Go's strings package is not suitable for all use cases. It works for most common string operations, but third-party libraries may be required for complex NLP tasks, regular expression matching, and specific format parsing.
Go strings package: Is it suitable for all use cases?
Before we start exploring whether the strings package in Go is suitable for all use cases, we need to clarify the fact that no tool or library can meet all the needs of everyone. Go's strings package, as part of the Go standard library, provides rich string processing capabilities, but is it perfect? Let's take a deeper look.
Go's strings package is indeed very powerful. It provides many commonly used string manipulation functions, such as splicing, segmentation, searching, replacement, etc. These functions are sufficient for most scenarios in daily programming. However, real-life programming requirements are diverse, and some specific domain or complex use cases may require more advanced string processing capabilities.
For example, when dealing with natural language processing (NLP) tasks, you may need more complex text analysis functions, and the strings package may seem overwhelmed. Another example is that if you need to deal with a lot of regular expression matching, the regexp package in Go's standard library might be more suitable, but it's not part of the strings package.
I have encountered many times in my programming career where I need to deal with a certain string format and the features provided by the strings package are not enough to meet the needs. I remember one time when I needed to deal with a complex CSV file that contained a variety of escape characters and nested structures, the Split and Join functions of the strings package seemed not flexible enough in this case, and I ended up choosing a third-party library to solve this problem.
Of course, the advantage of the strings package lies in its simplicity and efficiency. It provides a fast and easy-to-use solution for most common string operations. Here is an example of using the strings package for basic string operations:
package main import ( "fmt" "strings" ) func main() { // String splicing str1 := "Hello" str2 := "World" result := strings.Join([]string{str1, str2}, " ") fmt.Println(result) // Output: Hello World // String split text := "apple,banana,cherry" fruits := strings.Split(text, ",") fmt.Println(fruits) // Output: [apple banana cherry] // String search sentence := "The quick brown fox jumps over the lazy dog" index := strings.Index(sentence, "fox") fmt.Println(index) // Output: 16 // String replacement original := "Go is awesome" replaced := strings.Replace(original, "awesome", "fantastic", 1) fmt.Println(replaced) // Output: Go is fantastic }
This example shows some basic usage of the strings package, but if you need more complex operations, such as multilingual support, complex regular expression processing, or parsing in specific formats, the strings package may appear to be stretched.
In this case, you may want to consider using a third-party library. For example, for complex text processing tasks, you can use github.com/blevesearch/bleve
for full text search and indexing; for regular expressions, you can use the regexp
package in the Go standard library; for parsing in specific formats, you can choose JSON parsing libraries like github.com/tidwall/gjson
.
Of course, using third-party libraries also has some potential risks and challenges. For example, third-party libraries may increase the complexity of project dependency management, may have version compatibility issues, or may introduce security vulnerabilities. Therefore, when choosing to use a third-party library, it is necessary to carefully evaluate its necessity and reliability.
Overall, Go's strings package is powerful enough for most common string operations, but in some specific scenarios, other tools or libraries may be required to meet the needs. As developers, we need to flexibly choose the right tools and methods according to specific project needs.
In actual projects, I suggest you try using the strings package to solve the problem first, and only consider introducing other libraries when encountering obvious limitations. This maximizes the benefits of the Go standard library while avoiding unnecessary increase in dependencies.
The above is the detailed content of Go strings package: is it complete for every use case?. For more information, please follow other related articles on the PHP Chinese website!
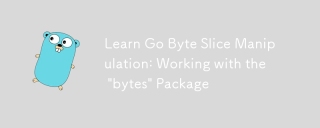
ThebytespackageinGoisessentialformanipulatingbytesliceseffectively.1)Usebytes.Jointoconcatenateslices.2)Employbytes.Bufferfordynamicdataconstruction.3)UtilizeIndexandContainsforsearching.4)ApplyReplaceandTrimformodifications.5)Usebytes.Splitforeffici
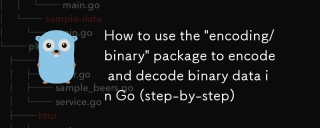
Tousethe"encoding/binary"packageinGoforencodinganddecodingbinarydata,followthesesteps:1)Importthepackageandcreateabuffer.2)Usebinary.Writetoencodedataintothebuffer,specifyingtheendianness.3)Usebinary.Readtodecodedatafromthebuffer,againspeci
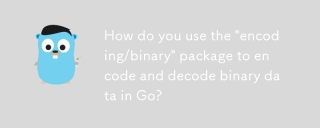
The encoding/binary package provides a unified way to process binary data. 1) Use binary.Write and binary.Read functions to encode and decode various data types such as integers and floating point numbers. 2) Custom types can be handled by implementing the binary.ByteOrder interface. 3) Pay attention to endianness selection, data alignment and error handling to ensure the correctness and efficiency of the data.

Go's strings package is not suitable for all use cases. It works for most common string operations, but third-party libraries may be required for complex NLP tasks, regular expression matching, and specific format parsing.
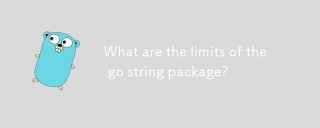
The strings package in Go has performance and memory usage limitations when handling large numbers of string operations. 1) Performance issues: For example, strings.Replace and strings.ReplaceAll are less efficient when dealing with large-scale string replacements. 2) Memory usage: Since the string is immutable, new objects will be generated every operation, resulting in an increase in memory consumption. 3) Unicode processing: It is not flexible enough when handling complex Unicode rules, and may require the help of other packages or libraries.
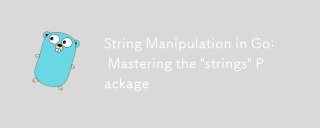
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
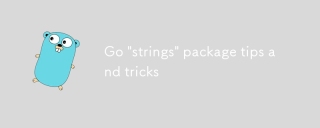
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
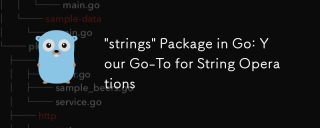
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
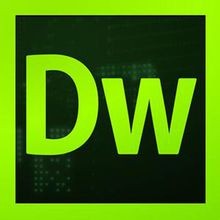
Dreamweaver CS6
Visual web development tools
