class is a tool class that provides various static methods for operating collections. One of them is the shuffle() function, which can be used to shuffle the order of elements in a collection. This article demonstrates how to use this function and provides corresponding code examples.
First, we need to import the Collections class in the java.util package in order to use the shuffle() function. The sample code is as follows:
import java.util.ArrayList; import java.util.Collections; import java.util.List; public class ShuffleExample { public static void main(String[] args) { List<Integer> numbers = new ArrayList<>(); // 向集合中添加元素 numbers.add(1); numbers.add(2); numbers.add(3); numbers.add(4); numbers.add(5); numbers.add(6); System.out.println("打乱前的集合顺序:" + numbers); // 使用shuffle()函数打乱集合元素的顺序 Collections.shuffle(numbers); System.out.println("打乱后的集合顺序:" + numbers); } }
In the above code, we create an ArrayList collection numbers and add some integer elements to it. Then, we print out the collection order before shuffling.
Next, we use the shuffle() function of the Collections class to shuffle the order of the collection elements. This function receives a List type parameter and uses the default random source for shuffling.
Finally, we print out the shuffled collection order again. Run the program and you will see output similar to the following:
打乱前的集合顺序:[1, 2, 3, 4, 5, 6] 打乱后的集合顺序:[4, 2, 1, 5, 3, 6]
As you can see, the order of the elements in the collection has been successfully shuffled.
In addition to List collections, the shuffle() function can also be used to shuffle other types of collections, such as Set and Queue. Just pass the corresponding collection object to the shuffle() function.
It should be noted that the shuffle() function will modify the original collection object and will not create a new shuffled collection. If you need to preserve the order of the original collection, you can make a copy of the collection and then use the shuffle() function on the copied collection.
Summary:
In this article, we introduced the use of the shuffle() function of the Collections class in Java to disrupt the order of elements in the collection. By using this function, you can easily shuffle the order of elements in a collection, making them more random. This is very useful in certain application scenarios, such as random draws or shuffle games.
I hope this article will help you understand and use the shuffle() function of Java's Collections class!
The above is the detailed content of Java uses the shuffle() function of the Collections class to disrupt the order of elements in the collection.. For more information, please follow other related articles on the PHP Chinese website!
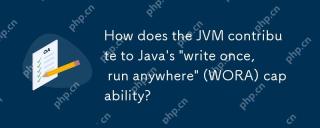
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
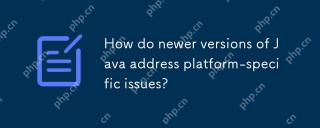
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
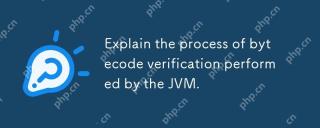
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.
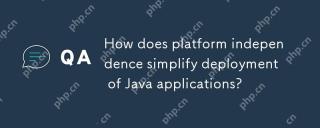
Java'splatformindependenceallowsapplicationstorunonanyoperatingsystemwithaJVM.1)Singlecodebase:writeandcompileonceforallplatforms.2)Easyupdates:updatebytecodeforsimultaneousdeployment.3)Testingefficiency:testononeplatformforuniversalbehavior.4)Scalab
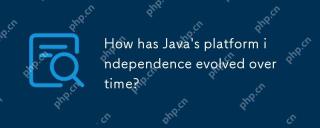
Java's platform independence is continuously enhanced through technologies such as JVM, JIT compilation, standardization, generics, lambda expressions and ProjectPanama. Since the 1990s, Java has evolved from basic JVM to high-performance modern JVM, ensuring consistency and efficiency of code across different platforms.
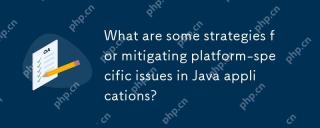
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
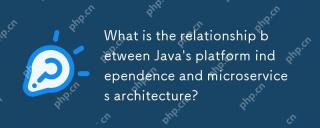
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
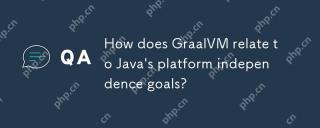
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
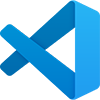
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
