


How to use Go's SectionReader module to encrypt and decrypt the content of a specified part of a file?
How to use Go's SectionReader module to encrypt and decrypt the content of a specified part of a file?
Introduction:
In development, file content encryption and decryption is a very common requirement. The Go language provides a wealth of libraries and modules to meet this need. Among them, SectionReader is a very practical module that allows us to specify the range of content in a large file and perform reading, encryption, and decryption operations. This article will introduce how to use Go's SectionReader module to encrypt and decrypt the content of a specified part of a file.
1. Overview:
The SectionReader module is an important module in the Go language. It implements the Read, Seek and ReadAt methods, allowing us to read a specified part of a large file. This article will use the SectionReader module to implement encryption and decryption of content. Encryption uses a simple XOR operation, and decryption uses the same XOR operation.
2. Code example:
The following is a code example that implements content encryption and decryption of a specified part of a file based on the SectionReader module:
package main import ( "crypto/rand" "fmt" "io" "os" ) // 加密内容 func encrypt(data []byte, key byte) { for i := range data { data[i] ^= key } } // 解密内容 func decrypt(data []byte, key byte) { encrypt(data, key) } func main() { // 打开文件 file, err := os.Open("sample.txt") if err != nil { fmt.Println("打开文件失败:", err) return } defer file.Close() // 获取文件大小 fileInfo, err := file.Stat() if err != nil { fmt.Println("获取文件信息失败:", err) return } fileSize := fileInfo.Size() // 生成随机密钥 key := make([]byte, 1) if _, err := rand.Read(key); err != nil { fmt.Println("生成随机密钥失败:", err) return } // 创建SectionReader sectionReader := io.NewSectionReader(file, 0, fileSize) // 读取文件内容 buffer := make([]byte, fileSize) if _, err := sectionReader.Read(buffer); err != nil { fmt.Println("读取文件内容失败:", err) return } // 加密文件内容 encrypt(buffer, key[0]) // 创建加密文件 encryptedFile, err := os.Create("encrypted_sample.txt") if err != nil { fmt.Println("创建加密文件失败:", err) return } defer encryptedFile.Close() // 写入加密内容 if _, err := encryptedFile.Write(buffer); err != nil { fmt.Println("写入加密内容失败:", err) return } // 重新打开加密文件 encryptedFile, err = os.Open("encrypted_sample.txt") if err != nil { fmt.Println("重新打开加密文件失败:", err) return } defer encryptedFile.Close() // 创建SectionReader encryptedSectionReader := io.NewSectionReader(encryptedFile, 0, fileSize) // 读取加密文件内容 encryptedBuffer := make([]byte, fileSize) if _, err := encryptedSectionReader.Read(encryptedBuffer); err != nil { fmt.Println("读取加密文件内容失败:", err) return } // 解密文件内容 decrypt(encryptedBuffer, key[0]) // 创建解密文件 decryptedFile, err := os.Create("decrypted_sample.txt") if err != nil { fmt.Println("创建解密文件失败:", err) return } defer decryptedFile.Close() // 写入解密内容 if _, err := decryptedFile.Write(encryptedBuffer); err != nil { fmt.Println("写入解密内容失败:", err) return } fmt.Println("加密解密完成") }
3. Code interpretation:
- First, we open a file and get the size of the file.
- Then, generate a random key.
- Next, create a SectionReader and use the Read method to read the contents of the file into the buffer.
- Use the encrypt function to encrypt the contents of the buffer.
- Create an encrypted file and write the encrypted content into it.
- Reopen the encrypted file and create a new SectionReader.
- Use the Read method to read the contents of the encrypted file into a new buffer.
- Use the decrypt function to decrypt the contents of the buffer.
- Create a decrypted file and write the decrypted content into it.
- The encryption and decryption process is completed.
4. Summary:
This article introduces how to use Go’s SectionReader module to encrypt and decrypt the content of a specified part of a file. Through the Read method of SectionReader, we can specify the content range to be read and perform encryption and decryption operations on it. Using the SectionReader module can facilitate the processing of large files and improve the efficiency and readability of the code.
It is worth noting that the encryption algorithm in this example is just a simple XOR operation, and the actual encryption algorithm should be selected and implemented according to specific needs. At the same time, security must be paid attention to when generating and saving keys to prevent the risk of key leakage and data leakage.
The above is the detailed content of How to use Go's SectionReader module to encrypt and decrypt the content of a specified part of a file?. For more information, please follow other related articles on the PHP Chinese website!
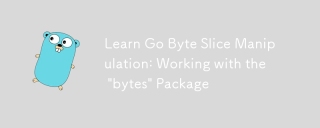
ThebytespackageinGoisessentialformanipulatingbytesliceseffectively.1)Usebytes.Jointoconcatenateslices.2)Employbytes.Bufferfordynamicdataconstruction.3)UtilizeIndexandContainsforsearching.4)ApplyReplaceandTrimformodifications.5)Usebytes.Splitforeffici
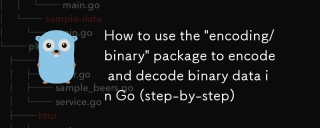
Tousethe"encoding/binary"packageinGoforencodinganddecodingbinarydata,followthesesteps:1)Importthepackageandcreateabuffer.2)Usebinary.Writetoencodedataintothebuffer,specifyingtheendianness.3)Usebinary.Readtodecodedatafromthebuffer,againspeci
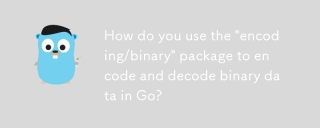
The encoding/binary package provides a unified way to process binary data. 1) Use binary.Write and binary.Read functions to encode and decode various data types such as integers and floating point numbers. 2) Custom types can be handled by implementing the binary.ByteOrder interface. 3) Pay attention to endianness selection, data alignment and error handling to ensure the correctness and efficiency of the data.

Go's strings package is not suitable for all use cases. It works for most common string operations, but third-party libraries may be required for complex NLP tasks, regular expression matching, and specific format parsing.
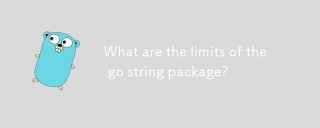
The strings package in Go has performance and memory usage limitations when handling large numbers of string operations. 1) Performance issues: For example, strings.Replace and strings.ReplaceAll are less efficient when dealing with large-scale string replacements. 2) Memory usage: Since the string is immutable, new objects will be generated every operation, resulting in an increase in memory consumption. 3) Unicode processing: It is not flexible enough when handling complex Unicode rules, and may require the help of other packages or libraries.
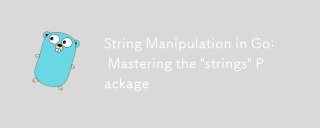
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
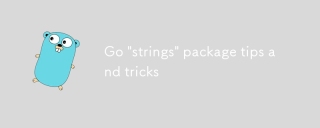
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
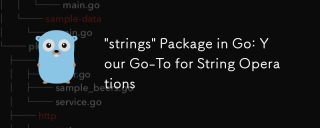
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
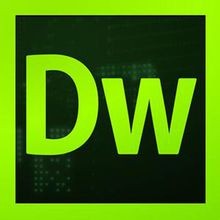
Dreamweaver CS6
Visual web development tools
